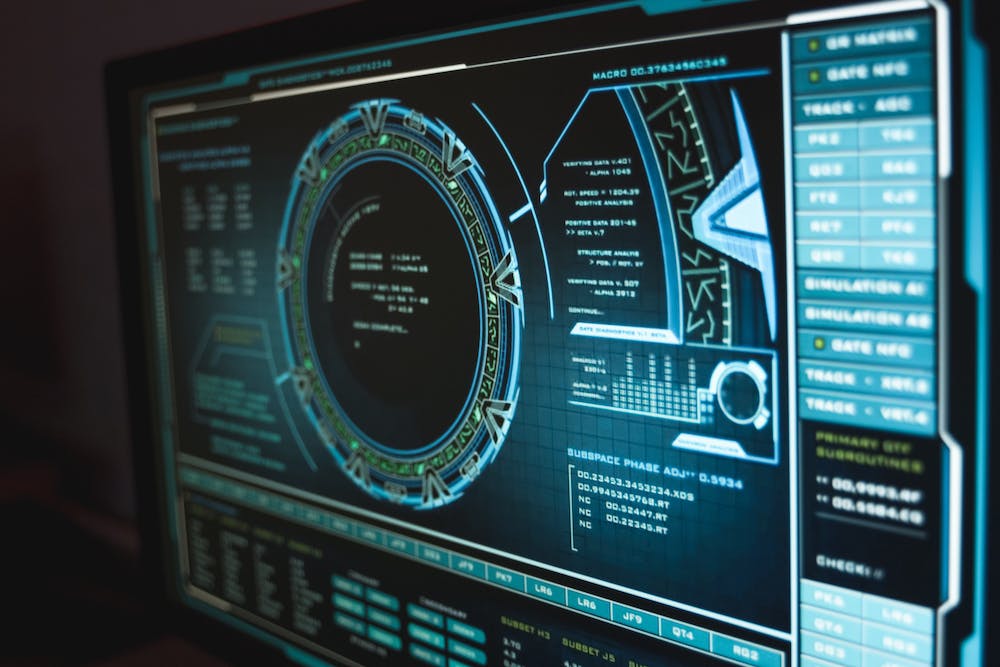
Python is a powerful and versatile programming language that is used by developers across the globe. IT has gained popularity due to its simplicity, readability, and the ability to support multiple programming paradigms. However, many Python programmers are unaware of the inner workings of the language, particularly when it comes to Python bytecode.
What is Python Bytecode?
Python bytecode is the low-level representation of a Python program that is used by the Python interpreter. When you write a Python program, the source code is first compiled into bytecode, which is then executed by the Python Virtual Machine (PVM). This compilation process is what allows Python to be a dynamically typed language without sacrificing performance.
How is Python Bytecode Generated?
When you run a Python script, the Python interpreter first parses the source code and creates an Abstract Syntax Tree (AST). This AST is then compiled into bytecode using the built-in compiler module. The resulting bytecode is then executed by the PVM, which is responsible for translating the bytecode into machine code that can be executed by the underlying hardware.
Understanding Python Bytecode
Python bytecode is a stack-based virtual machine language, which means that it uses a stack to hold intermediate values during the execution of a program. Each bytecode instruction operates on the stack, manipulating the values stored within it. For example, the LOAD_CONST
instruction pushes a constant value onto the stack, while the BINARY_ADD
instruction pops two values from the stack, adds them together, and pushes the result back onto the stack.
Let’s take a look at a simple Python function and its corresponding bytecode:
def add(a, b):
return a + b
2 0 LOAD_FAST 0 (a)
2 LOAD_FAST 1 (b)
4 BINARY_ADD
6 RETURN_VALUE
In this example, the bytecode for the add
function consists of four instructions. The LOAD_FAST
instructions load the values of a
and b
onto the stack, the BINARY_ADD
instruction adds the two values together, and the RETURN_VALUE
instruction returns the result to the caller.
Why Should You Care About Python Bytecode?
Understanding Python bytecode can be incredibly useful for Python programmers, even if they don’t plan on delving into the inner workings of the language. Here are a few reasons why you should care about Python bytecode:
- Performance Optimization: By understanding how Python bytecode is executed, you can write more efficient code that takes advantage of the underlying bytecode instructions.
- Debugging and Profiling: Knowledge of Python bytecode can help you diagnose performance issues and optimize your code by understanding how the interpreter executes your program.
- Security Analysis: Python bytecode can be disassembled and analyzed to uncover potential security vulnerabilities in your code.
Disassembling Python Bytecode
In order to view the bytecode for a Python function or module, you can use the built-in dis
module. For example, to disassemble the bytecode for the add
function from earlier, you can do the following:
import dis
def add(a, b):
return a + b
dis.dis(add)
When you run this code, you’ll see the disassembled bytecode for the add
function, which will give you a better understanding of how the Python interpreter executes the function’s code.
Common Misconceptions About Python Bytecode
There are several common misconceptions about Python bytecode that are worth addressing:
- Python bytecode is not machine code: While Python bytecode is executed by the PVM, it is not directly executed by the CPU. Instead, the PVM interprets the bytecode and translates it into machine code on the fly.
- Python bytecode is not platform-independent: The bytecode generated by the Python compiler is specific to the version of Python and the architecture of the system on which it was generated.
- Python bytecode is not obfuscated: It is possible to disassemble Python bytecode and view the underlying instructions, so it should not be considered a form of obfuscation or copy protection.
Conclusion
Python bytecode is an integral part of the Python programming language, and gaining a deeper understanding of how it works can help you write more efficient, secure, and maintainable code. By delving into the mysterious world of Python bytecode, you can unlock new insights into the inner workings of the language and become a more proficient Python programmer.
FAQs
Q: Does understanding Python bytecode make me a better programmer?
A: Understanding Python bytecode can certainly make you a more knowledgeable and versatile programmer. It can help you write more efficient code and troubleshoot performance issues more effectively.
Q: Can Python bytecode be optimized for better performance?
A: Yes, Python bytecode can be optimized by writing code that takes advantage of the underlying bytecode instructions. Additionally, you can use tools like the built-in dis
module to identify performance bottlenecks in your code.
Q: Can Python bytecode be decompiled or reverse-engineered?
A: Yes, Python bytecode can be disassembled and decompiled, allowing you to view the underlying instructions and logic of your Python programs. However, it’s important to note that decompiling bytecode should only be done for legitimate purposes, such as debugging or optimizing code.