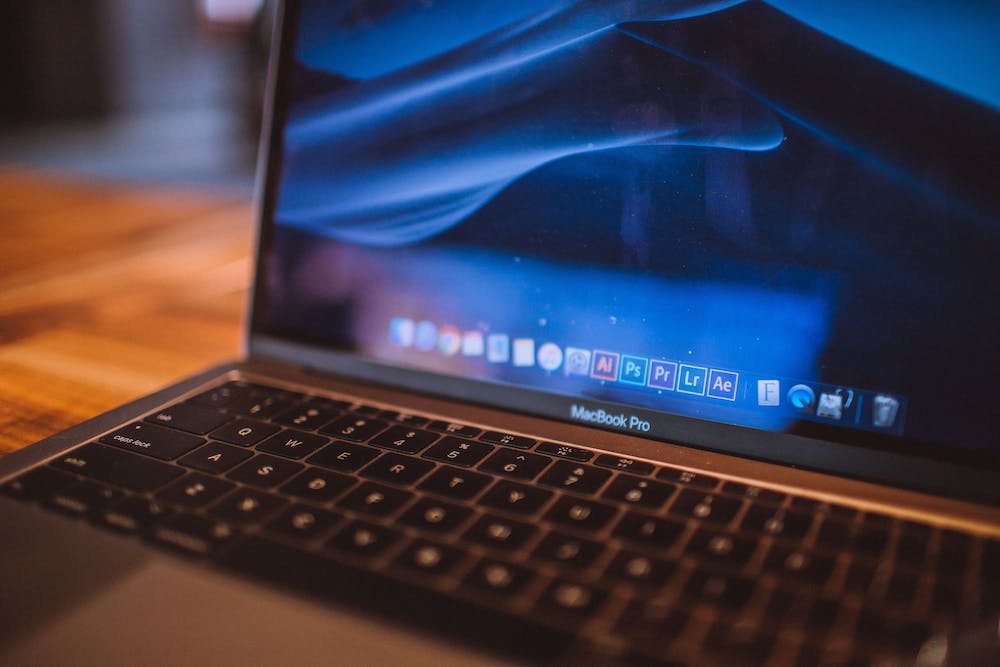
Uncovering the secrets of K Means Clustering is a fascinating journey into the world of unsupervised machine learning. With its ability to automatically classify data into groups, K Means Clustering has become an essential tool for data analysis and pattern recognition. In this article, we will delve into the inner workings of K Means Clustering and reveal the Python code that powers this powerful algorithm, all available on GitHub!
The Basics of K Means Clustering
At its core, K Means Clustering is a type of unsupervised learning algorithm that aims to partition a set of data points into K distinct, non-overlapping clusters. The algorithm accomplishes this by iteratively assigning each data point to the closest cluster centroid and then recalculating the centroids based on the mean of the data points assigned to each cluster. This process continues until the centroids no longer change significantly, or a specified number of iterations is reached.
One of the key advantages of K Means Clustering is its simplicity and efficiency, making IT well-suited for large datasets. However, it is important to note that K Means Clustering requires the number of clusters (K) to be specified in advance, which can be a limitation in some cases. Additionally, the algorithm is sensitive to the initial choice of centroids, and the clusters produced can vary depending on the starting centroids.
The Python Code Behind K Means Clustering
Thanks to the open-source nature of Python, the code for implementing K Means Clustering is readily available and easily accessible on GitHub. The popular machine learning library, scikit-learn, provides a robust implementation of the K Means algorithm, allowing users to leverage its power with just a few lines of code.
Let’s take a look at a simple example of how K Means Clustering can be implemented using Python and scikit-learn:
# Importing the necessary libraries
import numpy as np
from sklearn.cluster import KMeans
from sklearn.datasets import make_blobs
import matplotlib.pyplot as plt
# Generating random data
X, _ = make_blobs(n_samples=300, centers=4, cluster_std=0.60, random_state=0)
# Applying K Means Clustering
kmeans = KMeans(n_clusters=4)
kmeans.fit(X)
y_kmeans = kmeans.predict(X)
# Visualizing the results
plt.scatter(X[:, 0], X[:, 1], c=y_kmeans, cmap='viridis')
centers = kmeans.cluster_centers_
plt.scatter(centers[:, 0], centers[:, 1], c='black', s=200, alpha=0.5)
plt.show()
In the above code snippet, we first import the necessary libraries, including numpy, scikit-learn, and matplotlib for data manipulation, K Means Clustering, and visualization, respectively. We then generate random data using the make_blobs function from scikit-learn, which creates a specified number of clusters of data points.
Next, we instantiate a KMeans object with the desired number of clusters and fit it to the data. The algorithm then assigns each data point to the closest cluster, and we can visualize the results by plotting the data points and cluster centroids using matplotlib.
Gaining Access to K Means Clustering Code on GitHub
For those interested in exploring the inner workings of K Means Clustering in more detail, the GitHub repository for scikit-learn provides the source code for the KMeans implementation. This allows users to gain a deeper understanding of the algorithm’s implementation and make custom modifications if necessary.
By accessing the GitHub repository, users can browse the code, documentation, and discussions related to K Means Clustering and other machine learning algorithms. This open development model fosters collaboration and encourages community contributions, making it an invaluable resource for aspiring data scientists and machine learning enthusiasts.
Conclusion
K Means Clustering is a powerful algorithm that has proven to be invaluable in the field of data analysis and pattern recognition. By leveraging the Python code available on GitHub, users can gain insight into the inner workings of K Means Clustering and harness its capabilities for their own projects. Whether it’s identifying customer segments, clustering similar documents, or detecting anomalies in data, K Means Clustering offers a versatile and efficient solution.
FAQs
Q: What are the limitations of K Means Clustering?
A: K Means Clustering requires the number of clusters (K) to be specified in advance, which can be a limitation in some cases. Additionally, the algorithm is sensitive to the initial choice of centroids, and the clusters produced can vary depending on the starting centroids.
Q: How do I choose the right number of clusters for K Means Clustering?
A: Selecting the optimal number of clusters can be done using techniques such as the elbow method or silhouette score. These methods help identify the number of clusters that best represents the underlying structure of the data.
Q: Can K Means Clustering be applied to high-dimensional data?
A: Yes, K Means Clustering can be applied to high-dimensional data, but it is important to be mindful of the curse of dimensionality. Preprocessing techniques such as dimensionality reduction or feature selection may be necessary to improve the algorithm’s performance.
Q: Can I contribute to the development of K Means Clustering on GitHub?
A: Yes, the GitHub repository for scikit-learn is open to contributions from the community. Whether it’s reporting issues, submitting bug fixes, or proposing new features, users can actively participate in the development and improvement of K Means Clustering.
Q: Where can I find the GitHub repository for scikit-learn?
A: The GitHub repository for scikit-learn can be found at https://github.com/scikit-learn/scikit-learn.