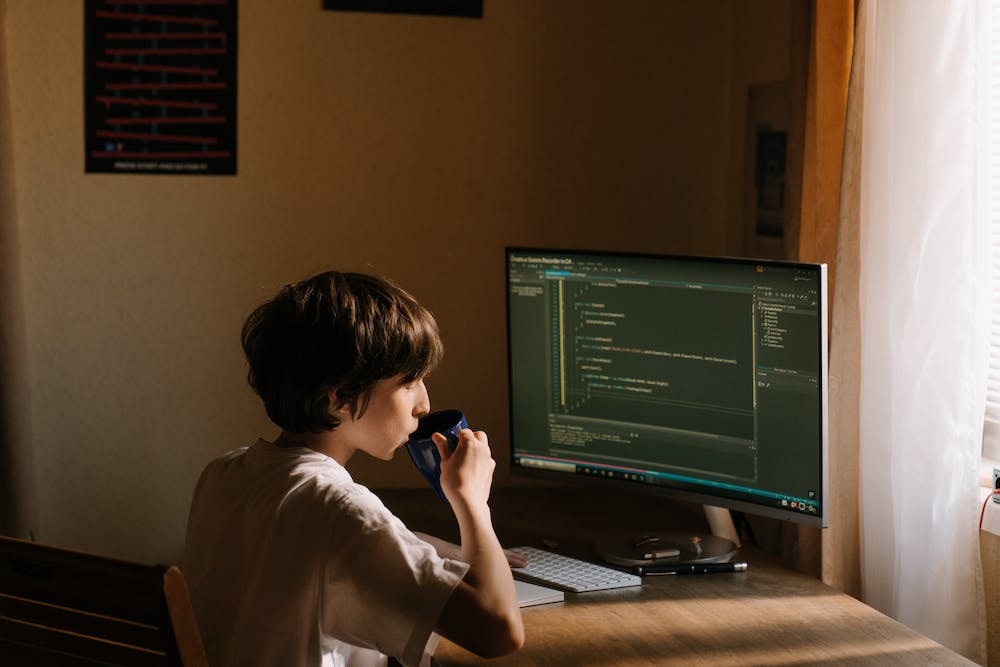
Laravel is a popular PHP framework that provides an elegant and efficient way to build web applications. IT offers a wide range of features and functionalities that can help developers create robust and scalable applications. One of the key components of Laravel is its API, which allows developers to easily build and consume web services. In this article, we will provide a comprehensive guide to understanding Laravel API for beginners, covering the basics, the key concepts, and some frequently asked questions.
Basics of Laravel API
Before diving into the details of Laravel API, IT is important to have a basic understanding of APIs in general. An API, or Application Programming Interface, is a set of rules and protocols that allows different software applications to communicate with each other. IT defines how certain functionalities of one application can be accessed and used by another application, making IT easier to build complex software systems by combining different services and modules.
Laravel API allows developers to expose their application’s functionality and data to other applications or clients, such as mobile apps or third-party systems. This can be done by defining routes, controllers, and endpoints to handle requests and return data in a desired format, such as JSON or XML.
Key Concepts of Laravel API
There are several key concepts in Laravel API that developers should be familiar with:
Routes
Routes define the URLs or endpoints that the API exposes. They determine which controller method should be called when a request is made to a particular endpoint. Routes can be defined in the routes/api.php
file in a Laravel application.
Controllers
Controllers are responsible for handling the logic of the API endpoints. They receive requests from the routes and perform the necessary actions, such as fetching data from a database, processing IT, and returning a response. Controllers can be created using the php artisan make:controller
command in Laravel.
Middleware
Middleware provides a convenient way to filter and modify HTTP requests and responses. IT sits between the route and the controller, allowing developers to perform actions such as authentication, validation, or logging before or after the request is handled. Laravel provides several built-in middleware, but developers can also create custom middleware for specific requirements.
Authentication
Authentication is an important aspect of building secure APIs. Laravel provides various authentication methods, such as API tokens, OAuth, or JWT (JSON Web Tokens), to authenticate and authorize users accessing the API. This ensures that only authorized users can make requests to protected endpoints.
Serializers
Serializers control how data is serialized and transformed before IT is returned as a response. Laravel provides a default serializer that converts data to JSON format, but developers can customize the serialization process based on their requirements.
Frequently Asked Questions (FAQs)
Q: How can I create a new API route in Laravel?
A: To create a new API route in Laravel, you can define IT in the routes/api.php
file. For example, to create a route that handles GET requests to /api/users
, you can use the following code:
Route::get('/users', 'UserController@index');
Q: How do I pass parameters to an API endpoint?
A: You can pass parameters to an API endpoint in Laravel by defining them in the route definition. For example, to retrieve a specific user by their ID, you can define the route as follows:
Route::get('/users/{id}', 'UserController@show');
In the controller method, you can access the parameter using the request object:
public function show($id)
{
$user = User::find($id);
// Process and return the user data
}
Q: How can I protect my API endpoints with authentication?
A: Laravel provides several authentication methods for protecting API endpoints. One common approach is to use API tokens, which are unique tokens associated with each user. These tokens can be generated and stored in the user’s database record, and then sent with each API request as an authorization header or a query parameter. Laravel provides built-in methods to generate, verify, and revoke API tokens.
Q: Can I customize the response format of my API?
A: Yes, you can customize the response format of your API in Laravel. By default, Laravel serializes data as JSON, but you can modify this behavior by creating a custom serializer or using a third-party package. Laravel allows you to transform and format the data before IT is returned as a response, giving you full control over the output format.
Q: How can I handle errors and exceptions in my API?
A: Laravel provides a convenient way to handle errors and exceptions in your API. You can use the built-in exception handling mechanisms to catch and handle different types of exceptions, such as model not found exceptions or validation errors. Laravel also allows you to define custom exception handlers to provide meaningful error responses to your API clients.
In conclusion, Laravel API provides a powerful and flexible way to build and consume web services. By understanding the basics, key concepts, and best practices of Laravel API, developers can create robust and secure APIs that integrate seamlessly with other applications. With its rich set of features and extensive documentation, Laravel is a great choice for building APIs and web applications.