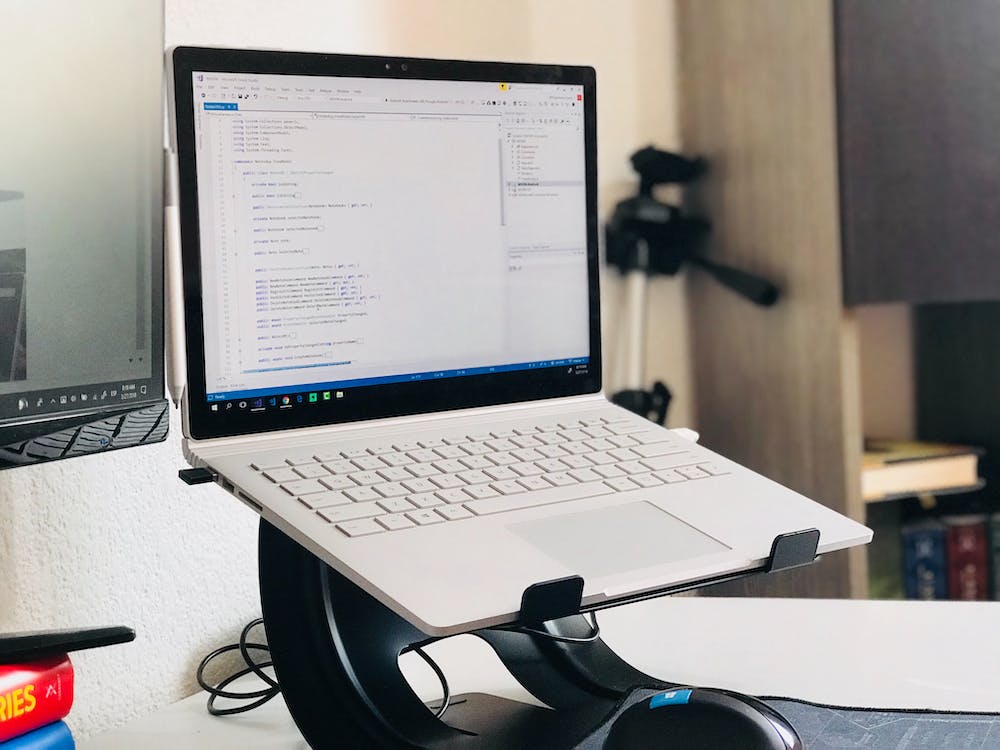
PHP is a powerful programming language that is widely used for web development. One of the key features of PHP is its ability to handle global variables, which can be incredibly useful for managing data across different parts of a program. In this article, we will uncover the secret powers of PHP global variables that you may not have known about.
Understanding Global Variables
In PHP, global variables are variables that can be accessed from anywhere in a program. This means that they are not limited to a specific function or class, and can be used to store and manipulate data across different parts of a program. Global variables are declared using the global keyword, which makes them accessible from any part of the program.
The Secret Powers of PHP Global Variables
While global variables may seem straightforward, they actually have a number of powerful features that you may not have been aware of. Here are some of the hidden powers of PHP global variables:
1. Accessing Global Variables from Functions
One of the most useful features of global variables is their ability to be accessed from within functions. This means that you can use global variables to pass data to functions without needing to explicitly pass them as parameters. For example:
“`php
$name = “John”;
function greet() {
global $name;
echo “Hello, ” . $name;
}
greet(); // Output: Hello, John
“`
2. Using Global Variables Across Files
Another powerful feature of global variables is their ability to be used across different files. This means that you can declare a global variable in one file, and then access IT from another file without needing to pass it as a parameter. For example:
File 1 (file1.php):
“`php
$count = 10;
“`
File 2 (file2.php):
“`php
include ‘file1.php’;
echo $count; // Output: 10
“`
3. Avoiding Variable Clashes
Global variables can also be used to avoid clashes between variable names. In larger programs, it is common to have multiple variables with the same name, which can lead to conflicts and errors. By using global variables, you can ensure that each variable is unique and does not clash with others.
Best Practices for Using PHP Global Variables
While PHP global variables can be incredibly useful, it is important to use them carefully to avoid potential issues. Here are some best practices for using PHP global variables:
- Avoid overusing global variables, as it can lead to a lack of clarity and maintainability in your code.
- Use global variables sparingly, and only when it is absolutely necessary to share data across different parts of a program.
- Consider using alternatives such as passing variables as function parameters or using classes and objects to manage data.
Conclusion
In conclusion, PHP global variables have a number of secret powers that make them incredibly useful for managing data across different parts of a program. By understanding and leveraging these powers, you can write more efficient and maintainable code in PHP.
FAQs
Q: Can global variables be accessed from within classes?
A: Yes, global variables can be accessed from within classes by using the global keyword to declare them.
Q: Are global variables always the best solution for sharing data across different parts of a program?
A: No, global variables should be used judiciously and only when it is necessary to share data across different parts of a program. In many cases, passing variables as function parameters or using objects and classes may be a better alternative.