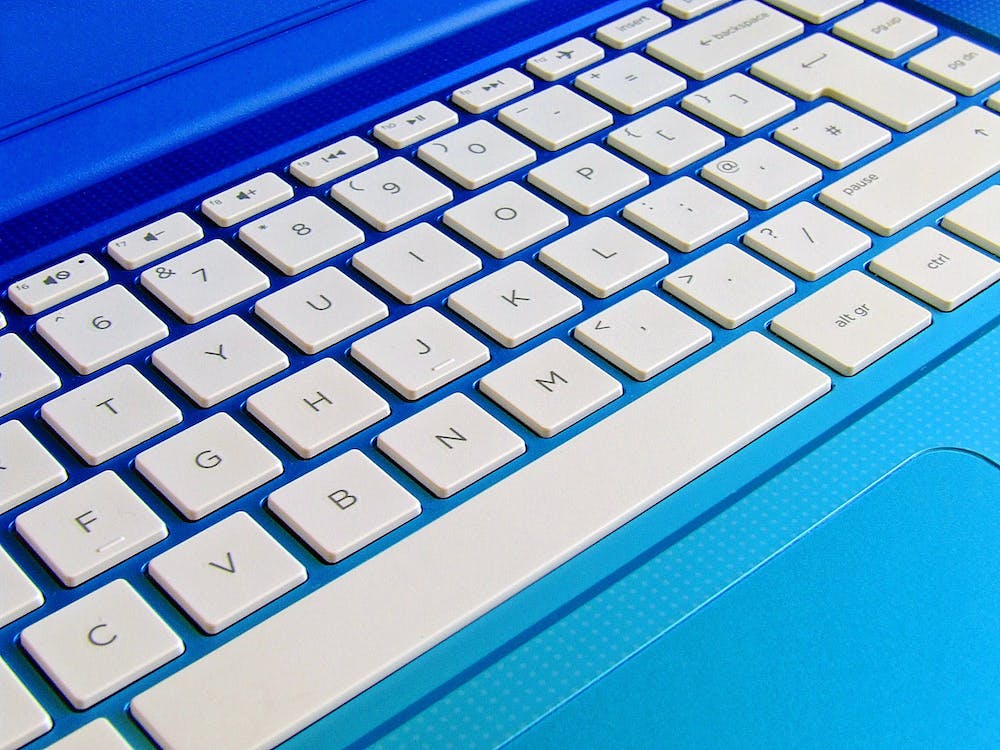
PHP is a widely used programming language for building dynamic web applications. One of the most commonly used features of PHP is the foreach
loop, especially when working with arrays. Despite its widespread use, there are still hidden secrets and best practices that many developers are not aware of. In this article, we will uncover these hidden secrets and show you how to make the most out of the foreach
loop when working with arrays in PHP.
The Basics of the foreach Loop
Before we dive into the hidden secrets, let’s start with the basics. The foreach
loop in PHP is used to iterate over each element of an array. IT has a simple syntax:
foreach ($array as $value) {
// code to be executed for each element
}
Here, $array
is the array we want to iterate over, and $value
is the current element of the array that the loop is processing. This makes it easy to perform operations on each element of the array without the need for manual index management.
Accessing Keys and Values
One of the hidden secrets of the foreach
loop is that it can also be used to access both the keys and the values of an array. By slightly modifying the syntax, we can access both the key and the value of each array element:
foreach ($array as $key => $value) {
// code to be executed for each element
}
With this syntax, $key
will contain the key of the current array element, and $value
will contain the value. This allows for more flexibility when working with associative arrays, where both the keys and the values are important.
Iterating over Nested Arrays
Another hidden secret of the foreach
loop is its ability to iterate over nested arrays. When working with multi-dimensional arrays, the foreach
loop can be used to iterate over the outer array and then use another foreach
loop to iterate over the inner arrays:
foreach ($outerArray as $innerArray) {
foreach ($innerArray as $value) {
// code to be executed for each element
}
}
This allows for easy traversal of complex data structures and is especially useful when working with data retrieved from APIs or databases.
Skipping and Breaking the Loop
While iterating over an array, there are times when you may want to skip certain elements or stop the loop altogether based on a condition. The continue
and break
statements can be used to achieve this within a foreach
loop:
foreach ($array as $value) {
if ($value == 'skip') {
continue; // skip this iteration and move to the next element
}
if ($value == 'stop') {
break; // stop the loop altogether
}
// code to be executed for each element
}
These statements provide fine-grained control over the loop, allowing you to tailor the iteration to your specific needs.
Using References for Better Performance
When iterating over large arrays, the default behavior of the foreach
loop is to make a copy of each element. For small arrays, this is not an issue, but for larger arrays, it can impact performance. To address this, you can use references within the foreach
loop to work directly with the original array elements:
foreach ($array as &$value) {
// code to be executed for each element
}
By adding an ampersand (&) before the $value
variable, we are telling PHP to use a reference to the original array element rather than creating a copy. This can lead to significant performance improvements when dealing with large arrays.
Conclusion
The foreach
loop is a powerful feature of PHP for iterating over arrays, but its full potential is often overlooked. By uncovering these hidden secrets and best practices, you can make the most out of the foreach
loop and improve the performance and readability of your code when working with arrays in PHP.
FAQs
Q: Can I use the foreach
loop with objects in PHP?
A: Yes, the foreach
loop can be used to iterate over the public properties of an object in PHP.
Q: Are there any performance considerations when using the foreach
loop with large arrays?
A: Yes, for large arrays, using references within the foreach
loop can lead to performance improvements by avoiding unnecessary copying of array elements.
Q: Can the foreach
loop be used with associative arrays in PHP?
A: Yes, the foreach
loop can iterate over both indexed and associative arrays, providing access to keys and values if needed.