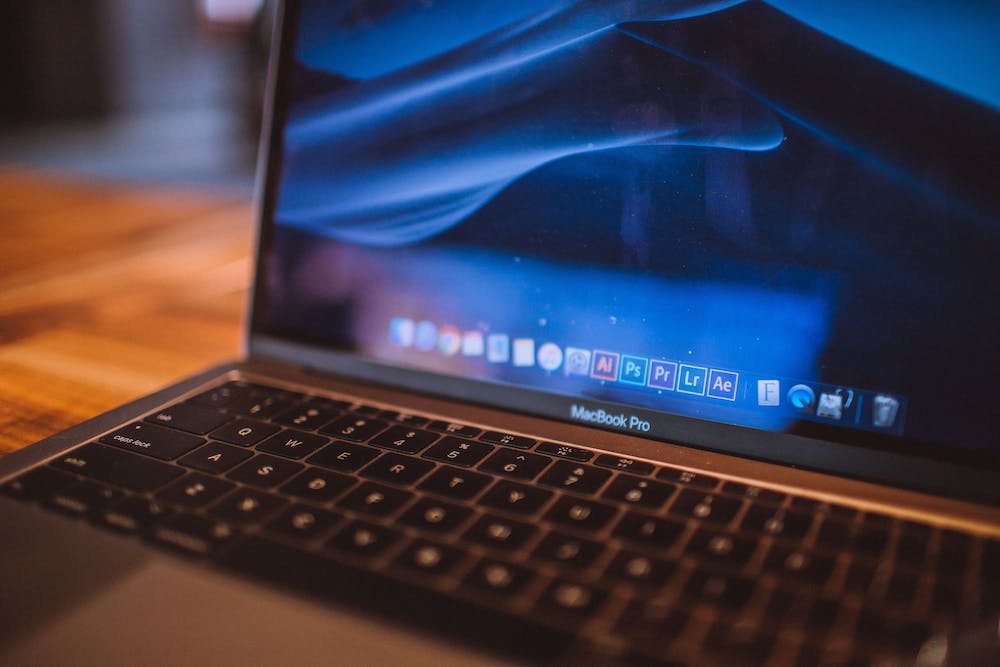
Binary search is a powerful algorithm used to efficiently search for a specific element in a sorted list. IT follows a divide and conquer approach, which allows IT to locate the target element with a time complexity of O(log n). In this article, we will explore the intricacies of binary search and unveil a Python code implementation that will help you perfect this searching technique.
Understanding Binary Search
Binary search operates on the principle of repeatedly dividing the search space in half until the target element is found. The algorithm starts by comparing the target element with the middle element of the sorted list. If the target is smaller, the search continues on the lower half of the list. Conversely, if the target is larger, the search proceeds on the upper half. This process is repeated iteratively until the element is located or the search space becomes empty.
Let’s take a look at the Python code for binary search:
def binary_search(arr, target):
low = 0
high = len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
# Example usage:
arr = [2, 5, 8, 12, 16, 23, 38, 56, 72, 91]
target = 16
result = binary_search(arr, target)
if result != -1:
print(f"Element found at index {result}")
else:
print("Element not found")
In the code above, we define a function called binary_search
that takes an array arr
and a target element target
. We initialize two variables, low
and high
, to represent the lower and upper bounds of the search space respectively. The algorithm enters a while loop that continues until the search space is exhausted.
Inside the loop, we calculate the midpoint of the search space using the formula mid = (low + high) // 2
. We then compare the value of the element at the midpoint with the target element. If they are equal, we have found the element and return the midpoint index. If the element at the midpoint is smaller than the target, we update the low
variable to move the search space to the upper half. Otherwise, if the element at the midpoint is larger, we update the high
variable to move the search space to the lower half.
If the while loop terminates without finding the target element, we return -1 to indicate that the element is not present in the array.
Advantages of Binary Search
Binary search offers several advantages over other search algorithms, making IT widely used in various applications:
- Efficiency: Binary search has a time complexity of O(log n), which is significantly faster than linear search algorithms with a time complexity of O(n).
- Applicability: Binary search can be applied to any sorted list, making IT versatile and usable in various scenarios.
- Optimality: Binary search guarantees finding the target element in the fewest number of comparisons, making IT an optimal search algorithm.
Conclusion
Binary search is a powerful algorithm that allows for efficient searching in a sorted list. Its divide and conquer approach and time complexity of O(log n) make IT an ideal choice in many scenarios. By implementing the Python code provided in this article, you can unlock the secrets to perfecting your binary search skills and enhance your problem-solving abilities.
FAQs
1. Is binary search applicable to unsorted lists?
No, binary search can only be applied to sorted lists. IT relies on the assumption that the list is sorted in ascending or descending order for its effectiveness.
2. What happens if the target element is not present in the array?
If the target element is not found in the array, the binary search algorithm will return -1 to indicate that the element is not present.
3. Why is binary search preferred over linear search?
Binary search is preferred over linear search because IT has a significantly faster time complexity of O(log n) compared to linear search’s O(n). This efficiency makes binary search ideal for large datasets.
4. Can binary search be used in languages other than Python?
Absolutely! Binary search is a general algorithm and can be implemented in various programming languages, including but not limited to C++, Java, and JavaScript.