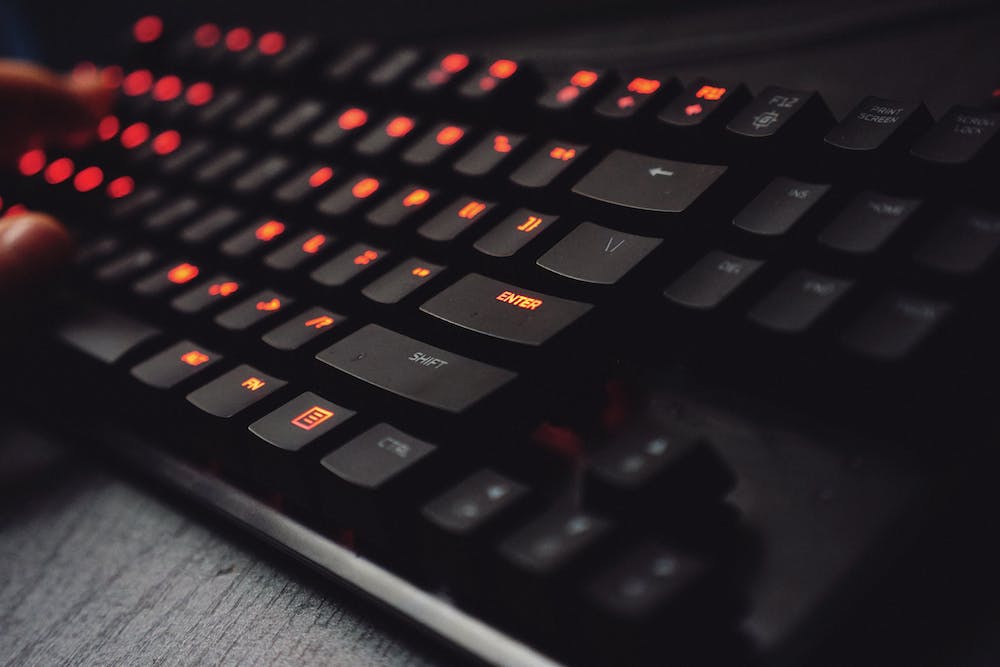
Data clustering is a fundamental concept in data analysis and machine learning. IT involves grouping similar data points together to uncover patterns and relationships within the data. One of the most popular clustering algorithms is K-Means, which is known for its simplicity and efficiency. In this tutorial, we will dive into the world of K-Means clustering and learn how to implement it in Python. By the end of this tutorial, you will have the skills to efficiently cluster your own data using the K-Means algorithm.
What is K-Means Clustering?
K-Means clustering is a method of vector quantization, originally from signal processing, that is popular for cluster analysis in data mining. It aims to partition n observations into k clusters in which each observation belongs to the cluster with the nearest mean, serving as a prototype of the cluster. To achieve this, K-Means iteratively assigns each data point to the nearest cluster center and then recalculates the cluster centers based on the assigned data points. This process continues until the cluster centers stabilize, or a specified number of iterations is reached.
Implementing K-Means Clustering in Python
Now, let’s take a deep dive into implementing the K-Means algorithm in Python. We will be using the popular machine learning library, scikit-learn, for this purpose. If you don’t have scikit-learn installed, you can do so using pip:
pip install scikit-learn
Once you have scikit-learn installed, we can start by importing the necessary libraries in Python:
import numpy as np
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
Next, let’s generate some sample data to work with:
X = np.array([[1, 2], [5, 8], [1.5, 1.8], [8, 8], [1, 0.6], [9, 11]])
Now, we can initialize the K-Means algorithm with the desired number of clusters and fit it to our data:
kmeans = KMeans(n_clusters=2)
kmeans.fit(X)
Once the algorithm has been fitted to the data, we can retrieve the cluster centers and the labels for each data point:
centroids = kmeans.cluster_centers_
labels = kmeans.labels_
Finally, we can visualize the clusters using a scatter plot:
plt.scatter(X[:,0], X[:,1], c=labels, cmap='viridis')
plt.scatter(centroids[:,0], centroids[:,1], c='red', marker='x')
plt.show()
Conclusion
Congratulations! You now have a solid understanding of how to implement the K-Means clustering algorithm in Python using scikit-learn. By mastering this powerful tool, you can efficiently cluster your own data and uncover valuable insights that can drive informed decision-making. Whether you are working with customer segmentation, anomaly detection, or any other application of clustering, K-Means can be a game-changer in your data analysis arsenal. So, go ahead and apply what you’ve learned to your own projects and unlock the potential of your data!
FAQs
What is the optimal number of clusters to use in K-Means?
Deciding the optimal number of clusters, also known as the “elbow method”, is a common challenge when using K-Means. One approach is to plot the within-cluster sum of squares (WCSS) for different numbers of clusters and look for the “elbow” point where the rate of decrease sharply changes. This point is often a good indication of the optimal number of clusters to use.
Can K-Means handle non-linear clusters?
No, K-Means assumes that the clusters are spherically shaped and of equal size. If your data contains non-linear clusters, you may need to explore other clustering algorithms such as DBSCAN or spectral clustering.