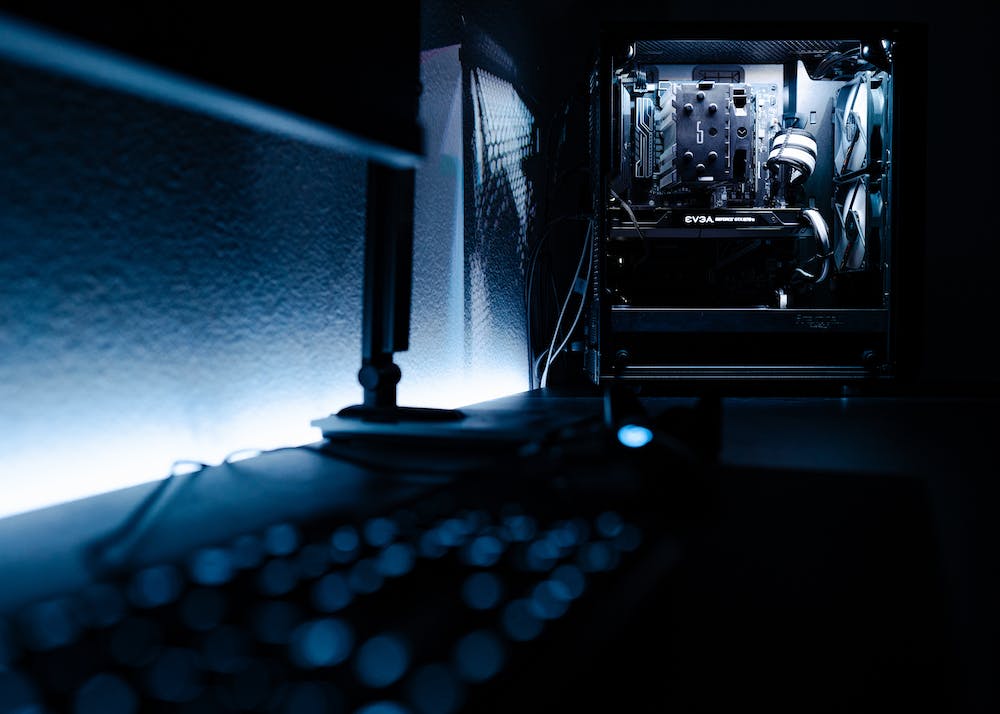
JavaScript is a widely used programming language that allows you to add interactivity and dynamic features to your webpages. IT has been around for decades and has become an integral part of web development. Most developers are familiar with the basic syntax and concepts of JavaScript, but there are hidden gems within the language that can truly level up your skills. One of these hidden gems is the ‘do’ statement.
The ‘do’ Statement
The ‘do’ statement in JavaScript is a lesser-known alternative to the more commonly used ‘while’ loop. While loops are used when you want to repeat a block of code as long as a specified condition is true. The ‘do’ statement, on the other hand, allows you to execute a block of code at least once, and then repeat IT while a specified condition is true. The syntax for the ‘do’ statement is as follows:
do {
// Code block to be executed
} while (condition);
Let’s say you want to calculate the sum of all numbers from 1 to 10 using a ‘do’ statement:
let sum = 0;
let i = 1;
do {
sum += i;
i++;
} while (i <= 10);
console.log(sum); // Output: 55
In this example, the code block inside the ‘do’ statement is executed first, and then the condition ‘i <= 10' is checked. If the condition is true, the code block is executed again, and this process continues until the condition becomes false.
The Power of the ‘do’ Statement
The ‘do’ statement can be particularly useful in situations where you want to ensure that a block of code is executed at least once, regardless of whether the specified condition is initially true or false. This can be handy when validating user input or performing certain actions based on user interactions.
Let’s take an example of a simple form validation using the ‘do’ statement:
let userInput;
do {
userInput = prompt("Please enter a number:");
} while (isNaN(userInput)); // Repeat until a number is entered
console.log("Valid number entered:", userInput);
In this example, the code prompts the user to enter a number and keeps repeating until a valid number is entered. The ‘isNaN()’ function is used to check if the user input is not a number. If the condition is true, the code block inside the ‘do’ statement is executed again, prompting the user to enter a number. Once a valid number is entered, the loop terminates, and the number is displayed in the console.
Common Use Cases
While the ‘do’ statement may not be commonly used in day-to-day coding, IT can be particularly useful in certain scenarios:
- Form validation: You can use the ‘do’ statement to ensure that user input meets certain criteria, such as being a valid email address or a strong password.
- Error handling: The ‘do’ statement can be used to repeatedly execute a block of code until a desired outcome is achieved, such as successfully connecting to a server or fetching data from an API.
- User interactions: When building interactive web applications, you can use the ‘do’ statement to continuously update the user interface based on user interactions, such as changing the appearance of a button when clicked multiple times.
Conclusion
The ‘do’ statement in JavaScript is a hidden gem that can enhance your coding skills and offer a different approach to solving problems. While IT may not be used extensively, IT is certainly worth exploring for specific use cases where executing a block of code at least once is required. By understanding the power of the ‘do’ statement, you can expand your arsenal of JavaScript techniques and become a more proficient web developer.
FAQs
Q: Are ‘do’ statements in JavaScript the same as ‘do-while’ loops?
A: Yes, the ‘do’ statement and ‘do-while’ loops are essentially the same thing. The term ‘do-while’ loop is often used to refer to loops that start with the ‘do’ keyword and end with the ‘while’ keyword, specifying the loop condition.
Q: Is the ‘do’ statement an alternative to ‘for’ and ‘while’ loops?
A: No, the ‘do’ statement is not an alternative to ‘for’ and ‘while’ loops but rather a variant of the ‘while’ loop. IT is used in scenarios where you want to ensure that a block of code is executed at least once before checking the loop condition.
Q: Can I nest a ‘do’ statement within another ‘do’ statement?
A: Yes, you can nest a ‘do’ statement within another ‘do’ statement or any other loop construct in JavaScript. This allows for further control and flexibility when dealing with complex looping scenarios.
Q: Are there any performance differences between the ‘do’ statement and ‘while’ loops?
A: In terms of performance, there is no significant difference between the ‘do’ statement and ‘while’ loops. Both constructs achieve similar results and can be used interchangeably based on the requirements of your code.