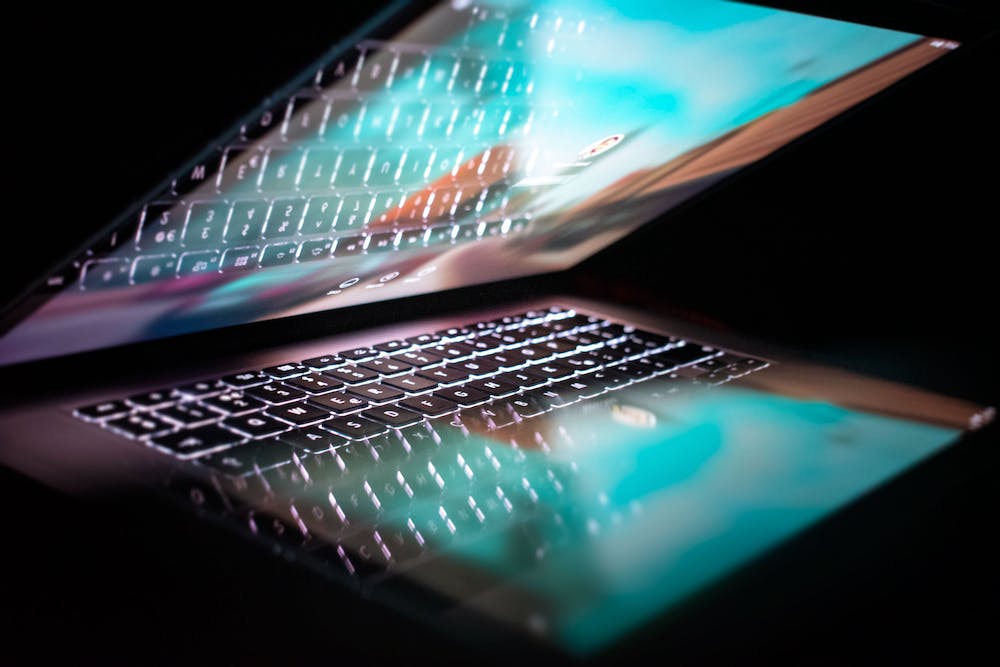
JavaScript is a powerful and widely used programming language that adds interactivity and dynamic behavior to websites. IT is primarily used for front-end web development but can also be used on the server-side. This article aims to provide a comprehensive understanding of the fundamentals of JavaScript, including its syntax, data types, control flow, and functions. Whether you are a beginner looking to dive into programming or an experienced developer seeking to refresh your knowledge, this article will serve as a valuable resource.
Syntax
The syntax of JavaScript is quite similar to other programming languages such as C++ and Java. IT is case-sensitive and uses a combination of keywords, operators, and variables to form expressions and statements. JavaScript code can be embedded directly into HTML documents using the <script>
tag. Alternatively, IT can be written in separate external files and linked to HTML pages for better code organization and reusability.
Data Types
JavaScript has several built-in data types, including:
- Number: used for numeric values.
- String: used for text values enclosed in quotation marks.
- Boolean: represents logical values either
true
orfalse
. - Array: a collection of values that can be accessed by their indices.
- Object: a collection of key-value pairs, where values can be of any data type.
- Null and Undefined: used to represent the absence of value.
- Symbol: introduced in ES6, used to create unique identifiers.
Control Flow
Control flow refers to the order in which statements and expressions are executed. JavaScript provides several control flow structures such as conditional statements (if
, else
, switch
), loops (for
, while
, do-while
), and branching statements (break
, continue
, return
). These structures allow developers to control the flow of program execution based on specific conditions and repetitive tasks.
Functions
Functions in JavaScript are reusable blocks of code that perform a specific task or return a value. They help in dividing the code into logical units and promote code reusability, readability, and maintainability. A function declaration begins with the function
keyword, followed by the function name, arguments (if any), and function body enclosed in curly braces. Functions can also be assigned to variables, known as function expressions. JavaScript supports both named and anonymous functions.
FAQs
Q1: What is the difference between let
, const
, and var
in JavaScript?
A: var
is function-scoped, and let
and const
are block-scoped. This means that variables declared with var
have their scope limited to the function they are defined in, while variables declared with let
and const
have their scope limited to the block they are defined in (e.g., within loops or conditional statements). Additionally, const
variables cannot be re-assigned once they are assigned a value, while let
variables can be re-assigned.
Q2: How can I check if a variable is an array in JavaScript?
A: JavaScript provides the Array.isArray()
method to check if a variable is an array. IT returns true
if the variable is an array, and false
otherwise. Here’s an example:
const arr = [1, 2, 3];
console.log(Array.isArray(arr)); // Output: true
Q3: What is the difference between ==
and ===
in JavaScript?
A: In JavaScript, ==
is a loose equality operator, while ===
is a strict equality operator. The loose equality operator performs type coercion, meaning IT converts the operands to a common type before comparison, while the strict equality operator requires both the value and the type to be the same for two values to be considered equal. IT is generally recommended to use ===
to avoid unexpected behavior caused by type coercion.