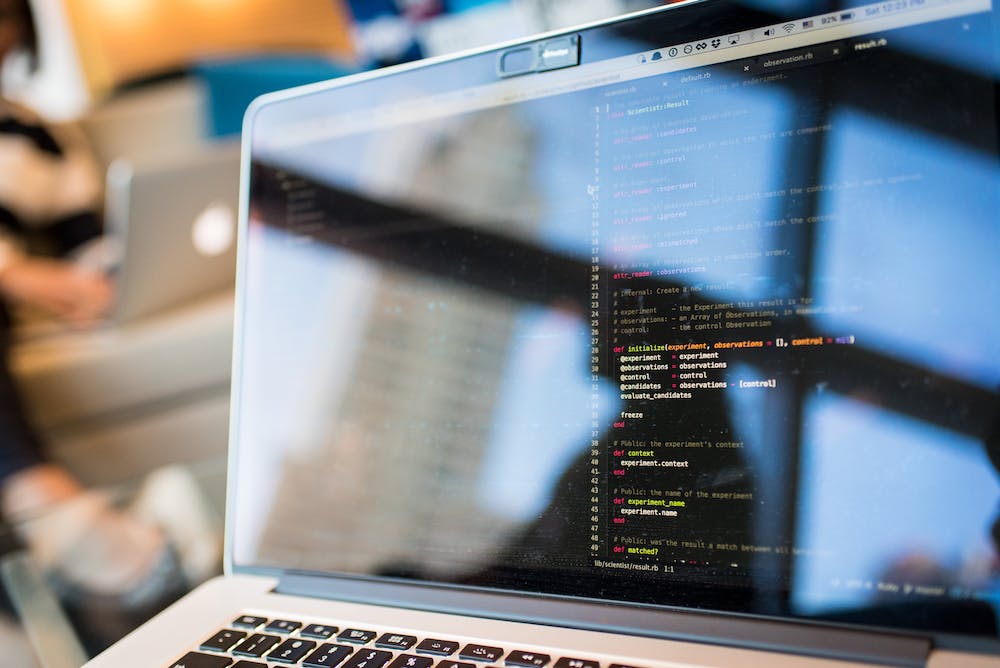
Laravel is a widely popular PHP framework that simplifies web application development. One of its key features is its caching system, which helps improve performance by storing frequently used data in memory for quick retrieval. Understanding how Laravel cache works is essential for optimizing your application’s performance and delivering a seamless user experience. This guide will introduce you to Laravel cache, its benefits, and how to effectively implement IT in your projects.
What is Laravel Cache?
Laravel Cache is a mechanism that allows you to store data in memory for quick access. When a user requests certain data from your application, Laravel checks if IT has already been cached. If IT has, the cached data is retrieved and returned, eliminating the need for time-consuming database queries or expensive computations. If the data is not cached, Laravel retrieves IT from the original source, caches IT for future use, and returns IT to the user.
Why Use Laravel Cache?
Implementing cache in your Laravel applications offers several benefits:
1. Improved Performance: By caching frequently used data, you can significantly reduce the load on your database and improve your application’s overall performance. Caching eliminates the need for repetitive and time-consuming database queries, resulting in faster response times.
2. Reduced Database Load: Caching ensures that your database is not overwhelmed with frequent requests for the same data. By storing commonly accessed data in memory, you minimize the number of database queries, thereby reducing the load on your database server.
3. Better Scalability: Caching helps your application scale by efficiently handling increased traffic. As the number of users accessing your application grows, a well-implemented cache can handle more requests without impacting performance. With cache, you can deliver a responsive application even during peak traffic periods.
4. Enhanced User Experience: Faster response times lead to a better user experience. By serving cached data, you can ensure that users receive the information they need quickly, reducing wait times and improving overall satisfaction.
How to Use Laravel Cache?
Laravel provides a simple and intuitive API for working with cache. The framework supports various cache drivers, including file, database, Redis, and Memcached, allowing you to choose the most suitable caching solution for your application.
To start using cache in Laravel, you need to follow these steps:
1. Configure Cache Driver: Laravel uses the “config/cache.php” file to manage cache configuration. Open this file and set the appropriate cache driver based on your requirements.
2. Storing Data in Cache: To store data in cache, you can use the “put” method provided by the cache facade. For example, to cache the result of a database query, you can write:
$users = Cache::remember('users', 60, function () {
return DB::table('users')->get();
});
In the above code, the “remember” method checks if the “users” data is cached. If IT is, the cached data is returned. If not, the closure function is executed, and the result is cached for 60 seconds.
3. Retrieving Data from Cache: To retrieve data from the cache, you can use the “get” method provided by the cache facade. The method accepts the cache key as an argument and returns the corresponding data if found. If the data is not cached or has expired, the method returns null.
$users = Cache::get('users');
4. Forgetting Data from Cache: Laravel also allows you to manually remove data from the cache using the “forget” method. This can be useful when you want to invalidate cache after a certain event.
Cache::forget('users');
These are the basic steps to implement cache in your Laravel application. By utilizing cache effectively, you can fine-tune the performance of your application and provide a seamless user experience.
Frequently Asked Questions (FAQs)
Q1: Can I use different cache drivers within the same Laravel application?
A1: Yes, you can use multiple cache drivers simultaneously in a Laravel application. By configuring different cache drivers, you can cache different types of data using the most appropriate caching solution.
Q2: How do I clear the entire cache in Laravel?
A2: Laravel provides an artisan command to clear the entire cache. Simply open your command line interface, navigate to your Laravel project, and run the “php artisan cache:clear” command.
Q3: Can I cache database query results using Laravel Cache?
A3: Absolutely! Laravel Cache is especially useful for caching the results of database queries. By caching query results, you can avoid hitting the database unnecessarily, resulting in noticeable performance improvements.
Q4: How long does cached data persist in Laravel?
A4: The duration for which data remains cached in Laravel depends on how IT is configured. By default, cache data lasts for as long as you specify when storing IT. However, you can also set an expiration time to automatically invalidate the cache.
Q5: Can I cache entire views in Laravel?
A5: Yes, Laravel allows you to cache entire views effortlessly. You can use the “cache” method provided by the framework to cache the rendering of views, improving the performance of pages that are not frequently modified.
Laravel cache is an essential tool for optimizing the performance of your applications. By storing frequently accessed information in memory, you can significantly reduce the load on your database and provide a seamless user experience. Understanding how to leverage Laravel cache and its various features can empower you to build high-performing applications that scale effortlessly.