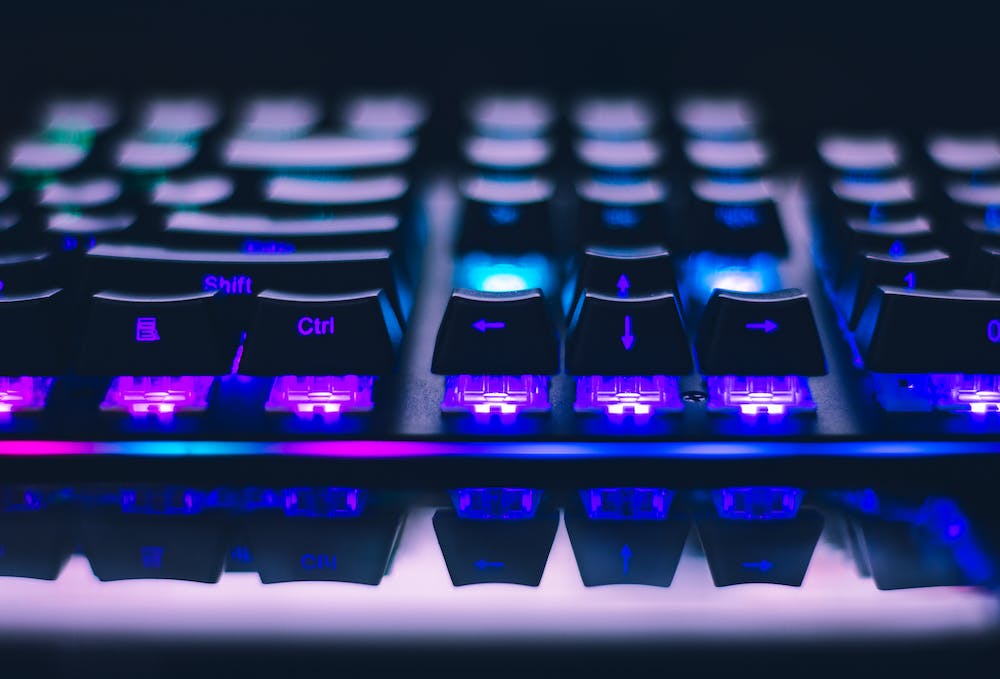
MD5 hashing is a widely used cryptographic hash function that is commonly used in PHP programming. IT takes an input (or message) and returns a fixed-size string of characters, typically a 128-bit hash value. The primary purpose of MD5 hashing is to ensure data integrity by generating a unique hash code for a given input. In this article, we will delve into the workings of MD5 hashing in PHP and explore its various uses.
Before we dive into the details of MD5 hashing, IT‘s essential to understand the concept of hashing. A hash function is a one-way mathematical algorithm that has the ability to convert any given input into a fixed-size string of characters. The output of a hash function is known as a hash code or hash value. One significant characteristic of a hash function is that even a small change in the input will produce a significant change in the output.
Now, let’s discuss how to use MD5 hashing in PHP. The MD5 function is built-in to PHP, so there is no need to import any external libraries or modules. To generate the MD5 hash value of a string, we can use the md5()
function. Here’s an example:
<?php
$input = "Hello, World!";
$hash = md5($input);
echo "MD5 hash of '$input' is: $hash";
?>
In this example, we create a variable named $input
with the value “Hello, World!” and then use the md5()
function to generate its MD5 hash value. The resulting hash value is stored in the variable $hash
. Finally, we print the hash value using the echo
statement.
MD5 hashing is commonly used for various purposes, including:
- Password Hashing: MD5 hashing can be used for securely storing passwords in a database. When a user creates an account or changes their password, the password can be converted to an MD5 hash and stored in the database. This way, even if the database is compromised, the actual passwords remain hidden, as IT is computationally infeasible to reverse-engineer the original password from its hash.
- Data Integrity Verification: MD5 hashing can be used to verify the integrity of data to ensure that IT has not been tampered with. By comparing the hash value of the received data with the expected hash value, we can quickly determine if the data has been altered during transmission or storage.
- Checksum Generation: MD5 hashing is often used to generate checksums for files. A checksum is a unique value that represents the contents of a file. By comparing the checksum of a downloaded file with the original checksum provided by the file’s creator, we can validate the file’s integrity.
- URL Shortening: MD5 hashing can also be used in URL shortening services. When a long URL is submitted, IT can be converted to an MD5 hash, which serves as a unique identifier for the original URL. This allows for shorter and neater URLs.
Despite its widespread usage, MD5 hashing is not without its flaws. IT has been found to have vulnerabilities that make IT susceptible to cryptographic attacks, such as collisions. A collision refers to finding two different inputs that produce the same hash output. Due to these vulnerabilities, MD5 is no longer considered secure for cryptographic purposes.
Now, let’s address some frequently asked questions about MD5 hashing:
Q1. Is MD5 hashing reversible?
No, MD5 hashing is a one-way function, which means that IT is nearly impossible to reverse-engineer the original input from its hash value.
Q2. Can MD5 hashes be decrypted?
No, MD5 hashes cannot be decrypted. Since MD5 is a one-way function, there is no way to retrieve the original input from the hash value.
Q3. Is MD5 secure?
No, MD5 is no longer considered secure for cryptographic purposes. IT has known vulnerabilities that make IT susceptible to attacks.
Q4. Should I still use MD5 hashing?
For cryptographic purposes, IT is recommended to use more secure hashing algorithms, such as bcrypt or SHA-256.
Q5. Can I use MD5 hashing for non-cryptographic purposes?
Yes, MD5 hashing can still be used for non-cryptographic purposes, such as checksum generation or URL shortening, where security is not a critical concern.
In conclusion, MD5 hashing is a widely used cryptographic hash function in PHP programming. Although IT is no longer secure for cryptographic purposes, IT can still be valuable for non-cryptographic use cases where security is not a significant concern. Nonetheless, IT is crucial to use more secure hashing algorithms for applications that require robust data security.