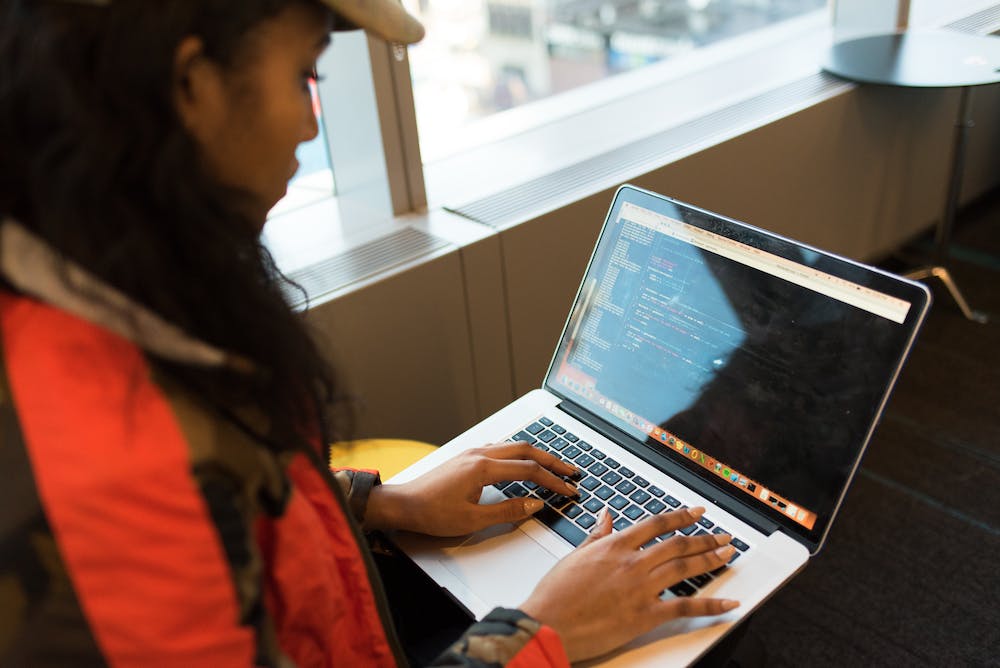
PHP is a popular programming language widely used for web development. One of the important tasks in PHP programming is converting strings to arrays. In this article, we will explore the various techniques to convert strings into arrays and understand the process step by step. Additionally, we will also address some frequently asked questions related to this topic.
Before diving into the conversion process, let’s discuss what arrays and strings are in PHP.
Arrays in PHP are data structures that can store multiple values under a single variable name. Each value in an array is identified by an index, which can be either numeric or associative. Arrays can be used to store different types of data, such as integers, strings, or even other arrays.
On the other hand, strings in PHP are sequences of characters enclosed within quotes. They can contain letters, numbers, symbols, and special characters. String manipulation is a common task in PHP programming, and converting strings to arrays is often required to process or manipulate the data efficiently.
Now, let’s explore some techniques to convert strings into arrays in PHP:
1. Using explode() function:
The explode() function is a commonly used method to split a string into an array based on a specified delimiter. IT takes two parameters – the delimiter and the string to be split. The function returns an array containing the substrings separated by the delimiter.
“`php
$string = “apple,banana,orange”;
$array = explode(“,”, $string);
print_r($array);
?>
“`
Output:
“`html
Array
(
[0] => apple
[1] => banana
[2] => orange
)
“`
2. Using str_split() function:
The str_split() function splits a string into an array of characters. Each character is assigned to a separate element in the resulting array.
“`php
$string = “Hello”;
$array = str_split($string);
print_r($array);
?>
“`
Output:
“`html
Array
(
[0] => H
[1] => e
[2] => l
[3] => l
[4] => o
)
“`
3. Using preg_split() function:
The preg_split() function allows splitting a string into an array using a regular expression pattern as the delimiter. IT is useful when the delimiter is more complex or dynamic.
“`php
$string = “apple1banana2orange3”;
$array = preg_split(“/\d+/”, $string);
print_r($array);
?>
“`
Output:
“`html
Array
(
[0] => apple
[1] => banana
[2] => orange
)
“`
4. Using str_word_count() function with str_word_count() function:
The str_word_count() function counts the number of words in a string and returns them as an array. By passing the second parameter as 1, we can obtain all the individual words in the string as elements of the resulting array.
“`php
$string = “Hello world”;
$array = str_word_count($string, 1);
print_r($array);
?>
“`
Output:
“`html
Array
(
[0] => Hello
[1] => world
)
“`
Now, let’s address some frequently asked questions related to converting strings to arrays in PHP:
Q1. Can we convert a string into an associative array?
Yes, we can convert a string into an associative array as per our requirements. We need to extract the key-value pairs from the string and assign them accordingly while creating the array.
Q2. What is the difference between using explode() and str_split() functions?
The explode() function splits a string into an array based on a specified delimiter, whereas the str_split() function splits a string into an array of characters. The choice between the two depends on the specific use case and the desired outcome.
Q3. Is IT possible to convert a multidimensional string into a multidimensional array?
Yes, IT is possible to convert a multidimensional string into a multidimensional array. We need to apply the appropriate conversion technique based on the structure of the string and the desired array structure.
Q4. Can we convert a comma-separated string into an array without using the explode() function?
Yes, we can use other functions like strtok() or regular expressions to achieve the task of converting a comma-separated string into an array. However, explode() is the simplest and most commonly used approach for this purpose.
Q5. What should be done if the string contains leading or trailing white spaces?
If the string contains leading or trailing white spaces, you can use the trim() function to remove them before performing the conversion. This ensures that unwanted spaces do not affect the resulting array.
In conclusion, understanding how to convert strings to arrays in PHP is a crucial skill for web developers. The different techniques mentioned in this article provide flexibility and efficiency when IT comes to manipulating and processing string data. By exploring these methods and addressing related FAQs, we hope to have provided a comprehensive understanding of this topic.