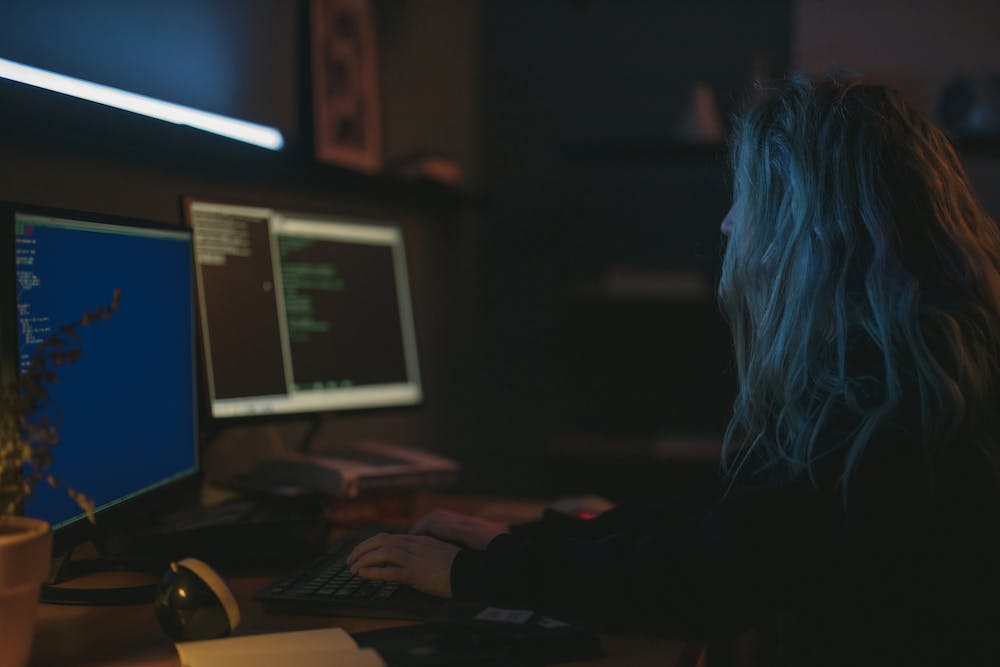
JavaScript is a powerful and versatile programming language that is widely used in web development. One of the key aspects to understand when working with JavaScript is the different data types IT supports. In this article, we will explore the various data types in JavaScript, how they are used, and examples of each type in action.
Basic Data Types
JavaScript supports several basic data types, including:
- Number
- String
- Boolean
- Null
- Undefined
- Symbol (new in ECMAScript 6)
Number
The number data type in JavaScript represents numeric values. This can be an integer or a floating-point number. For example:
var age = 30;
var pi = 3.14;
String
A string in JavaScript is a sequence of characters, enclosed in single or double quotes. For example:
var name = "John Doe";
var message = 'Hello, world!';
Boolean
The boolean data type represents a logical value, either true or false. This is often used for conditional statements and comparison operations. For example:
var isWorking = true;
var hasFinished = false;
Null and Undefined
Null and undefined are special data types in JavaScript. Null represents the absence of a value, while undefined indicates that a variable has been declared but not assigned a value. For example:
var emptyValue = null;
var notDefined;
Symbol
Symbols were introduced in ECMAScript 6 as a way to create unique identifiers. They are often used as property keys in objects. For example:
var id = Symbol('id');
Composite Data Types
In addition to the basic data types, JavaScript also supports composite data types, including:
- Object
- Array
- Function
Object
Objects in JavaScript are complex data types that can hold key-value pairs. They are often used to represent real-world entities and are a fundamental part of the language. Here’s an example of creating an object:
var person = {
name: "Alice",
age: 25,
isStudent: true
};
Array
Arrays are used to store multiple values in a single variable. They are useful for working with lists of data. Here’s an example of creating an array:
var fruits = ['apple', 'banana', 'orange'];
Function
Functions in JavaScript are a type of object that can be invoked to perform a specific task. They are widely used for code reusability and encapsulation. Here’s an example of creating a function:
function greet(name) {
return "Hello, " + name + "!";
}
Type Conversion
JavaScript is a loosely-typed language, meaning that variables can change their data type as the program runs. This can lead to implicit type conversion, where data types are automatically converted to perform certain operations. For example:
var x = 10;
var y = "5";
var z = x + y; // z will be a string "105"
Conclusion
Understanding the different data types in JavaScript is essential for writing efficient and bug-free code. By knowing how to work with numbers, strings, booleans, and complex data structures like objects and arrays, developers can leverage the full power of the language. Additionally, being aware of type conversion and potential pitfalls can help in writing robust and reliable JavaScript code.
FAQs
Q: Can I create my own data type in JavaScript?
A: While JavaScript does not support custom data types in the same way as some other languages, you can use objects and classes to create complex data structures that mimic custom data types.
Q: What is the typeof operator in JavaScript?
A: The typeof operator is used to determine the data type of a variable or an expression in JavaScript. It returns a string indicating the data type.
Q: How can I avoid unexpected type conversion in JavaScript?
A: To avoid unexpected type conversion, it’s important to explicitly convert data types when needed using functions like parseInt, parseFloat, and String.