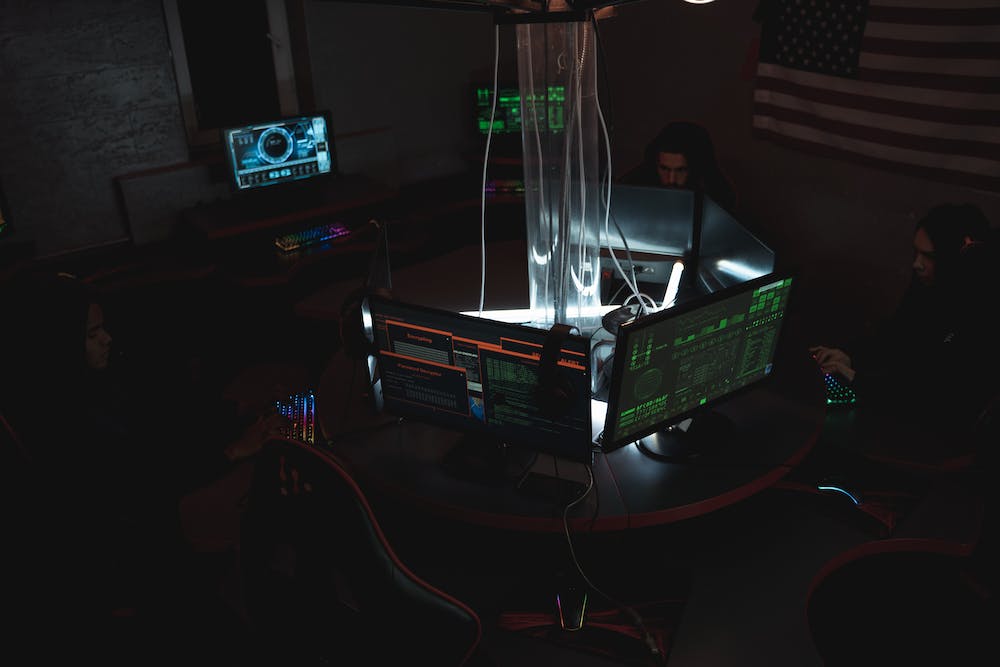
The PHP $_SERVER variable is a superglobal variable that holds information about headers, paths, and script locations. IT provides useful information about the server and the execution environment of a PHP script. Understanding this variable is crucial for developing dynamic web applications. In this article, we will explore the basics of the $_SERVER variable, its different elements, and how to use IT effectively in PHP programming.
What is the $_SERVER variable?
The $_SERVER variable is an associative array that contains various information about the current server and the execution environment. IT is a superglobal variable, which means that IT is available in all scopes throughout a PHP script. The elements of this variable are created by the web server and vary depending on the server configuration and the nature of the request.
Common Elements of the $_SERVER Variable
Here are some of the commonly used elements of the $_SERVER variable:
- $_SERVER[‘PHP_SELF’]: This element contains the filename of the currently executing script, relative to the document root.
- $_SERVER[‘REQUEST_METHOD’]: IT holds the request method used to access the page, such as GET or POST.
- $_SERVER[‘HTTP_HOST’]: This element specifies the host name provided by the client, including the port number if specified.
- $_SERVER[‘REMOTE_ADDR’]: IT contains the IP address of the client who is accessing the script.
- $_SERVER[‘HTTP_USER_AGENT’]: This element provides information about the client’s browser and operating system.
These are just a few examples, and there are many more elements available in the $_SERVER variable. You can access them by using the $_SERVER global variable followed by the element name in square brackets, like $_SERVER[‘element_name’].
Using the $_SERVER Variable
The $_SERVER variable can be used in various ways to enhance the functionality of your PHP scripts. Here are a few examples:
Example 1: Retrieving the current URL:
<?php
$currentURL = "http://$_SERVER[HTTP_HOST]$_SERVER[REQUEST_URI]";
echo "The current URL is: $currentURL";
?>
Example 2: Checking if the request is made over HTTPS:
<?php
if(isset($_SERVER['HTTPS']) && $_SERVER['HTTPS'] === 'on'){
echo "The request is made over HTTPS";
} else {
echo "The request is not made over HTTPS";
}
?>
Example 3: Displaying the client’s IP address:
<?php
$clientIP = $_SERVER['REMOTE_ADDR'];
echo "Your IP address is: $clientIP";
?>
These examples demonstrate how the $_SERVER variable can be used to retrieve important information about the server and the client, and perform specific actions based on that information.
Frequently Asked Questions (FAQs)
Q1: What is the difference between $_SERVER[‘PHP_SELF’] and $_SERVER[‘SCRIPT_NAME’]?
A1: $_SERVER[‘PHP_SELF’] contains the filename of the currently executing script, relative to the document root. On the other hand, $_SERVER[‘SCRIPT_NAME’] contains the path of the currently executing script, relative to the server’s document root.
Q2: Can we modify the $_SERVER variable?
A2: No, you cannot directly modify the elements of the $_SERVER variable. Its values are set by the server and cannot be altered within the script.
Q3: How can we access the query string parameters?
A3: The query string parameters can be accessed through the $_GET superglobal variable. For example, if the URL is “http://example.com/?id=123”, you can retrieve the value of the “id” parameter using $_GET[‘id’].
In conclusion, the PHP $_SERVER variable provides essential information about the server environment and the current request. By understanding this variable and its different elements, you can enhance the functionality and security of your PHP applications.