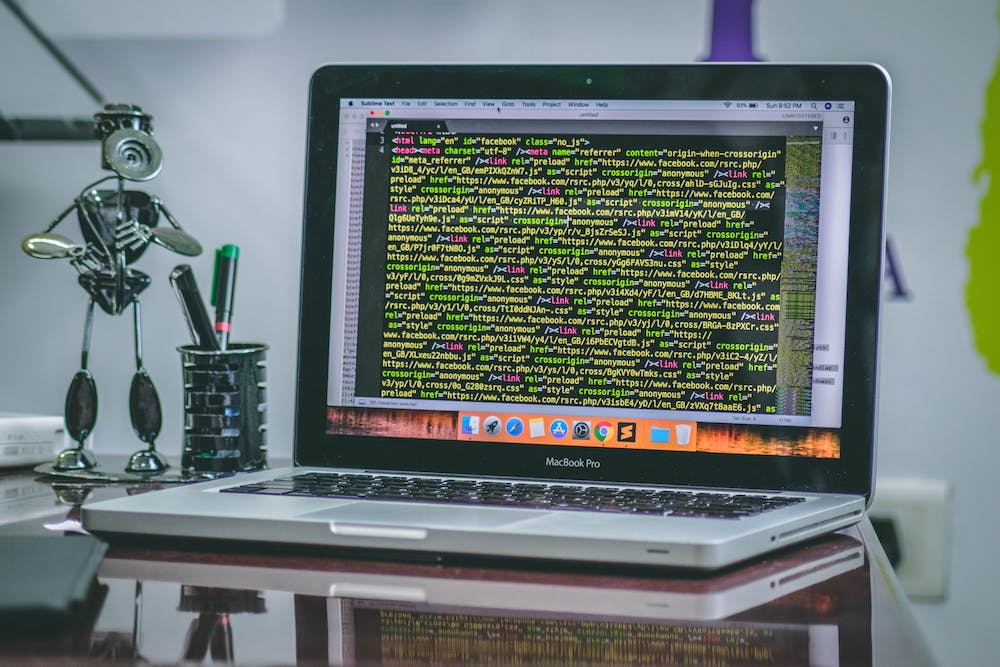
Understanding the Basics of ES6: A Comprehensive Guide
Introduction
ES6, or ECMAScript 2015, is the sixth major release of the ECMAScript language specification. IT brought significant changes and additions to JavaScript that have drastically improved the language’s readability and functionality. In this comprehensive guide, we will delve into the basics of ES6, exploring the new features and syntax IT introduced, and how they can enhance your JavaScript code.
Block Scope with Let and Const
One of the most impactful additions in ES6 was the introduction of block-scoping using the keywords ‘let’ and ‘const’. In previous versions of JavaScript, variables declared with ‘var’ had function scope, which led to confusion and unexpected behavior.
The ‘let’ keyword allows the declaration of variables with block scope. Block scope means that a variable is only accessible within the block IT was defined in. This enhances code readability and avoids scope-related bugs.
The ‘const’ keyword, on the other hand, is used to declare constants. Constants are variables whose value cannot be reassigned once they are initialized. They provide an effective way to declare values that should not change throughout the program.
Arrow Functions
ES6 introduced a concise syntax called arrow function expressions that provide a more elegant alternative to traditional function expressions. Arrow functions offer shorter syntax and lexical scoping of ‘this’.
An arrow function can be written using the following syntax:
const sum = (a, b) => {
return a + b;
};
This compact syntax eliminates the need for the ‘function’ keyword and curly braces when the function body consists of a single expression. Additionally, arrow functions inherit the ‘this’ value from the enclosing lexical scope, avoiding the need for ‘bind’, ‘call’, or ‘apply’ to control the function’s context.
Template Literals
Template literals provide an elegant way to work with strings in JavaScript. They allow for string interpolation, multiline strings, and expression evaluation within the string.
Here’s an example:
const name = 'John';
const greeting = `Hello, ${name}!
Welcome to our Website.`;
Template literals are enclosed within backticks (`) instead of single or double quotes. The placeholders, indicated by the dollar sign ($) and curly braces, allow for dynamic content and embedded expressions.
Destructuring Assignment
ES6 introduced the destructuring assignment, enabling us to extract values from arrays or objects and assign them to variables in a single statement. This not only simplifies code but also improves readability and reduces boilerplate.
An example of array destructuring:
const [x, y, z] = [1, 2, 3];
And an example of object destructuring:
const { firstName, lastName } = { firstName: 'John', lastName: 'Doe' };
In both cases, the variables ‘x’, ‘y’, and ‘z’, or ‘firstName’ and ‘lastName’, will be assigned the corresponding values, respectively.
Modules
Prior to ES6, JavaScript did not have native support for modules, which made organizing and reusing code more challenging. ES6 introduced a standardized module syntax that allows developers to divide their code into reusable modules with clear dependencies.
The new module syntax includes imports and exports:
// Exporting a function
export function greet(name) {
return `Hello, ${name}!`;
}
// Importing the exported function
import { greet } from './greetingModule.js';
This modular approach makes IT easier to manage dependencies, promotes code organization, and facilitates code sharing between projects.
Conclusion
Understanding the basics of ES6 is crucial for any JavaScript developer. The new features and syntax introduced in ES6 greatly enhance the language’s capabilities, making code more readable, maintainable, and efficient. Implementing ES6 in your codebase will bring improvements in productivity and help you stay up-to-date with modern JavaScript practices.
FAQs
Q: Can I use ES6 features in all modern browsers?
A: Most modern browsers have implemented ES6 features, but older browsers may not support them. To ensure compatibility, you can use a transpiler like Babel to convert your ES6 code into ES5, which is better supported across browsers.
Q: Are there any downsides to using ES6?
A: While ES6 brings many benefits, its extensive use of new syntax could initially increase the learning curve for developers who are not familiar with the changes. Additionally, older browsers may not fully support ES6 features, requiring extra steps for compatibility.
Q: Can I start using ES6 in my existing projects?
A: Yes, you can start using ES6 features gradually in your existing projects. You can leverage tools like Babel to transpile your ES6 code into ES5, ensuring compatibility with older browsers. Additionally, you can use module bundlers like Webpack to bundle your code and handle dependencies.
Q: Are there any performance benefits to using ES6?
A: While ES6 introduces several optimizations, the performance benefits may not always be significant. However, the improved readability, maintainability, and developer productivity that come with using ES6 can indirectly contribute to optimized and efficient code.