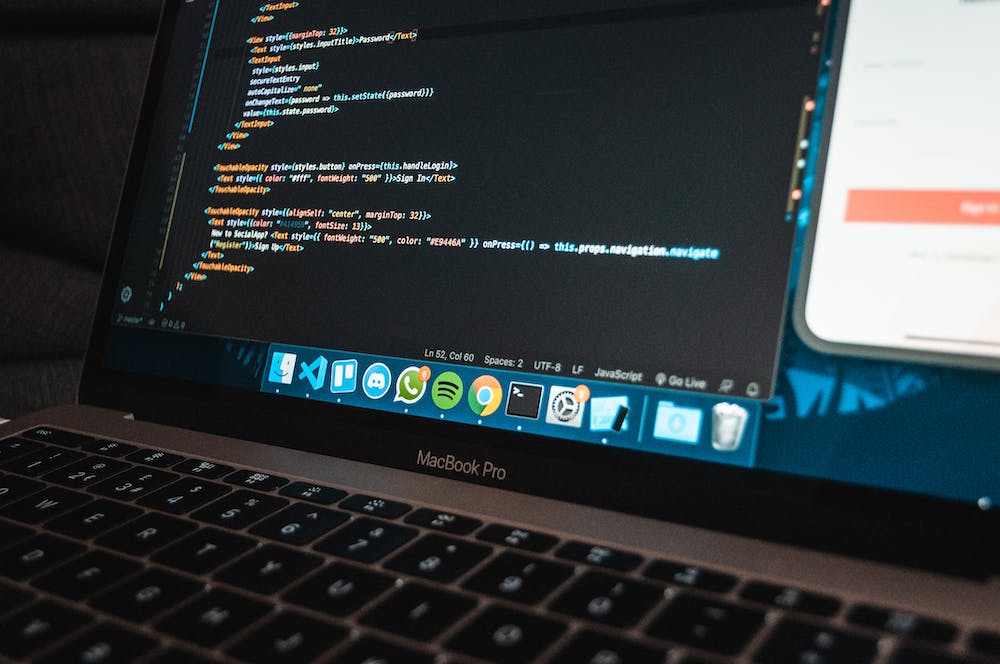
Understanding PHP Arrays: A Beginner’s Guide
HTML Headings:
Introduction
What is PHP?
What are Arrays in PHP?
Creating an Array
Numeric Arrays
Associative Arrays
Multidimensional Arrays
Manipulating Arrays
Accessing Array Elements
Adding and Removing Elements
Modifying Array Elements
Looping Through Arrays
Using the foreach Loop
Using the for Loop
Conclusion
FAQs
Introduction:
PHP is a widely-used programming language that is particularly suited for web development. IT is versatile, powerful, and easy to learn. One of the most fundamental concepts in PHP is arrays, which allow you to store multiple values in a single variable. Arrays come in handy when you need to organize and manipulate large sets of data efficiently. In this beginner’s guide, we will explore the basics of PHP arrays, including how to create, manipulate, and loop through them.
What is PHP?
PHP stands for “Hypertext Preprocessor” and is an open-source server-side scripting language. IT is widely used for web development and embedded into HTML code. With PHP, you can dynamically generate web pages, handle form data, manage cookies and sessions, perform database operations, and much more. PHP is compatible with all major operating systems and can be easily integrated with various databases, including MySQL, PostgreSQL, and Oracle.
What are Arrays in PHP?
In PHP, an array is a special type of variable that can hold multiple values. These values can be of the same or different data types, such as strings, numbers, booleans, or even other arrays. Arrays in PHP are incredibly flexible and can be used to store and manipulate various types of data, from simple lists to complex data structures.
Creating an Array:
There are different types of arrays in PHP, including numeric arrays, associative arrays, and multidimensional arrays.
Numeric Arrays:
A numeric array is the simplest and most common type of array in PHP. IT is a collection of elements, where each element is assigned a numerical index starting from 0. To create a numeric array, you can use the array() function and specify the values within the parentheses, separated by commas.
Example:
$fruits = array("apple", "banana", "orange");
In this example, the variable $fruits is an array that contains three elements: “apple”, “banana”, and “orange”. The elements can be accessed using their respective indexes, such as $fruits[0], $fruits[1], and $fruits[2].
Associative Arrays:
An associative array, also known as a key-value pair array, allows you to assign a specific key to each element instead of a numerical index. The keys can be strings or numbers, and they are used to access the corresponding values in the array. To create an associative array, you can use the array() function and specify the key-value pairs within the parentheses, using the => (double arrow) operator.
Example:
$student = array("name" => "John", "age" => 25, "major" => "computer Science");
In this example, the variable $student is an associative array with three elements: “name”, “age”, and “major”. To access the values, you can use the corresponding keys, such as $student[“name”], $student[“age”], and $student[“major”].
