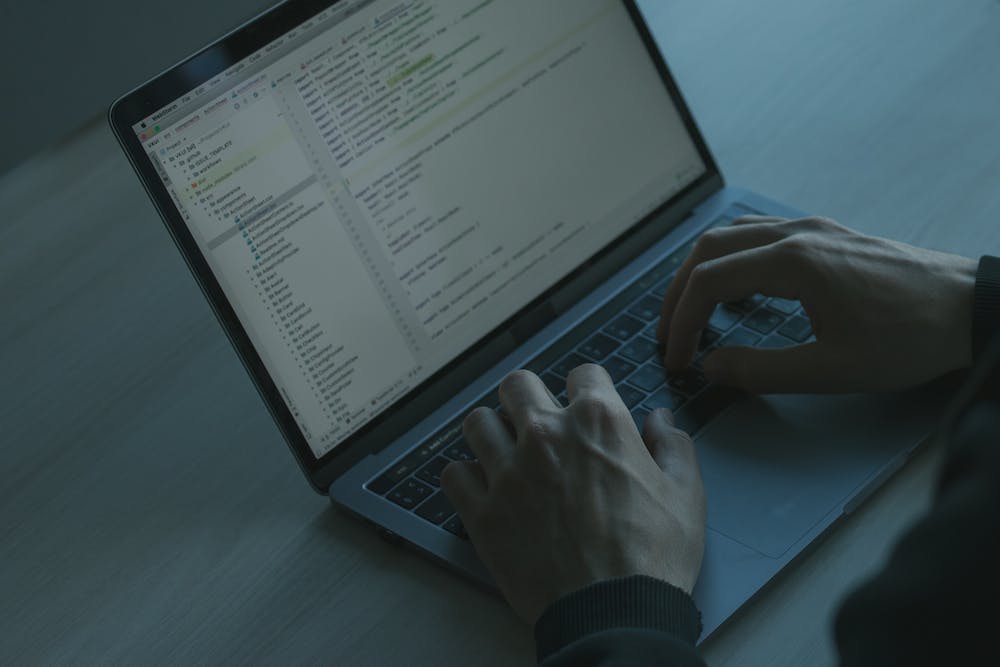
PHP associative arrays are a powerful data structure that allows developers to store key-value pairs. Unlike regular indexed arrays, which use numbers as keys, associative arrays use strings or other data types as keys. This allows for greater flexibility and ease of access to array elements. In this comprehensive guide, we will explore the concept of associative arrays in PHP, understand their syntax, learn various methods to manipulate them, and discover some best practices.
Syntax of PHP Associative Arrays
Before diving deeper into associative arrays, IT‘s important to understand their syntax. Associative arrays in PHP are declared using the array()
function or the shorter []
notation. Each element is specified using the key-value pair format, where the key is enclosed in quotes, followed by a colon, and then the corresponding value. Multiple elements are separated by commas. Let’s look at an example:
$student = array(
"name" => "John Doe",
"age" => 25,
"major" => "computer Science"
);
In this example, the associative array $student
has three key-value pairs: "name" => "John Doe"
, "age" => 25
, and "major" => "computer Science"
.
Accessing Elements of an Associative Array
To access elements of an associative array, you can use the square bracket notation or the array_key_exists()
function. When using the square bracket notation, simply provide the key within the brackets. Here’s an example:
$name = $student["name"];
echo $name; // Output: John Doe
In this example, the value associated with the key "name"
is assigned to the variable $name
. The value can then be printed or used in further processing.
The array_key_exists()
function allows you to check if a specific key exists in an associative array. IT returns true
if the key exists and false
otherwise. Here’s how you can use IT:
if (array_key_exists("age", $student)) {
echo "Age is present";
} else {
echo "Age is not present";
}
In this example, the code checks if the "age"
key exists in the $student
array. Depending on the result, IT prints either "Age is present"
or "Age is not present"
.
Modifying and Adding Elements
Associative arrays in PHP are mutable, meaning you can modify their elements and add new elements even after their initial declaration. To modify an existing element, you can simply use the key to access IT and assign a new value. Here’s an example:
$student["major"] = "Electrical Engineering";
echo $student["major"]; // Output: Electrical Engineering
In this example, the value associated with the key "major"
is changed from "computer Science"
to "Electrical Engineering"
.
If you want to add a new element to an associative array, you can do so by providing a new key-value pair. Here’s an example:
$student["year"] = 3;
echo $student["year"]; // Output: 3
In this example, a new key-value pair "year" => 3
is added to the $student
array.
Looping through an Associative Array
To iterate over all the elements of an associative array, you can use a foreach
loop. The foreach
loop automatically assigns the key and value of each element to specified variables. Here’s an example:
foreach ($student as $key => $value) {
echo "$key: $value\n";
}
In this example, the foreach
loop iterates over each key-value pair in the $student
array. IT assigns the key to the variable $key
and the value to the variable $value
. The loop body then echoes the key-value pair.
Frequently Asked Questions (FAQs)
Q: Can I use numeric keys in associative arrays?
A: Yes, PHP allows the use of numeric keys in associative arrays. However, IT is recommended to use numeric keys only for indexed arrays to avoid confusion and potential inconsistencies.
Q: How can I check if an associative array is empty?
A: You can use the empty()
function to check if an associative array is empty. IT returns true
if the array has no elements and false
otherwise. Here’s an example:
if (empty($student)) {
echo "The array is empty";
} else {
echo "The array is not empty";
}
In this example, the code checks if the $student
array is empty and prints the corresponding message.
Q: Can I nest associative arrays?
A: Yes, IT is possible to nest associative arrays within other associative arrays in PHP. This allows for the creation of multi-dimensional data structures to represent complex relationships or hierarchies.
Understanding PHP associative arrays is essential for effective manipulation and management of data. By mastering the concepts and techniques explained in this guide, you’ll be able to leverage the full power of associative arrays in your PHP projects.