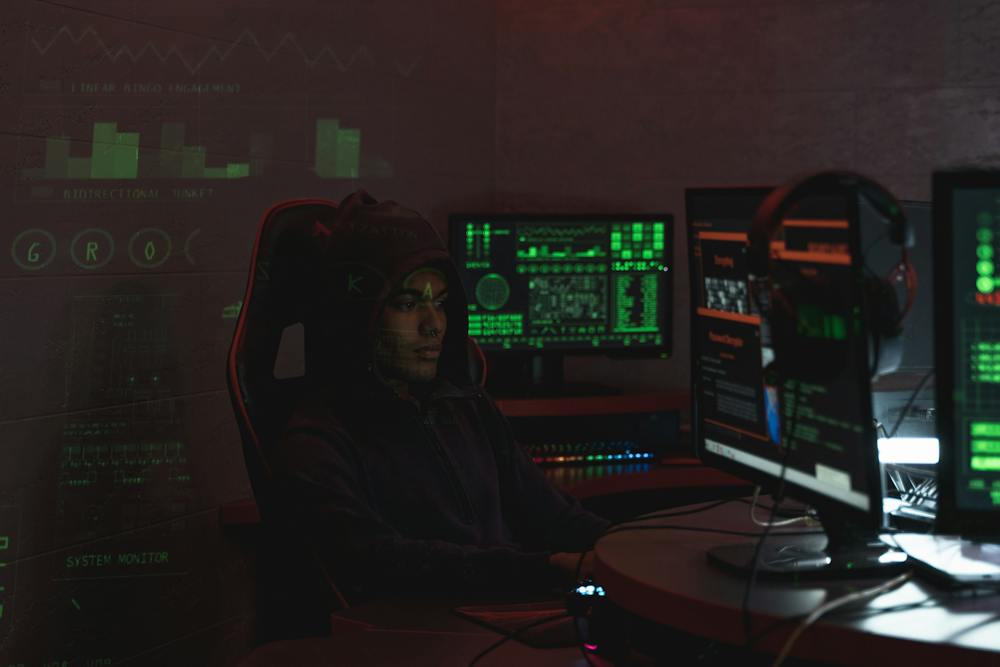
Python is a popular programming language known for its simplicity and readability. One of the factors that contribute to its readability is Pep8, a style guide for Python code. Pep8 provides a set of guidelines for writing clean, consistent, and maintainable code. In this article, we’ll explore the importance of Pep8 in Python programming and how following its guidelines can benefit developers and their projects.
What is Pep8?
Pep8 is a style guide for Python code, created by Guido van Rossum, Barry Warsaw, and Nick Coghlan. IT provides guidelines for writing clean, consistent, and readable code. The name “Pep8” is derived from the Python Enhancement Proposal (PEP) that documents the style guide, which is PEP 8.
The Pep8 style guide covers various aspects of Python programming, including indentation, line length, import statements, naming conventions, whitespace, and comments. By following these guidelines, developers can ensure that their code is easy to read, understand, and maintain.
Why is Pep8 Important?
Adhering to Pep8 guidelines is important for several reasons:
- Readability: Pep8 promotes code readability by establishing consistent formatting and style. This makes it easier for developers to understand and work with each other’s code.
- Maintainability: Consistent code style makes it easier to maintain and update code, reducing the likelihood of introducing errors.
- Collaboration: When multiple developers follow the same code style, it improves collaboration and communication within a development team.
- Professionalism: Adhering to Pep8 demonstrates professionalism and a commitment to writing high-quality code, which is important in a professional setting.
Pep8 Guidelines
Pep8 covers a wide range of coding conventions. Some of the key guidelines include:
- Indentation: Use 4 spaces per indentation level and never mix tabs and spaces.
- Line Length: Limit all lines to a maximum of 79 characters.
- Import Statements: Import statements should be on separate lines and should be grouped in the following order: standard library imports, related third-party imports, and local application/library-specific imports.
- Naming Conventions: Use descriptive names for variables, functions, and classes. Variables should be in lowercase with underscores, while functions and classes should be in mixed case with initial caps.
- Whitespace: Use whitespace to improve readability. For example, surround operators with a single space on either side.
- Comments: Use comments to explain the purpose and functionality of your code. Comments should be clear, concise, and relevant.
Enforcing Pep8
There are several tools available that help developers enforce Pep8 guidelines in their code. These tools can automatically check for Pep8 violations and provide suggestions for improvement. Some popular tools include:
- PEP8: The official Pep8 tool for checking Python code for style violations.
- flake8: A wrapper around PEP8, PyFlakes, and McCabe for checking Python code.
- Black: A tool that automatically formats Python code to comply with Pep8 guidelines.
By using these tools, developers can ensure that their code adheres to Pep8 guidelines and maintain a consistent code style throughout their projects.
Examples of Pep8 Guidelines
Let’s take a look at some examples of code that follows Pep8 guidelines:
Example 1: Indentation
“`python
def greet(name):
print(‘Hello, ‘ + name)
“`
Example 2: Line Length
“`python
long_string = ‘This is a very long string that exceeds the 79 character limit as recommended by Pep8. It should be broken into multiple lines to improve readability.’
“`
Example 3: Import Statements
“`python
import os
import sys
import requests
“`
Conclusion
Adhering to Pep8 guidelines is important for writing clean, consistent, and maintainable Python code. By following these guidelines, developers can improve the readability, maintainability, and collaboration of their code, ultimately leading to higher quality software. Additionally, using tools to enforce Pep8 guidelines can streamline the coding process and ensure that code style remains consistent throughout a project.
FAQs
What is the purpose of Pep8?
The purpose of Pep8 is to provide guidelines for writing clean, consistent, and readable Python code. By following these guidelines, developers can ensure that their code is easy to read, understand, and maintain.
How can I enforce Pep8 guidelines in my code?
There are several tools available, such as PEP8, flake8, and Black, that can help you enforce Pep8 guidelines in your code. These tools can automatically check for Pep8 violations and provide suggestions for improvement.
Does following Pep8 guidelines have any impact on performance?
Following Pep8 guidelines does not have a direct impact on the performance of your code. However, adhering to these guidelines can improve the readability and maintainability of your code, ultimately leading to higher quality software.