Multidimensional Arrays:
A multidimensional array is an array of arrays, where each element can have its own set of keys and values. IT allows you to represent complex data structures, such as tables or matrices. To create a multidimensional array, you can nest arrays within arrays.
Example:
$matrix = array(
array(1, 2, 3),
array(4, 5, 6),
array(7, 8, 9)
);
In this example, the variable $matrix is a multidimensional array that represents a 3×3 matrix. To access the elements, you need to specify both the row index and the column index, such as $matrix[0][0], $matrix[1][2], and $matrix[2][1].
Manipulating Arrays:
Once you have created an array, you can manipulate IT in various ways, such as accessing elements, adding and removing elements, and modifying existing elements.
Accessing Array Elements:
To access a specific element in an array, you can use the corresponding index or key. For numeric arrays, you can use the index within square brackets ([]), and for associative arrays, you can use the key within square brackets ([]).
Example:
$fruits = array("apple", "banana", "orange");
echo $fruits[1]; // Output: banana
$student = array("name" => "John", "age" => 25, "major" => "computer Science");
echo $student["age"]; // Output: 25
In this example, $fruits[1] returns the element with index 1 from the $fruits array, which is “banana”. $student[“age”] returns the value associated with the key “age” from the $student array, which is 25.
Adding and Removing Elements:
To add elements to an existing array, you can use the array_push() or assignment (=) operator. The array_push() function adds one or more elements to the end of an array, while the assignment operator assigns a value to a specific index or key.
Example:
$fruits = array("apple", "banana");
array_push($fruits, "orange");
$fruits[3] = "grape";
print_r($fruits);
In this example, “orange” is added to the $fruits array using the array_push() function, and “grape” is assigned to the index 3 using the assignment operator. The print_r() function displays the contents of the array.
To remove elements from an array, you can use the unset() function or the assignment operator with the null value.
Example:
$fruits = array("apple", "banana", "orange");
unset($fruits[1]);
print_r($fruits);
In this example, the element with index 1 (“banana”) is removed from the $fruits array using the unset() function. The print_r() function displays the modified array without the removed element.
Modifying Array Elements:
To modify the value of a specific element in an array, you can assign a new value to the corresponding index or key.
Example:
$fruits = array("apple", "banana", "orange");
$fruits[1] = "pear";
print_r($fruits);
In this example, the value of the element with index 1 (“banana”) in the $fruits array is changed to “pear”. The print_r() function displays the modified array.
Looping Through Arrays:
Looping through an array allows you to perform repetitive tasks for each element in the array. PHP provides different looping mechanisms, such as the foreach loop and the for loop.
Using the foreach Loop:
The foreach loop is particularly useful for iterating over arrays, as IT automatically assigns each element to a variable in each iteration.
Example:
$fruits = array("apple", "banana", "orange");
foreach ($fruits as $fruit) {
echo $fruit;
}
In this example, the foreach loop iterates through each element in the $fruits array and assigns IT to the variable $fruit. The echo statement outputs each fruit on a new line.
Using the for Loop:
The for loop is a more traditional looping mechanism that allows you to specify the start, condition, and increment/decrement values explicitly.
Example:
$fruits = array("apple", "banana", "orange");
for ($i = 0; $i < count($fruits); $i++) {
echo $fruits[$i];
}
In this example, the for loop iterates through the indices of the $fruits array, starting from 0 and increasing by 1 until the condition $i < count($fruits) is no longer true. The echo statement outputs each fruit on a new line.
Conclusion:
In this beginner’s guide, we have explored the fundamentals of PHP arrays. We learned about different types of arrays, such as numeric arrays, associative arrays, and multidimensional arrays. We also discovered how to create, manipulate, and loop through arrays using various techniques. Understanding arrays is crucial for anyone starting their journey in PHP, as they are essential for storing and manipulating data efficiently.
FAQs:
Q: Can arrays in PHP store different types of values?
A: Yes, arrays in PHP can store values of different data types, such as strings, numbers, booleans, or even other arrays.
Q: Is IT possible to change the size of an array dynamically?
A: Yes, in PHP, arrays can be resized dynamically by adding or removing elements using functions like array_push() or unset().
Q: Is IT possible to have arrays within arrays in PHP?
A: Yes, PHP supports multidimensional arrays, which are arrays of arrays. This allows for the creation of complex data structures.
Q: What is the difference between numeric arrays and associative arrays?
A: In numeric arrays, elements are assigned numerical indexes, starting from 0. In associative arrays, elements are assigned specific keys that can be strings or numbers.
Q: How can I loop through an array in PHP?
A: PHP provides looping mechanisms such as the foreach loop and the for loop, which allow you to iterate through array elements and perform operations on them.
Q: Can I modify the elements of an array in PHP?
A: Yes, you can modify the elements of an array in PHP by assigning new values to specific indexes or keys.
Remember, arrays are an essential part of PHP programming and mastering them will greatly enhance your skills as a web developer. Practice creating, manipulating, and looping through arrays to become proficient in their usage. Happy coding!