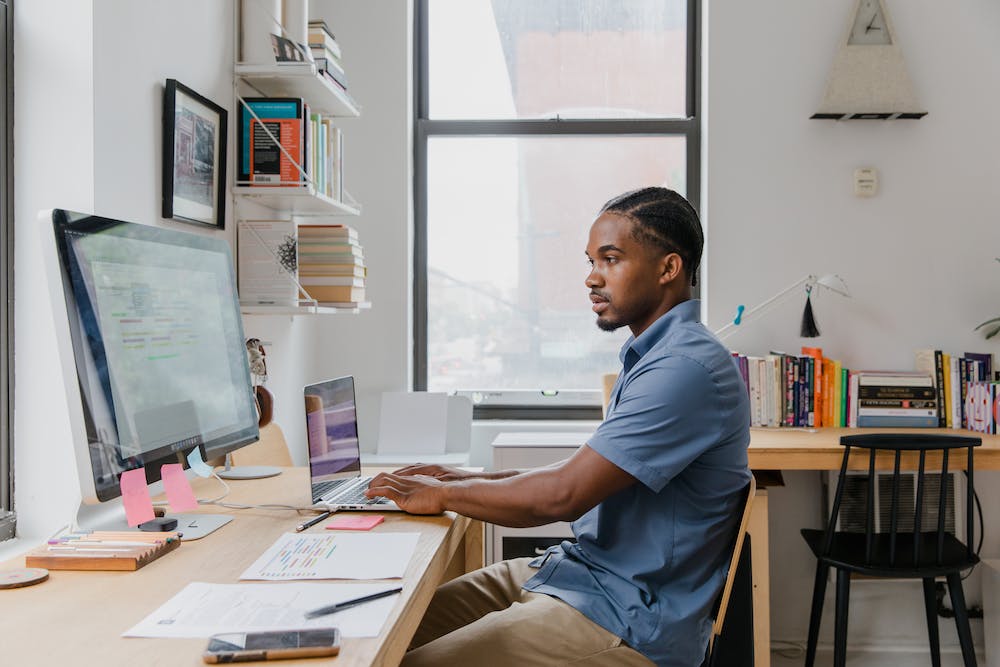
Understanding PHP: Strings and Their Importance in Web Development
In web development, strings play a vital role in various aspects of programming. Whether IT‘s displaying dynamic data, processing user input, or manipulating text, PHP offers powerful string functions and features that make IT easier to work with strings. In this article, we will delve into the world of PHP strings, their importance, and how they can be effectively utilized in web development.
Before we explore the functionalities of PHP strings, let’s first understand what strings are and why they are essential in the context of web development. In simple terms, a string is a sequence of characters enclosed within single quotes (”) or double quotes (“”). These characters can include letters, numbers, symbols, and even empty spaces. Strings are used to represent textual data, such as names, addresses, messages, or any information that can be represented as characters.
Creating and Manipulating Strings
PHP provides several methods to create and manipulate strings. Let’s take a look at some of the most commonly used techniques:
1. String Concatenation:
String concatenation is the process of combining multiple strings into a single string. In PHP, concatenation is done using the dot (.) operator. For example:
$name = “John”;
$age = 25;
$message = “Hello, my name is ” . $name . ” and I am ” . $age . ” years old.”;
echo $message;
The above code will output: “Hello, my name is John and I am 25 years old.” By using the dot operator, we can concatenate variables, constants, or even plain text within a single string.
2. String Length:
Often, we need to determine the length of a string for various purposes. The strlen() function in PHP allows us to retrieve the length of a string. For instance:
$message = “Hello, world!”;
$length = strlen($message);
echo $length;
The above code will output: 13. The strlen() function counts the number of characters in a string, including white spaces and special characters.
3. Substring Extraction:
PHP provides the substr() function to extract a portion of a string. IT takes two parameters: the string itself and the starting position from which the extraction should begin. For example:
$message = “Hello, world!”;
$substring = substr($message, 0, 5);
echo $substring;
The above code will output: “Hello”. The substr() function allows us to extract a section of a string based on the provided starting position and the optional length.
FAQs
Q1. Can I concatenate a string with a numeric value?
Yes, PHP automatically converts the numeric value to a string when concatenating. For example:
$number = 42;
$message = “The answer is: ” . $number;
The variable $message will now hold the string: “The answer is: 42”.
Q2. What is the difference between single quotes and double quotes for strings?
In PHP, single-quoted strings are treated as literal strings where variables are not expanded, and escape sequences are not interpreted. On the other hand, double-quoted strings allow variable interpolation and interpret escape sequences. For example:
$name = “John”;
$singleQuote = ‘Hello, $name!’;
$doubleQuote = “Hello, $name!”;
The $singleQuote will store the string: “Hello, $name!”, while $doubleQuote will store the string: “Hello, John!”.
Q3. How can I replace a specific part of a string?
PHP provides the str_replace() function to replace specific portions of a string with a new value. IT takes three parameters: the portion to be replaced, the replacement value, and the main string. For example:
$message = “The quick brown fox jumps over the lazy dog.”;
$newMessage = str_replace(“brown”, “red”, $message);
The $newMessage will now hold the string: “The quick red fox jumps over the lazy dog.”. Multiple replacements can be done by passing arrays to the str_replace() function.
Summary:
PHP strings are essential in web development as they allow us to work with textual data effectively. Whether IT‘s combining strings, determining their length, or extracting substrings, PHP offers various functions and methods to manipulate strings effortlessly. Understanding how to use these string features is crucial for any developer aiming to build dynamic websites and web applications.