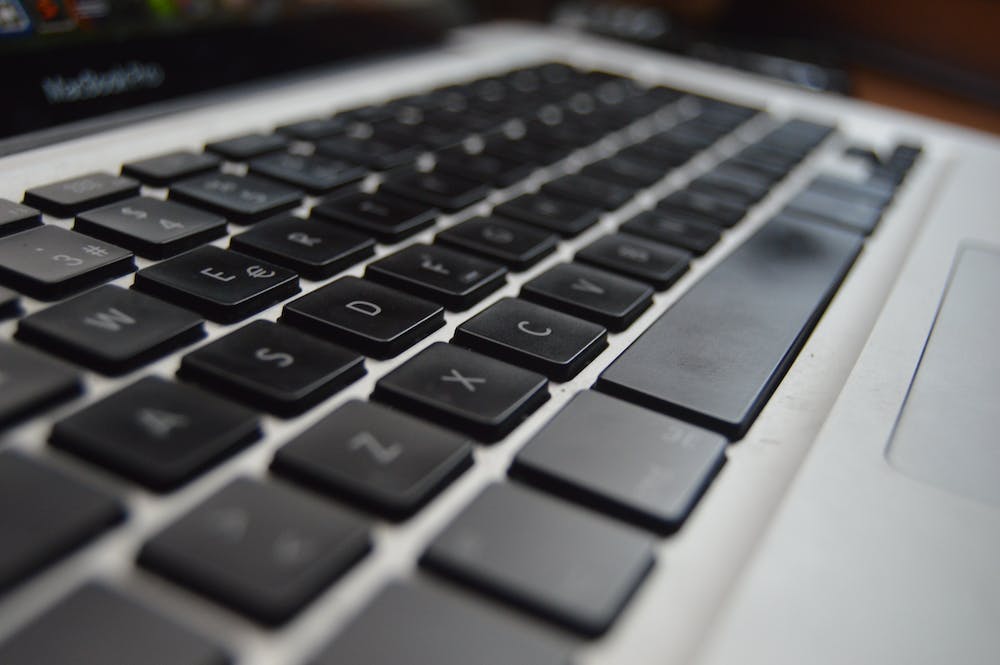
The mysqli_real_escape_string() function is a crucial tool for building secure database queries in PHP. When dealing with user input that is inserted into database queries, IT is essential to protect against SQL injection attacks and ensure data integrity. In this guide, we will explore what mysqli_real_escape_string() is, how IT works, and how to use IT effectively to safeguard your database queries.
If you are working with a MySQL database, you are likely familiar with PHP’s MySQLi extension. This extension is designed to offer improved performance and enhanced functionality over the older MySQL extension.
One of the most critical aspects of building secure database queries is sanitizing user input to prevent SQL injection vulnerabilities. SQL injection occurs when an attacker can manipulate an SQL query by inserting malicious code into user input, leading to unauthorized access or unintended actions on your database.
Understanding mysqli_real_escape_string()
mysqli_real_escape_string() is a PHP function specifically designed to escape special characters in a string before IT is used in an SQL statement. By escaping these characters, we prevent them from being interpreted as SQL commands and ensure that they are treated as literal values.
The function takes two parameters: the MySQL database connection object and the string to be escaped. IT returns the escaped string, which can then be safely used in a database query.
Using mysqli_real_escape_string()
To use mysqli_real_escape_string() effectively, you must follow these steps:
- Establish a connection to the MySQL database using the mysqli_connect() function.
- Validate and sanitize any user input that will be included in the query.
- Escape the user input using mysqli_real_escape_string() before incorporating IT into the query.
- Execute the query using mysqli_query().
- Clean up by closing the database connection using mysqli_close().
Example:
// Step 1: Connect to the MySQL database
$connection = mysqli_connect("localhost", "username", "password", "database");
// Step 2: Validate and sanitize user input
$username = $_POST["username"];
$username = mysqli_real_escape_string($connection, $username);
// Step 3: Escape the user input
$query = "SELECT * FROM users WHERE username = '" . $username . "'";
// Step 4: Execute the query
$result = mysqli_query($connection, $query);
// Step 5: Close the database connection
mysqli_close($connection);
Frequently Asked Questions
Q: Why should I use mysqli_real_escape_string()? Can’t I just use prepared statements?
A: While prepared statements offer a more robust solution for preventing SQL injection, mysqli_real_escape_string() remains an effective method for escaping user input when prepared statements are not feasible or practical.
Q: Is mysqli_real_escape_string() sufficient to protect against all types of SQL injection?
A: No, mysqli_real_escape_string() is not a foolproof solution but provides a solid line of defense in most cases. IT is still essential to regularly review your code and consider other security measures to protect against potential vulnerabilities.
Q: Can I use mysqli_real_escape_string() on all types of data?
A: mysqli_real_escape_string() is designed to escape characters in text strings. IT may not be suitable for other data types such as integers or dates. In such cases, you should use appropriate validation and sanitization techniques relevant to the data type.
Q: Can I use mysqli_real_escape_string() on data already stored in the database?
A: No, mysqli_real_escape_string() is intended for use on user input before IT is included in a query. IT is not necessary or appropriate for escaping data that has already been passed through the function.
In conclusion, mysqli_real_escape_string() is a valuable tool for securing your database queries in PHP. By escaping special characters, you can prevent SQL injection attacks and maintain the integrity of your data. While IT is not a substitute for other security measures, understanding and using this function correctly can significantly enhance the security of your applications.