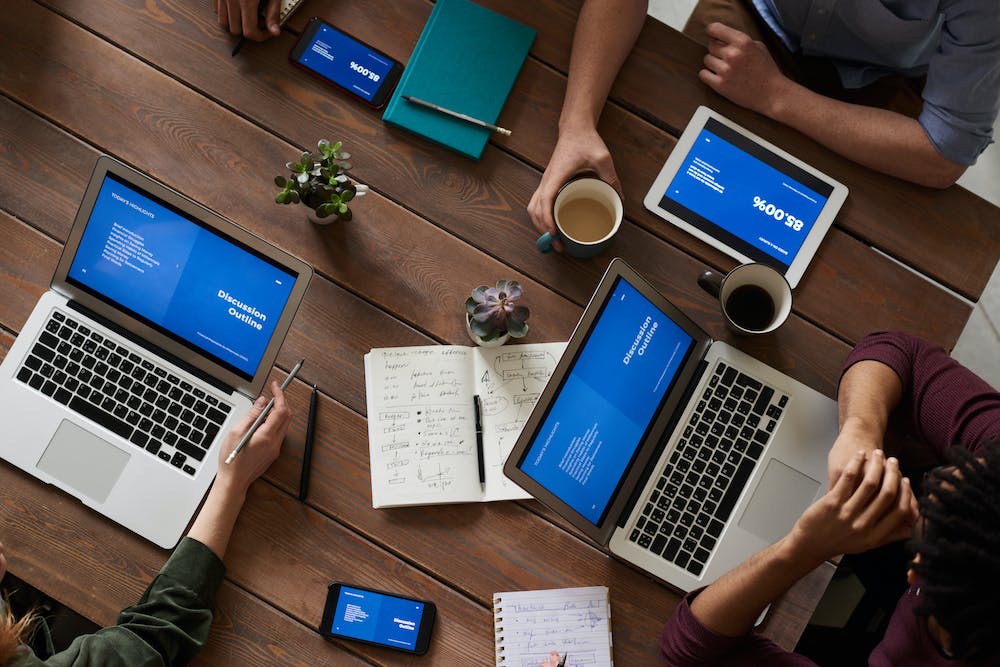
Python is a powerful and versatile programming language that is widely used in various fields such as web development, data analysis, artificial intelligence, and more. One of the key benefits of Python is its simplicity and readability, making IT a great choice for beginners and experienced programmers alike. In this article, we will explore how to master the area of circle program in Python like a pro, uncovering the ultimate shortcut to make your coding more efficient and effective.
The Basics of Calculating the Area of a Circle
Before we dive into the Python program, let’s quickly review the basic formula for calculating the area of a circle. The area of a circle is given by the formula:
Area = π * r2
Where π (pi) is a constant approximately equal to 3.14159, and r is the radius of the circle. This simple formula allows us to calculate the area of any circle by simply knowing its radius.
writing the Program in Python
Now that we understand the basic formula for the area of a circle, let’s see how we can write a Python program to calculate IT. Here is a simple program that takes the radius of the circle as input and computes its area:
def calculate_circle_area(radius):
pi = 3.14159
area = pi * (radius ** 2)
return area
radius = float(input("Enter the radius of the circle: "))
print("The area of the circle is:", calculate_circle_area(radius))
Let’s break down the program:
- We define a function
calculate_circle_area
that takes the radius as a parameter and returns the calculated area. - We prompt the user to enter the radius of the circle using the
input
function, and then convert the input to a float usingfloat()
. - We call the
calculate_circle_area
function with the given radius and print the result.
The Ultimate Shortcut: Using Python Libraries
While the above program works perfectly fine, Python offers a shortcut to calculate the area of a circle using the math
library. The math
library provides various mathematical functions, including the value of π. By using this library, we can simplify our code even further:
import math
radius = float(input("Enter the radius of the circle: "))
area = math.pi * (radius ** 2)
print("The area of the circle is:", area)
By using the math.pi
constant, we can directly access the value of π without defining IT ourselves. This makes our code more concise and easier to read, demonstrating the power of Python libraries in simplifying complex tasks.
Conclusion
Mastering the area of circle program in Python can be achieved by understanding the basic formula for calculating the area of a circle and leveraging the power of Python libraries. By embracing the ultimate shortcut of using the math
library, we can make our code more efficient and effective, demonstrating the elegance and simplicity of Python programming. Whether you are a beginner or an experienced Python programmer, this knowledge will undoubtedly elevate your coding skills to the next level.
FAQs
Q: Can I use a negative radius in the program?
A: The formula for calculating the area of a circle does not restrict the use of negative numbers for the radius. However, in practical scenarios, a negative radius does not make sense geometrically, so IT‘s best to handle input validation to prevent such cases.
Q: Can I use a different value for π?
A: While the standard value of π is approximately 3.14159, you can use a more precise value if needed. The math
library provides a more accurate value of π using math.pi
.
Q: Can I calculate the area of a circle with a diameter instead of a radius?
A: Yes, you can easily modify the program to accept the diameter instead of the radius. Simply divide the diameter by 2 to get the radius before calculating the area.
Q: Are there other ways to calculate the area of a circle in Python?
A: Yes, there are various ways to calculate the area of a circle in Python, including using different mathematical formulas or algorithms. However, the basic formula Area = π * r2
remains the most common and straightforward method.