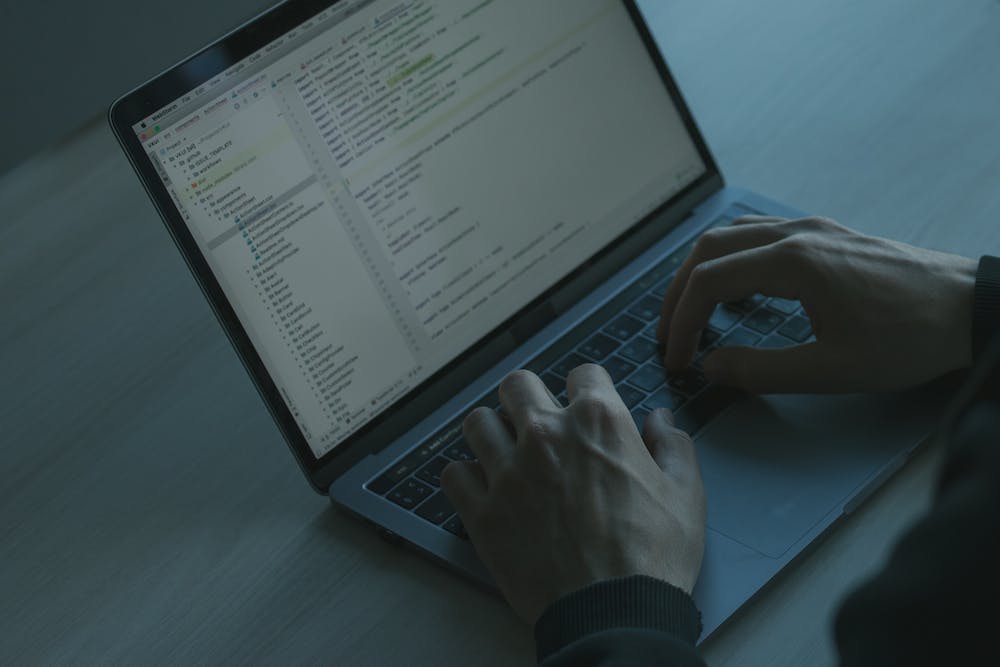
Understanding PHP Syntax: A Beginner’s Guide
PHP, which stands for Hypertext Preprocessor, is one of the most widely used programming languages for web development. IT is known for its simplicity, flexibility, and the ability to seamlessly integrate with HTML. As a beginner in PHP, IT is crucial to have a clear understanding of its syntax – how to structure and write code effectively. This guide will take you through the basics of PHP syntax, providing you with the foundation to start building dynamic websites or web applications.
The Basics: Variables, Functions, and Statements
In PHP, a variable is a container that holds a value. IT starts with a dollar sign ($) followed by the variable name. Variables are case-sensitive, so $myVar and $myvar are considered different.
Here is an example of declaring and using variables:
<?php
$firstName = "John";
$lastName = "Doe";
$age = 25;
echo "My name is " . $firstName . " " . $lastName . " and I am " . $age . " years old.";
?>
Functions are blocks of reusable code that perform specific tasks. PHP has built-in functions that can be used immediately, such as strlen()
to get the length of a string or array_push()
to add an element to an array. You can also create custom functions to suit your needs.
Here is an example of using a built-in function:
<?php
$message = "Hello, World!";
$length = strlen($message);
echo "The length of the message is " . $length . " characters.";
?>
PHP statements are instructions to be executed. Each statement must end with a semicolon (;). Here is an example:
<?php
$x = 5;
$y = 10;
$sum = $x + $y;
if ($sum > 15) {
echo "The sum is greater than 15.";
} else {
echo "The sum is less than or equal to 15.";
}
?>
Control Structures: Conditional Statements and Loops
Conditional statements and loops allow you to control the flow of your PHP code based on certain conditions or repeat code blocks multiple times.
The if
statement executes a block of code if a specified condition is true. Here is an example:
<?php
$age = 18;
if ($age >= 18) {
echo "You are eligible to vote.";
} else {
echo "You are not eligible to vote yet.";
}
?>
Loops are used to iterate through a block of code multiple times. PHP provides different types of loops, such as for
, while
, and foreach
. Here is an example of a for
loop:
<?php
for ($i = 0; $i <= 5; $i++) {
echo "Value of i: " . $i . "<br>";
}
?>
Common Mistakes and FAQs
1. Syntax Errors: Pay attention to your syntax and make sure to include semicolons at the end of each statement. Missing semicolons can lead to syntax errors.
2. Case Sensitivity: Remember that PHP is case-sensitive. Variable names must be consistent in their casing throughout your code.
3. Including Files: If you encounter issues with including files, double-check the file paths and ensure that the files you’re trying to include exist in the specified locations.
4. Undefined Variables: If you receive an “undefined variable” error, make sure the variable is declared and assigned a value before using IT.
5. Debugging: Utilize tools like error reporting and logging to help identify and fix any issues in your code. These tools can provide valuable insights into the source of errors.
Hopefully, this beginner’s guide has given you a solid understanding of PHP syntax and equipped you with the knowledge to start writing PHP code. Remember to practice and experiment with different aspects of PHP to further enhance your skills. Happy coding!