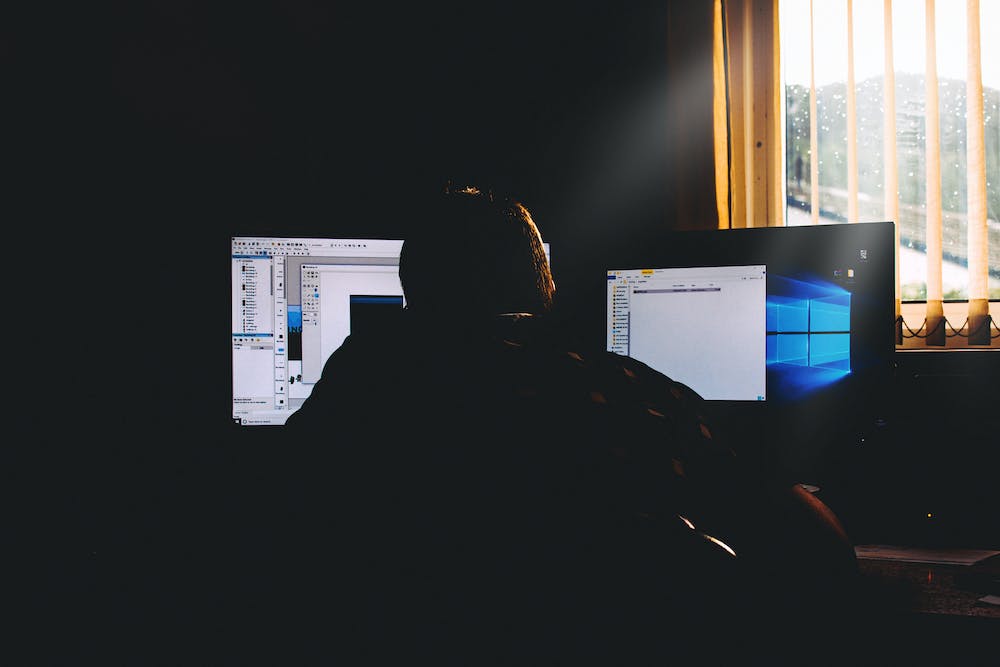
PHP, which stands for Hypertext Preprocessor, is a widely used server-side scripting language that is particularly well-suited for web development. One of the key functions in PHP is the count()
function, which is used to count the elements in an array. This article will explore the importance of the count()
function in web development and how IT can be used effectively to enhance the performance and functionality of web applications.
Importance of PHP Count in Web Development
The count()
function in PHP is an essential tool for web developers because it allows them to easily determine the number of elements in an array. This is particularly useful when working with dynamic data, such as user input or database records, where the size of the array may vary.
One of the main benefits of using the count()
function is that it provides a simple and efficient way to iterate through the elements of an array. By knowing the size of the array, developers can create loops that are tailored to the exact number of elements, which can result in more streamlined and optimized code.
Furthermore, the count()
function is often used in conjunction with conditional statements to ensure that the array has the expected number of elements before performing certain actions. This can help to prevent errors and improve the overall reliability of the code.
Another important aspect of the count()
function is its role in data validation and error handling. For example, when processing form submissions on a Website, developers can use the count()
function to check if the required fields have been filled out before proceeding with the data processing. This can help to improve the user experience by alerting users to any missing information before submitting the form.
Examples of Using PHP Count in Web Development
Let’s take a look at a few examples of how the count()
function can be used in practical web development scenarios:
Example 1: Iterating Through an Array
$fruits = array("apple", "banana", "orange");
$count = count($fruits);
for ($i = 0; $i < $count; $i++) {
echo $fruits[$i] . "
";
}
In this example, the count()
function is used to determine the size of the $fruits
array, and the result is then used to iterate through each element of the array using a for
loop. This can be a very efficient way to output the elements of an array without needing to hardcode the array size.
Example 2: Form Data Validation
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$username = $_POST["username"];
$password = $_POST["password"];
if (count($_POST) == 2) {
// Process the form data
} else {
echo "Please fill out all required fields.";
}
}
In this example, the count()
function is used to validate the form data received via the POST
method. The code checks if the number of elements in the $_POST
array matches the expected number of form fields, and then processes the data accordingly. This can help to prevent processing incomplete or erroneous form submissions.
Optimizing Performance with PHP Count
In addition to its functional importance, the count()
function can also play a role in optimizing the performance of web applications. By efficiently managing array sizes and avoiding unnecessary iterations, developers can ensure that their code runs smoothly and quickly, even when handling large amounts of data.
For example, when working with databases, the count()
function can be used to quickly determine the number of records returned by a query, allowing developers to make informed decisions about how to process the data. This can help to reduce unnecessary database calls and improve the overall efficiency of the application.
Conclusion
The count()
function in PHP is a powerful and versatile tool that is essential for web development. By providing a simple way to determine the size of arrays and effectively manage data, the count()
function plays a crucial role in creating reliable, efficient, and high-performing web applications.
FAQs
What is the syntax of the count() function in PHP?
The syntax of the count()
function is as follows:
count(array, mode)
Where array
is the input array and mode
is an optional parameter that determines the behavior of the function.
Can the count() function be used with other data types besides arrays?
Yes, the count()
function can also be used with other data types, such as objects and strings. When used with objects, the function returns the number of public properties of the object, and when used with strings, the function returns the length of the string.
Are there any performance considerations when using the count() function?
While the count()
function itself is relatively efficient, it’s important to use it judiciously, especially when working with large arrays or complex data structures. In some cases, it may be more efficient to cache the result of the count()
function if the size of the array is not expected to change frequently.