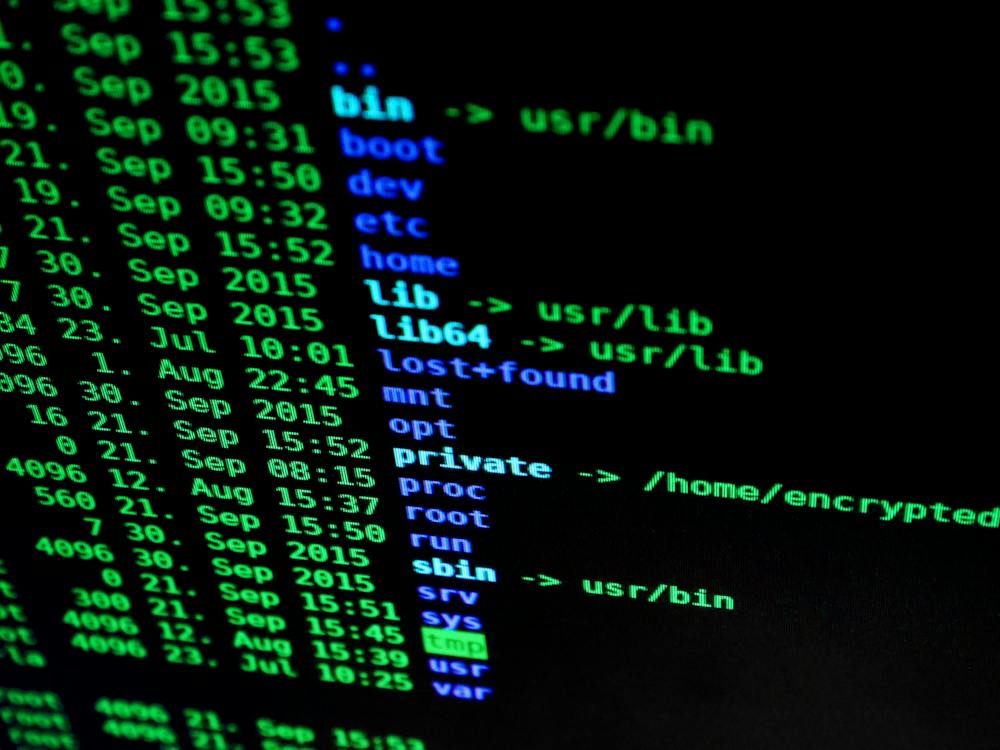
JavaScript is one of the most popular programming languages in the world. IT is used to create dynamic and interactive websites, web applications, and mobile apps. Many developers are familiar with the basics of JavaScript, but there are still many secrets and advanced features of the language that are not well-known.
In this article, we will uncover some of the lesser-known aspects of JavaScript and show you how to take your coding skills to the next level. Whether you’re a beginner or an experienced developer, there’s always something new to learn about this versatile language.
1. Closures and Scope
One of the most powerful and intriguing features of JavaScript is its support for closures. A closure is a way of creating a private scope within a function, allowing you to encapsulate variables and methods that are not accessible from outside the function. This can be extremely useful for creating modular and maintainable code.
Here’s a simple example of a closure in JavaScript:
“`javascript
function createCounter() {
let count = 0;
return function() {
return ++count;
};
}
let counter = createCounter();
console.log(counter()); // 1
console.log(counter()); // 2
console.log(counter()); // 3
“`
In this example, the createCounter
function returns an inner function that increments the count
variable. The count
variable is hidden from the outside world, but the inner function still has access to it thanks to closure.
2. Prototypal Inheritance
JavaScript uses a prototypal inheritance model, which is different from the classical inheritance model used in many other programming languages. In JavaScript, each object has a prototype property that points to another object. This allows objects to inherit properties and methods from their prototypes, creating a chain of objects.
Here’s an example of prototypal inheritance in JavaScript:
“`javascript
function Animal(name) {
this.name = name;
}
Animal.prototype.sayName = function() {
console.log(this.name);
};
function Dog(name, breed) {
Animal.call(this, name);
this.breed = breed;
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.bark = function() {
console.log(‘Woof!’);
};
let myDog = new Dog(‘Buddy’, ‘Golden Retriever’);
myDog.sayName(); // “Buddy”
myDog.bark(); // “Woof!”
“`
In this example, the Dog
object inherits the sayName
method from the Animal
prototype. By understanding the prototypal inheritance model, you can create powerful and flexible object-oriented code in JavaScript.
3. Event Loop and Asynchronous Programming
JavaScript is famously known for its asynchronous programming model, which is made possible by the event loop. Unlike many other programming languages, JavaScript runs in a single-threaded environment, meaning that it can only execute one piece of code at a time.
However, JavaScript can still handle asynchronous tasks such as fetching data from a server or responding to user input without getting blocked. This is achieved using the event loop, which manages the execution of asynchronous tasks and callbacks.
Here’s a simple example of asynchronous programming in JavaScript using setTimeout
:
“`javascript
console.log(‘Start’);
setTimeout(() => {
console.log(‘Timeout’);
}, 0);
console.log(‘End’);
“`
When you run this code, you might expect the output to be “Start”, “End”, “Timeout”. However, due to the asynchronous nature of setTimeout
, the actual output will be “Start”, “End”, “Timeout”. This demonstrates how JavaScript can handle asynchronous tasks without blocking the main thread of execution.
4. Modern JavaScript Features
JavaScript is constantly evolving, and new features and syntax enhancements are added to the language on a regular basis. Many of these features are designed to improve the readability, maintainability, and performance of JavaScript code.
Some of the modern JavaScript features that you might not be aware of include:
- Arrow functions
- Template literals
- Destructuring assignment
- Async/await syntax
- Modules
- And many more
By familiarizing yourself with these modern JavaScript features, you can write cleaner, more concise, and more efficient code.
5. Best Practices and Tips
Finally, it’s important to be aware of some best practices and tips for writing high-quality JavaScript code. These include:
- Using descriptive variable and function names
- Following a consistent coding style
- Using code comments to explain complex logic
- Avoiding global variables
- Handling errors and exceptions gracefully
Following these best practices and tips can help you write code that is more maintainable, easier to understand, and less prone to bugs and errors.
Conclusion
JavaScript is a powerful and versatile programming language that offers a wide range of features and capabilities. By uncovering the lesser-known aspects of JavaScript, you can become a more proficient and effective developer. Whether you’re working on web development, mobile app development, or server-side scripting, JavaScript has a lot to offer.
By understanding closures, prototypal inheritance, asynchronous programming, modern JavaScript features, and best practices, you can elevate your coding skills and create better, more efficient code. Keep learning and exploring the secrets of JavaScript, and you’ll be amazed at what you can achieve.
FAQs
1. What is JavaScript?
JavaScript is a programming language that is primarily used for creating interactive and dynamic content on websites. It is often used in conjunction with HTML and CSS to build rich user interfaces and web applications.
2. Is JavaScript the same as Java?
No, JavaScript is not the same as Java. While they share a similar name, they are completely different programming languages with different syntax, features, and use cases.
3. What are some common use cases for JavaScript?
JavaScript is commonly used for tasks such as form validation, interactive media elements, dynamic content updates, and asynchronous data fetching. It is also used for building web applications, mobile apps, and server-side scripting.