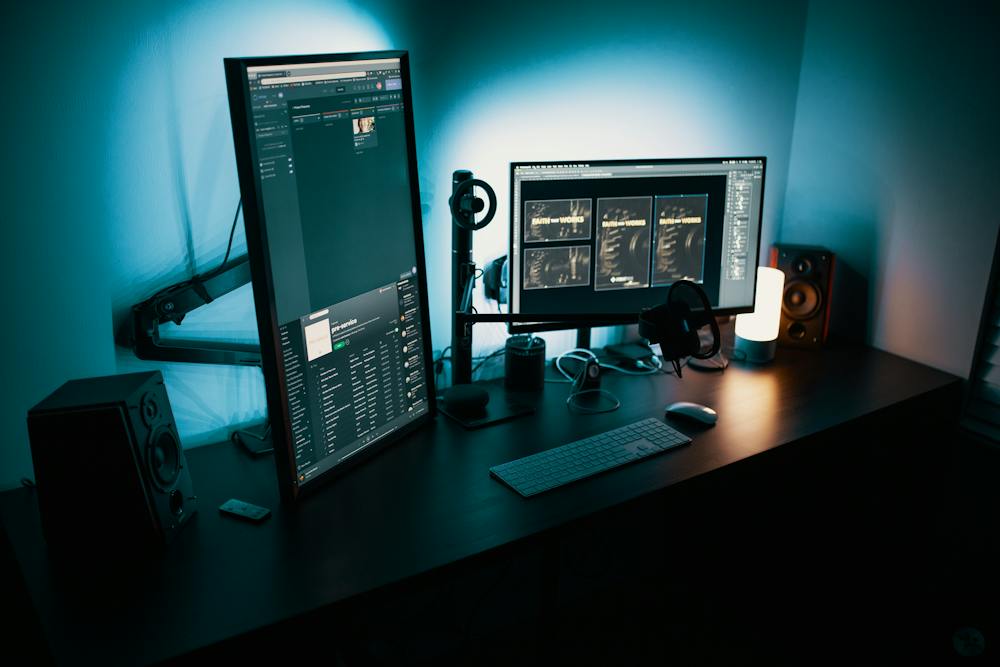
Python is a powerful and versatile programming language that is widely used for web development, data analysis, artificial intelligence, and more. One of the great things about Python is its simplicity and readability, making IT an ideal language for beginners to learn. In this article, we will uncover the magic of Python code by showing you how to build your own calculator GUI with just a few simple steps.
What is a GUI?
GUI stands for Graphical User Interface, and it refers to the visual elements of a program that allow users to interact with it. In the context of building a calculator in Python, a GUI will allow us to create a user-friendly interface with buttons for numbers, operators, and functions like clear and equals.
Step 1: Install Python
If you haven’t already, you will need to install Python on your computer. You can download the latest version of Python from the official Website, and follow the installation instructions to set it up on your machine. Once Python is installed, you can open a new file in your favorite text editor or Integrated Development Environment (IDE) to begin writing your calculator code.
Step 2: Import the Required Modules
Python has a rich library of modules that contain pre-written code for various tasks. For building a calculator GUI, we will need to import the tkinter module, which provides a toolkit for creating graphical interfaces in Python.
“`python
import tkinter as tk
“`
Step 3: Create the GUI Window
Next, we will create the main window for our calculator GUI using the Tk() constructor from the tkinter module. We can also set the title of the window using the title() method, and configure its size using the geometry() method.
“`python
root = tk.Tk()
root.title(“Calculator”)
root.geometry(“300×400”)
“`
Step 4: Create the Display
Now we will create a text box to display the input and output of the calculator. We can do this by creating a tk.StringVar() object to hold the text, and then associating it with a tk.Label widget using the textvariable attribute.
“`python
display_text = tk.StringVar()
display = tk.Label(root, textvariable=display_text, font=(“Arial”, 20), anchor=”e”)
display.pack(expand=True, fill=”both”)
“`
Step 5: Create the Buttons
With the display in place, we can now create the buttons for the numbers, operators, and functions of the calculator. We will create a 4×4 grid of buttons using the tk.Button widget, and assign each button a function to perform when clicked.
“`python
buttons = [
‘7’, ‘8’, ‘9’, ‘/’,
‘4’, ‘5’, ‘6’, ‘*’,
‘1’, ‘2’, ‘3’, ‘-‘,
‘0’, ‘.’, ‘C’, ‘+’
]
# create and position the buttons
for i in range(4):
for j in range(4):
button = tk.Button(root, text=buttons[i*4 + j], font=(“Arial”, 20), command=lambda x=buttons[i*4 + j]: on_button_click(x))
button.grid(row=i+1, column=j, sticky=”nsew”)
“`
Step 6: Define the Button Click Function
Finally, we need to define the on_button_click() function that will be called when a button is clicked. This function will update the display based on the button that was clicked, and perform the necessary calculations when the equals button is pressed.
“`python
def on_button_click(value):
if value == ‘C’:
display_text.set(”)
elif value == ‘=’:
try:
display_text.set(str(eval(display_text.get())))
except:
display_text.set(‘Error’)
else:
display_text.set(display_text.get() + value)
“`
Step 7: Run the Program
That’s it! You have now created a simple calculator GUI using Python’s tkinter module. You can save the code to a file with a .py extension, and run it using the command line or your favorite IDE. You should see a window pop up with the calculator interface, where you can click the buttons to perform calculations and see the results in the display.
Conclusion
Building a calculator GUI with Python is a great way to explore the capabilities of the language and learn about graphical user interfaces. With just a few simple steps, you can create a functional and user-friendly calculator that you can use and share with others. As you become more comfortable with Python and GUI programming, you can continue to enhance and customize the calculator to suit your needs and preferences.
FAQs
What is Python?
Python is a high-level programming language known for its readability and simplicity. It is widely used in web development, data analysis, artificial intelligence, and more.
What is tkinter?
tkinter is a built-in Python module that provides a toolkit for creating graphical user interfaces. It offers a variety of widgets and tools for building interactive applications with ease.
Can I customize the calculator GUI?
Yes, you can customize the appearance and functionality of the calculator GUI to suit your preferences. You can change the size, colors, fonts, and layout of the interface, as well as add new features and functionalities.
Is Python a good language for beginners?
Yes, Python is often recommended as a first programming language for beginners due to its simplicity, readability, and versatility. It has a gentle learning curve and a supportive community, making it an ideal choice for those new to programming.
Where can I learn more about Python programming?
There are many resources available for learning Python, including online tutorials, books, and courses. You can also join programming communities and forums to ask questions, share knowledge, and connect with other Python enthusiasts.