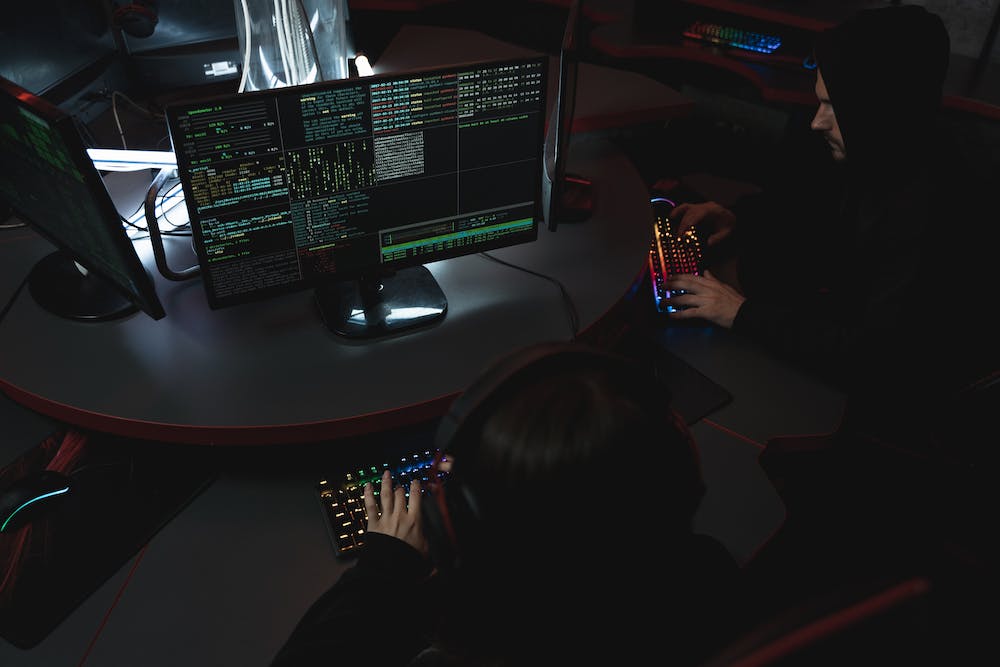
JavaScript is one of the most popular programming languages in the world, and for good reason. IT allows developers to create dynamic and interactive websites, making it an essential tool for web development. One of the key features of JavaScript is its data types, which are crucial for understanding how the language functions. In this article, we’ll take a deep dive into the world of JavaScript data types, uncovering the secrets that will help you become a more skilled and knowledgeable developer.
Understanding Data Types
JavaScript has several different data types, each with its own unique characteristics and uses. The main data types in JavaScript are:
- Number: Represents numeric values, both integers and floating-point numbers.
- String: Represents textual data, such as words and characters.
- Boolean: Represents a logical value, either true or false.
- Undefined: Represents a variable that has been declared but not assigned a value.
- Null: Represents a variable with an empty value.
- Object: Represents a collection of key-value pairs, making it a versatile and powerful data type.
- Array: Represents a special kind of object that stores a list of values.
Working with Data Types
Understanding the different data types in JavaScript is essential for writing effective and efficient code. For example, when performing mathematical calculations, you need to ensure that you are working with numbers and not strings. Similarly, when working with arrays, you need to understand how to access and manipulate the elements within them.
Let’s take a closer look at some common operations involving data types in JavaScript:
Converting Data Types
JavaScript allows you to convert data from one type to another using built-in functions. For example, you can use the parseInt()
function to convert a string to an integer, or the toString()
function to convert a number to a string. Understanding how to perform these conversions is essential for working with different data types in JavaScript.
Checking Data Types
JavaScript provides a typeof
operator that allows you to check the data type of a variable. This can be useful for debugging and ensuring that your code is working as expected. For example, if you want to ensure that a variable is a number before performing mathematical operations on it, you can use the typeof
operator to verify its data type.
Working with Objects and Arrays
Objects and arrays are two of the most powerful data types in JavaScript, allowing you to store and manipulate complex data structures. Understanding how to create, access, and modify objects and arrays is essential for building sophisticated applications. For example, you can use dot notation to access properties of an object, or use array methods like push()
and pop()
to add and remove elements from an array.
Best Practices for Working with Data Types
Now that we’ve uncovered the secrets of JavaScript data types, it’s important to consider some best practices for working with them. By following these guidelines, you can ensure that your code is efficient, bug-free, and easy to maintain.
Use Strict Equality
When comparing values in JavaScript, it’s best to use the strict equality operator (===
) rather than the loose equality operator (==
). The strict equality operator checks both the value and the data type of the operands, whereas the loose equality operator only checks the value. This can help prevent unexpected behavior and bugs in your code.
Handle Type Coercion Carefully
JavaScript has a feature called type coercion, which can lead to unexpected results if not handled carefully. Type coercion is the process of converting one data type to another, often implicitly. For example, when using the +
operator to concatenate strings, JavaScript will automatically convert other data types to strings. Understanding how type coercion works and when it occurs is essential for writing reliable and predictable code.
Use Descriptive Variable Names
When working with different data types, it’s important to use descriptive variable names that reflect the purpose and content of the data. This can make your code more readable and maintainable, as it will be easier to understand the role of each variable in your code.
Conclusion
JavaScript data types are fundamental to understanding how the language works and how to write effective and efficient code. By mastering the different data types and following best practices for working with them, you can become a more skilled and knowledgeable developer. Whether you’re building simple web pages or complex web applications, a solid understanding of JavaScript data types will be invaluable in your journey as a developer.
FAQs
Q: How many data types are there in JavaScript?
A: JavaScript has seven primary data types: Number, String, Boolean, Undefined, Null, Object, and Array.
Q: How do I check the data type of a variable in JavaScript?
A: You can use the typeof
operator to check the data type of a variable. For example, typeof 42
will return “number”.
Q: What is the strict equality operator in JavaScript?
A: The strict equality operator (===
) checks both the value and the data type of the operands, whereas the loose equality operator (==
) only checks the value.