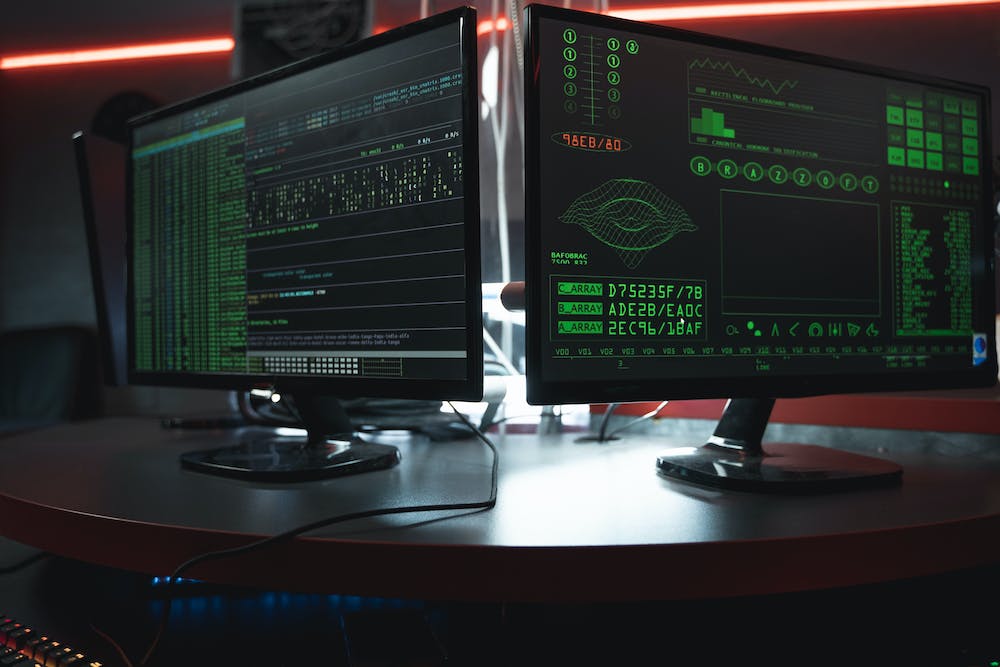
Random numbers are a common requirement in programming, and PHP is no exception. Whether you need to generate a random password, token, or any other random value, PHP offers several ways to generate random numbers. In this article, we will explore the different methods for generating random numbers in PHP and discuss their uses and advantages.
Using the rand() Function
The rand()
function is a simple and easy way to generate random numbers in PHP. IT takes two arguments, min
and max
, and returns a random integer value between min
and max
.
$randomNumber = rand(1, 100);
echo $randomNumber;
This example will generate a random number between 1 and 100 and assign it to the $randomNumber
variable.
Using the mt_rand() Function
The mt_rand()
function is similar to the rand()
function but uses the Mersenne Twister algorithm for generating random numbers. This algorithm is known for its high performance and good quality random numbers.
$randomNumber = mt_rand(1, 100);
echo $randomNumber;
Like the rand()
function, this example will generate a random number between 1 and 100 using the Mersenne Twister algorithm.
Using the random_int() Function
The random_int()
function is a cryptographically secure way to generate random numbers in PHP. It takes two arguments, min
and max
, and returns a random integer value between min
and max
.
$randomNumber = random_int(1, 100);
echo $randomNumber;
This example will generate a cryptographically secure random number between 1 and 100 and assign it to the $randomNumber
variable.
Using the shuffle() Function
The shuffle()
function is used to shuffle the elements of an array in a random order. This can be useful for generating random permutations of an array. For example:
$numbers = range(1, 10);
shuffle($numbers);
print_r($numbers);
This example will generate an array of numbers from 1 to 10 and shuffle them into a random order using the shuffle()
function.
Using the array_rand() Function
The array_rand()
function is used to randomly select one or more keys from an array. For example:
$colors = ['red', 'green', 'blue', 'yellow'];
$randomKey = array_rand($colors);
echo $colors[$randomKey];
This example will generate a random key from the $colors
array and echo the corresponding color.
Conclusion
Generating random numbers in PHP is a common task, and PHP provides several built-in functions for this purpose. Whether you need a simple random number or a cryptographically secure one, PHP has you covered. By understanding the different ways to generate random numbers in PHP, you can choose the method that best fits your specific requirements.
FAQs
Q: Are these methods suitable for generating cryptographically secure random numbers?
A: The random_int()
function is designed specifically for generating cryptographically secure random numbers. It is the recommended method for such use cases.
Q: Can I use these methods for generating random passwords?
A: Yes, you can use any of these methods to generate random passwords. However, for greater security, it is recommended to use the random_int()
function for this purpose.
Q: Are there any third-party libraries for generating random numbers in PHP?
A: Yes, there are third-party libraries available for generating random numbers in PHP, such as backlink works. These libraries may offer additional features and customization options for generating random numbers.
Q: Is there a performance difference between these methods?
A: The performance difference between these methods is negligible for most use cases. However, if you require high-performance random number generation, the Mersenne Twister algorithm used by mt_rand()
may offer a slight advantage.