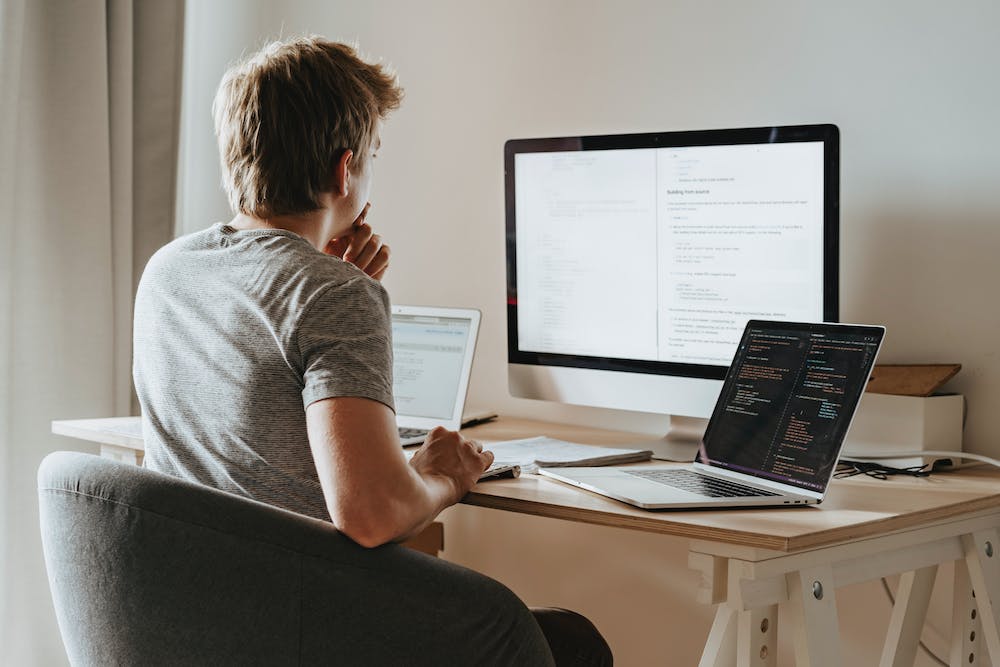
Python is a versatile programming language that has gained immense popularity in recent years. IT is known for its simplicity, readability, and versatility, which make it an ideal language for both beginner and experienced programmers. In this article, we will uncover some of the secret snake codes of Python and reveal some tips and tricks that can help enhance your Python programming skills.
Python Programming Tips and Tricks
Python has a plethora of features and functionalities that can make programming more efficient and enjoyable. Here are some tips and tricks to unlock the full potential of Python:
1. Use List Comprehension
List comprehension is a powerful feature in Python that allows you to create lists in a more concise and readable manner. Instead of using traditional for loops to create lists, you can use list comprehension to achieve the same result in a single line of code. Here’s an example:
“`python
# Traditional for loop
result = []
for i in range(10):
result.append(i * 2)
# List comprehension
result = [i * 2 for i in range(10)]
“`
2. Leverage Built-in Functions and Modules
Python comes with a wide range of built-in functions and modules that can help simplify your code and make it more efficient. For example, the ‘os’ module provides functionalities for interacting with the operating system, while the ‘math’ module offers mathematical functions for complex calculations.
3. Use Virtual Environments
Virtual environments are a great way to manage dependencies and isolate projects in Python. By creating separate virtual environments for each project, you can ensure that the dependencies are not conflicting with each other, leading to a more stable and manageable codebase.
4. Explore Python Libraries
Python has a vast ecosystem of third-party libraries that can help you accomplish various tasks without reinventing the wheel. For example, the ‘requests’ library is great for making HTTP requests, while the ‘pandas’ library is perfect for data manipulation and analysis.
Optimizing Python Code for Performance
In addition to the tips and tricks mentioned above, it’s essential to optimize your Python code for performance, especially when working on large-scale projects. Here are some strategies to consider:
1. Profile Your Code
Profiling your code can help identify bottlenecks and performance issues. Python comes with built-in profiling tools, such as cProfile and timeit, which can help analyze the performance of your code and identify areas for improvement.
2. Use Generators
Generators are a memory-efficient way to iterate over large datasets. Instead of creating and storing the entire dataset in memory, generators yield one item at a time, reducing memory consumption and improving performance.
3. Employ Multithreading and Multiprocessing
Python’s threading and multiprocessing modules allow you to run multiple tasks concurrently, improving the performance of your code, especially when working with CPU-bound or I/O-bound tasks.
4. Utilize C Extensions
If performance is critical for your project, consider writing critical parts of your code in C or C++ and using Python’s C API to integrate them with your Python codebase. This can significantly improve the performance of your application.
Conclusion
Python is a powerful and versatile programming language that offers endless possibilities for developers. By leveraging the tips and tricks mentioned in this article and optimizing your code for performance, you can unlock the full potential of Python and take your programming skills to the next level.
FAQs
Q: How can I improve my Python programming skills?
A: To improve your Python programming skills, it’s essential to practice regularly, explore Python libraries and modules, and stay updated with the latest trends and best practices in the Python community. Additionally, you can consider enrolling in online courses or attending workshops to enhance your skills.
Q: What are some common pitfalls to avoid in Python programming?
A: Some common pitfalls to avoid in Python programming include inefficient coding practices, not leveraging built-in functions and modules, and neglecting code optimization for performance. It’s important to write clean, readable, and efficient code to avoid potential pitfalls in Python programming.