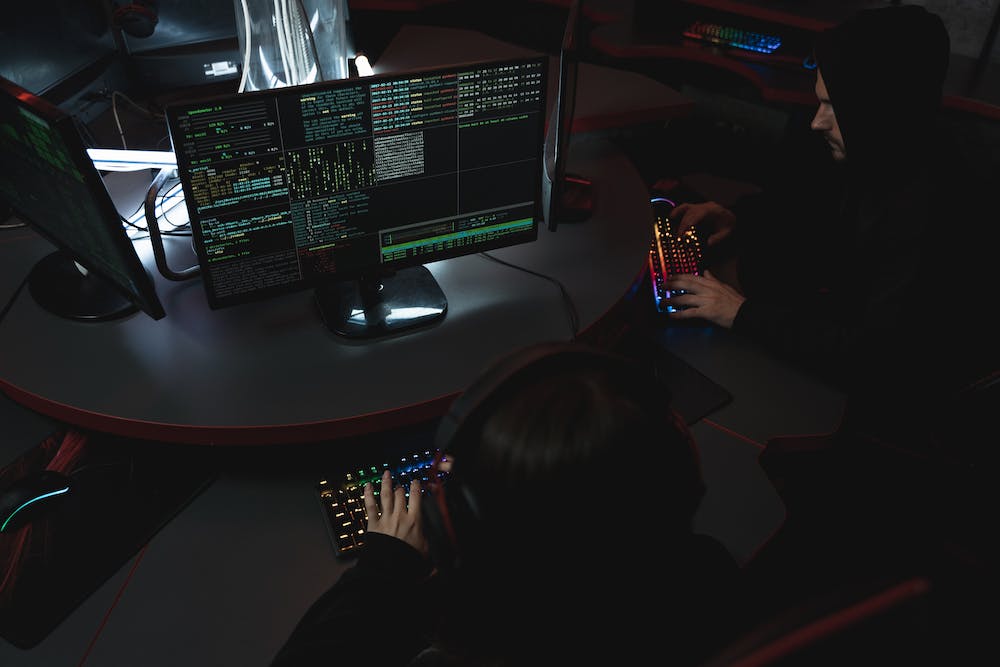
Object-Oriented Programming (OOP) is a programming paradigm that organizes code into reusable objects. PHP, as a versatile server-side scripting language, also follows this paradigm. Understanding the basics of PHP Object-Oriented Programming can greatly enhance your coding skills and allow you to build more scalable and maintainable applications. In this article, we will explore the fundamental concepts of PHP OOP and provide answers to frequently asked questions.
Classes and Objects
In PHP, a class is a blueprint for creating objects. IT defines the properties (variables) and methods (functions) that an object of that class can have. Objects, on the other hand, are instances of a class. They can have their own unique states, but they also inherit the properties and methods defined in their class.
Let’s consider an example where we want to represent a car in our application:
“`php
class Car {
// Properties
public $color;
public $brand;
// Methods
public function startEngine() {
echo “Starting the engine of the $this->color $this->brand…”;
}
public function accelerate() {
echo “Accelerating…”;
}
}
$myCar = new Car();
$myCar->color = “Red”;
$myCar->brand = “Honda”;
$myCar->startEngine(); // Output: Starting the engine of the Red Honda…
“`
In the example above, we define a `Car` class with two properties (`color` and `brand`) and two methods (`startEngine()` and `accelerate()`). We then create an instance of the `Car` class called `$myCar`. We can access the properties and methods of `$myCar` using the arrow operator `->`.
Visibility
PHP OOP supports three visibility modifiers for properties and methods: `public`, `protected`, and `private`. The visibility determines where and how the properties and methods can be accessed.
- `public` – Accessible from anywhere.
- `protected` – Accessible within the class and its subclasses.
- `private` – Accessible within the class only.
Let’s modify our `Car` class to demonstrate visibility:
“`php
class Car {
public $color; // Public property
protected $brand; // Protected property
private $engineType; // Private property
public function startEngine() {
// Accessing all properties within the class
echo “Starting the $this->engineType engine of the $this->color $this->brand…”;
}
}
$myCar = new Car();
$myCar->color = “Blue”; // Accessible as IT is a public property
$myCar->brand = “Toyota”; // Not accessible as IT is a protected property (outside the class)
$myCar->engineType = “Petrol”; // Not accessible as IT is a private property (outside the class)
$myCar->startEngine(); // Output: Starting the Petrol engine of the Blue Toyota…
“`
In the example above, we can see that the `color` property can be accessed from outside the class as IT is public. However, the `brand` and `engineType` properties are protected and private respectively, so we cannot access them outside the class.
Inheritance
Inheritance allows classes to inherit properties and methods from another class. In PHP, we can achieve inheritance using the `extends` keyword. Let’s consider an example to understand this concept:
“`php
class Vehicle {
public $color;
public function startEngine() {
echo “Starting the engine…”;
}
}
class Car extends Vehicle {
public function accelerate() {
echo “Accelerating…”;
}
}
$myCar = new Car();
$myCar->color = “Green”;
$myCar->startEngine(); // Output: Starting the engine…
$myCar->accelerate(); // Output: Accelerating…
“`
In the example above, we have a `Vehicle` class with a `color` property and a `startEngine()` method. The `Car` class extends the `Vehicle` class and adds its own method `accelerate()`. This means that the `Car` class inherits the `color` property and the `startEngine()` method from the `Vehicle` class.
FAQs
Q: What is the difference between procedural programming and object-oriented programming?
A: Procedural programming focuses on writing procedures or functions that perform operations on data, whereas object-oriented programming emphasizes organizing code into objects with properties and methods.
Q: What are the main benefits of using PHP OOP?
A: PHP OOP provides benefits like code reusability, modularity, scalability, and easier maintenance. IT allows you to build complex applications by breaking them into smaller, manageable components.
Q: Can a class inherit multiple classes in PHP?
A: No, PHP does not support multiple inheritance for classes. However, you can achieve similar functionality using interfaces and traits.
Q: What is the purpose of an abstract class?
A: An abstract class cannot be instantiated, but IT can be used as a blueprint for other classes to inherit from. IT can define abstract methods that must be implemented in the child classes.
Q: How can I autoload classes in PHP?
A: You can use the `spl_autoload_register()` function to register an autoloader function or a class method that will be called whenever a class is used but not yet loaded.
By understanding the basics of PHP Object-Oriented Programming, you can improve the structure, reusability, and maintainability of your code. This article covered key concepts such as classes, objects, visibility, inheritance, and also addressed common FAQs. Embracing OOP principles can fuel your PHP development journey and enable you to tackle complex projects with ease.