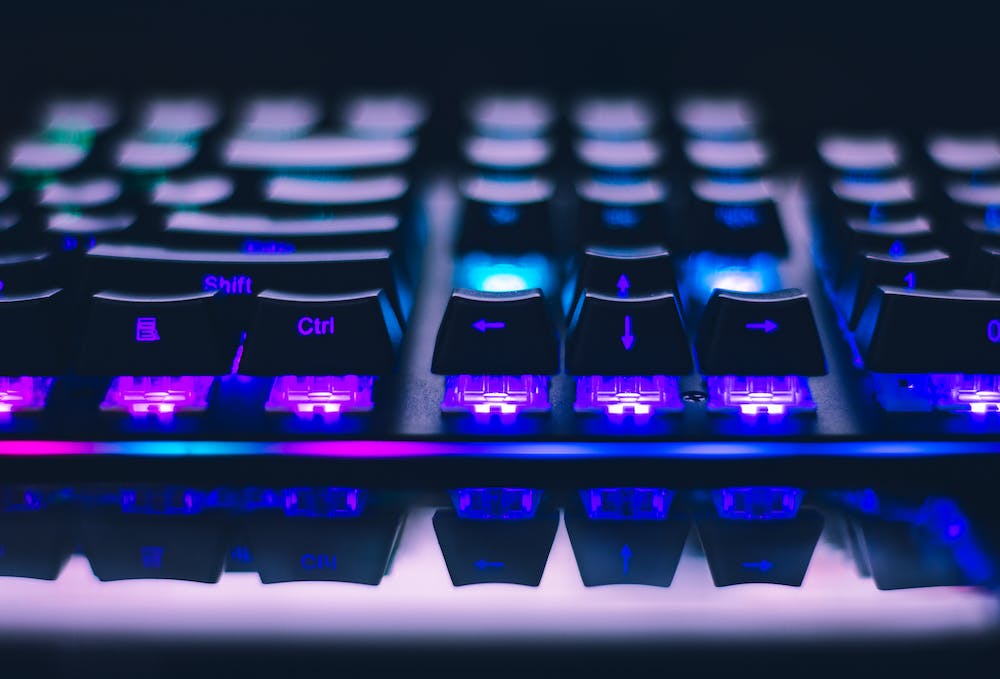
In software engineering, the Singleton design pattern is a creational pattern that ensures a class has only one instance and provides a global point of access to that instance. This pattern is widely used in various programming languages, including Node.js, to control object creation, limiting the number of instances to only one.
Implementing Singleton Design Pattern
Implementing the Singleton pattern in Node.js is quite straightforward. The key idea is to use a single instance of a class and provide a way to access that instance globally.
Here’s a simple example of implementing the Singleton pattern in Node.js:
“`javascript
class Singleton {
constructor() {
if (!Singleton.instance) {
Singleton.instance = this;
}
return Singleton.instance;
}
}
const instance1 = new Singleton();
const instance2 = new Singleton();
console.log(instance1 === instance2); // Output: true
“`
As you can see in the example above, regardless of how many times we instantiate the Singleton class, we always get the same instance. This is the essence of the Singleton pattern – ensuring that there is only one instance of a class.
Use Cases of Singleton Pattern in Node.js
The Singleton pattern can be used in various scenarios in Node.js. Some of the common use cases include:
- Database Connections: When working with databases in Node.js, IT‘s often desirable to have a single instance of a database connection that can be shared across different parts of the application.
- Logging: Managing a global logger instance that can be accessed from any part of the application.
- Configuration Settings: Storing and accessing application-wide configuration settings using a Singleton instance.
Pros and Cons of Singleton Pattern
Like any design pattern, the Singleton pattern has its own set of advantages and disadvantages. Let’s take a look at some of them:
Pros
- Global Access: Allows for global access to a single instance, making it easy to manage and share resources.
- Memory Efficiency: Avoids creating multiple instances of the same class, thus saving memory.
- Lazy Initialization: Allows for lazy initialization, meaning the instance is created only when it’s first accessed.
Cons
- Tight Coupling: The Singleton pattern can lead to tight coupling, making it harder to replace the instance with a mock for testing.
- Global State: Can lead to a global state, which might introduce unintended side effects and make the code harder to reason about.
- Concurrency Issues: If not implemented carefully, the Singleton pattern can lead to concurrency issues in a multi-threaded environment.
Best Practices for Using Singleton Pattern in Node.js
When using the Singleton pattern in Node.js, there are a few best practices to keep in mind:
- Ensure Thread Safety: If your Node.js application runs in a multi-threaded environment, make sure that your Singleton implementation is thread-safe to avoid concurrency issues.
- Consider Dependency Injection: Instead of directly referencing the Singleton instance in other parts of the code, consider using dependency injection to make the code more testable and modular.
- Avoid Overusing Singletons: While the Singleton pattern can be useful, it’s important not to overuse it. Use it only when there is a genuine need for a single instance of a class.
Conclusion
The Singleton pattern is a powerful design pattern that can be used to ensure there is only one instance of a class in Node.js. By providing a global point of access to that instance, the Singleton pattern can help manage resources effectively and efficiently.
However, it’s important to use the Singleton pattern judiciously and consider its pros and cons before incorporating it into your code. With careful implementation and consideration of best practices, the Singleton pattern can be a valuable tool in Node.js development.
FAQs
Q: Can I use the Singleton pattern with external dependencies in Node.js?
A: Yes, the Singleton pattern can be used with external dependencies in Node.js. However, it’s important to ensure that the dependencies are managed properly and the Singleton instance is created and accessed in a safe and efficient manner.
Q: Is the Singleton pattern the same as a global variable in Node.js?
A: While both the Singleton pattern and global variables provide a way to access a single instance globally, the Singleton pattern offers more control and encapsulation, making it a preferred choice for managing global instances in Node.js.
Q: Can I use the Singleton pattern with asynchronous operations in Node.js?
A: Yes, the Singleton pattern can be used with asynchronous operations in Node.js. However, it’s important to handle asynchronous operations carefully to ensure that the Singleton instance is created and accessed in a reliable and predictable manner.