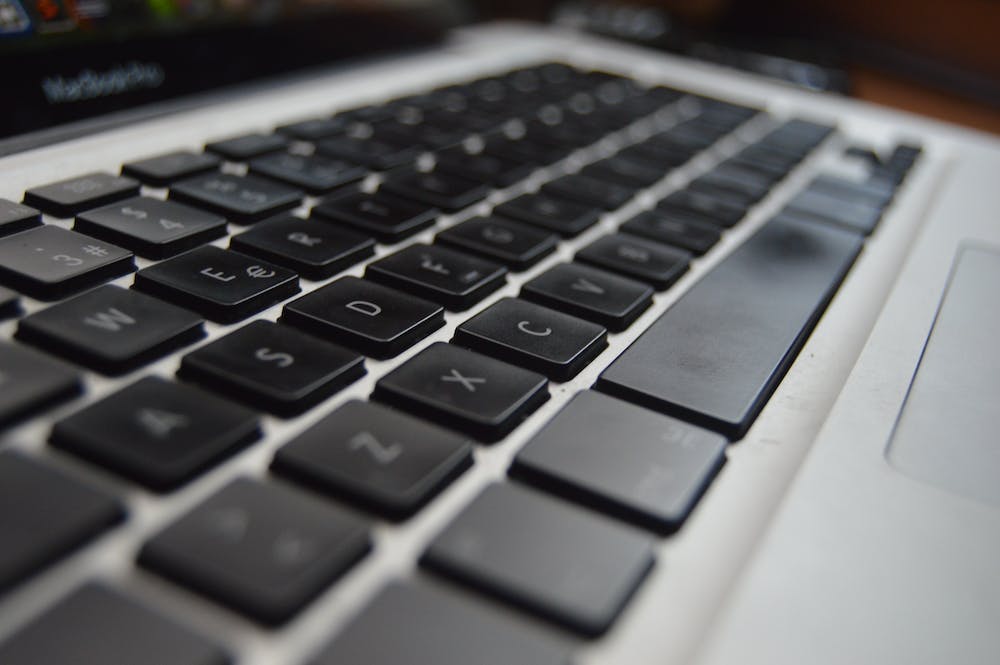
Understanding the Basics of Core JavaScript
JavaScript is a programming language widely used in web development to create interactive and dynamic web pages. IT is primarily responsible for adding behavior and interactivity to a Website. In this article, we will explore the basics of core JavaScript and learn about its essential concepts and features.
Variables:
In JavaScript, variables are used to store data or values. They can be declared using the ‘var’, ‘let’, or ‘const’ keywords.
var name = "John";
let age = 25;
const PI = 3.14;
Data Types:
JavaScript has several built-in data types, including:
- Number: represents numerical values.
- String: represents a sequence of characters.
- Boolean: represents true or false values.
- Array: represents a collection of values.
- Object: represents a collection of key-value pairs.
- Null: represents the absence of value.
- Undefined: represents an uninitialized variable.
Operators:
JavaScript provides various operators to perform different operations:
- Arithmetic Operators: +, -, *, /, %
- Assignment Operators: =, +=, -=, *=, /=
- Comparison Operators: ==, !=, >, <, >=, <=
- Logical Operators: && (and), || (or), ! (not)
Conditional Statements:
JavaScript allows you to control the flow of your program using conditional statements:
if (condition) {
// code to be executed if the condition is true
}
else {
// code to be executed if the condition is false
}
Loops:
Loops are used to execute a block of code repeatedly. JavaScript offers various types of loops:
for (initialization; condition; increment/decrement) {
// code to be executed
}
while (condition) {
// code to be executed
}
do {
// code to be executed
} while (condition);
Functions:
Functions are reusable blocks of code that perform a specific task:
function greet(name) {
return "Hello, " + name;
}
Objects:
JavaScript is an object-oriented language, and objects play a crucial role in IT. Objects are a collection of properties and methods:
var person = {
name: "John",
age: 25,
greet: function() {
return "Hello, I am " + this.name;
}
};
Event Handling:
JavaScript allows you to handle various events on web pages, such as clicks, mouse movements, and keyboard inputs.
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
Frequently Asked Questions:
Q: Is JavaScript the same as Java?
A: No, JavaScript is a scripting language used for web development, whereas Java is a general-purpose programming language.
Q: Can I use JavaScript in both front-end and back-end development?
A: Yes, JavaScript can be used in both front-end (client-side) and back-end (server-side) development. Node.js is a popular platform for back-end JavaScript development.
Q: Is there any difference between ‘let’ and ‘var’ for declaring variables?
A: Yes, ‘let’ is block-scoped, while ‘var’ is function-scoped. Variables declared with ‘let’ are limited to their block, while variables declared with ‘var’ have a broader scope.
Q: Can I modify a variable declared with ‘const’?
A: No, variables declared with ‘const’ are constants and cannot be reassigned a new value.
Q: What are the benefits of using JavaScript for web development?
A: JavaScript provides enhanced interactivity, allows dynamic content updates, facilitates form validation, and enables asynchronous operations through AJAX.
Q: Are there any frameworks or libraries available for JavaScript development?
A: Yes, there are several popular frameworks and libraries like React, Angular, and Vue.js that streamline JavaScript development and offer additional features and functionality.
Q: Is IT necessary to include JavaScript externally in HTML documents?
A: No, JavaScript can be included both internally within HTML documents using <script> tags or externally using separate JavaScript files referenced in the <script> tag.
Q: Can JavaScript be used for data validation on the client-side?
A: Yes, JavaScript can be used to validate user input on the client-side, providing immediate feedback and reducing the need for server-side validation.
Q: Are there any alternative programming languages for client-side web development?
A: Yes, other popular languages for client-side web development include TypeScript, Dart, and Kotlin.
JavaScript is a versatile and powerful programming language that forms the backbone of modern web development. Understanding the basics of core JavaScript is essential for anyone looking to build interactive and dynamic web pages. By grasping the fundamental concepts mentioned in this article, you will be well on your way to becoming proficient in JavaScript programming.