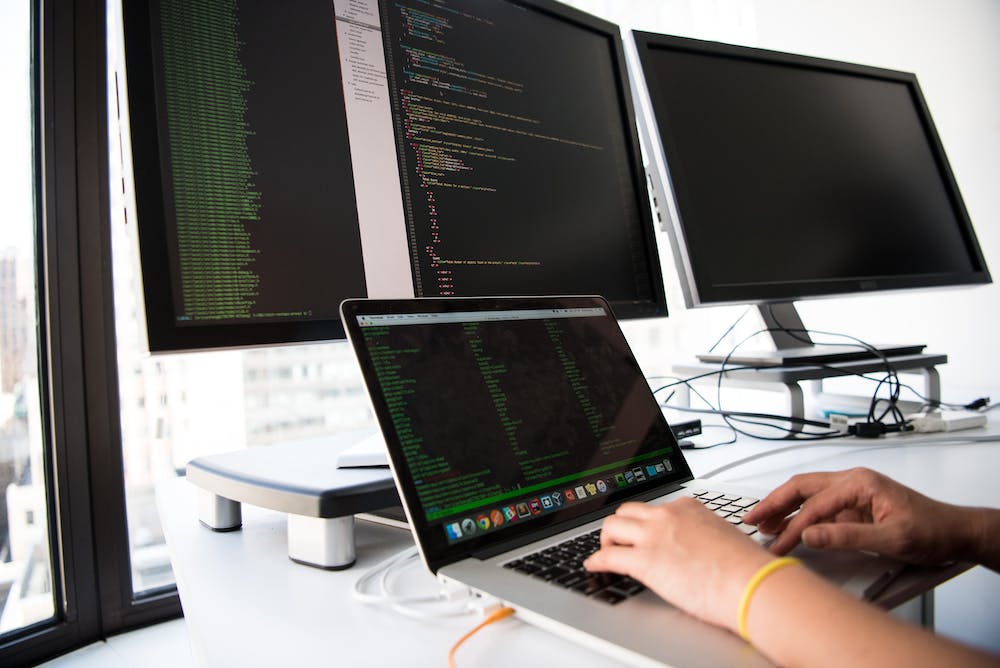
Introduction
K Means Clustering is a popular unsupervised machine learning algorithm used for data clustering and pattern recognition. IT aims to partition data points into K number of distinct clusters based on their similarity.
In this article, we will explore the concept of K Means Clustering and learn how to implement IT using Python code. We will begin by understanding the intuition behind the algorithm and its application areas. Then, we will dive into the implementation details, step-by-step, followed by a demonstration using a practical example dataset.
Understanding K Means Clustering
K Means Clustering is based on the concept of clustering, which is the process of dividing a set of data points into groups or clusters, such that objects within a cluster have high similarity, while objects from different clusters are dissimilar.
The algorithm works by iteratively assigning data points to their nearest centroid and updating the centroids based on the mean of the assigned points. This process continues until the centroids stabilize and the algorithm converges.
Application Areas of K Means Clustering
K Means Clustering has a wide range of applications across various domains. Some of the popular applications include:
- Customer segmentation in marketing
- Anomaly detection in network traffic
- Image segmentation
- Text clustering in Natural Language Processing
- Recommendation systems
These are just a few examples, and the algorithm can be applied to many other domains depending on the problem and available data.
Implementing K Means Clustering with Python
Now, let’s understand the step-by-step process of implementing K Means Clustering using Python code. We will be using the popular machine learning library, scikit-learn, for this purpose.
Step 1: Importing Libraries
The first step is to import the required libraries, including numpy, pandas, matplotlib, and sklearn.
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.cluster import KMeans
Step 2: Loading the Dataset
Next, we will load the dataset on which we want to apply K Means Clustering. We can use pandas to read the data from a CSV file or any other format, depending on the dataset’s type.
dataset = pd.read_csv("data.csv")
Step 3: Data Preprocessing
Before applying the clustering algorithm, IT‘s essential to preprocess the data. This step includes handling missing values, scaling features (if required), and converting categorical variables to numerical representations.
# Handle missing values
dataset = dataset.dropna()
# Scale features
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
scaled_data = scaler.fit_transform(dataset)
# Convert categorical variables
from sklearn.preprocessing import LabelEncoder
encoder = LabelEncoder()
dataset['Category'] = encoder.fit_transform(dataset['Category'])
Step 4: Finding the Optimal Number of Clusters (K)
K Means Clustering requires specifying the number of clusters, K, in advance. To determine the optimal value of K, we can use the elbow method, which plots the within-cluster sum of squares (WCSS) against different values of K and selects the K that causes a significant decrease in WCSS.
wcss = []
for i in range(1, 11):
kmeans = KMeans(n_clusters=i, init='k-means++', random_state=42)
kmeans.fit(scaled_data)
wcss.append(kmeans.inertia_)
plt.plot(range(1, 11), wcss)
plt.title('Elbow Method')
plt.xlabel('Number of Clusters')
plt.ylabel('WCSS')
plt.show()
Step 5: Applying K Means Clustering
Once we have determined the optimal value of K, we can apply the K Means Clustering algorithm.
kmeans = KMeans(n_clusters=3, init='k-means++', random_state=42)
kmeans.fit(scaled_data)
labels = kmeans.labels_
Step 6: Visualizing the Clusters
Finally, we can visualize the clusters using a scatter plot.
plt.scatter(scaled_data[:, 0], scaled_data[:, 1], c=labels, cmap='viridis')
plt.scatter(kmeans.cluster_centers_[:, 0], kmeans.cluster_centers_[:, 1], s=300, c='red')
plt.title('Clusters')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.show()
Conclusion
K Means Clustering is a powerful algorithm for uncovering hidden patterns in data through clustering. By partitioning data into clusters, IT helps in better understanding and analysis of complex datasets. In this article, we explored the concept of K Means Clustering, its application areas, and step-by-step implementation using Python code. By following the examples and guidelines, you can now apply this algorithm to your own datasets and gain valuable insights.
FAQs
Q: What is K Means Clustering?
K Means Clustering is an unsupervised machine learning algorithm that partitions data points into K number of distinct clusters based on their similarity.
Q: How is the optimal number of clusters determined in K Means Clustering?
The optimal number of clusters, K, can be determined using techniques such as the elbow method, silhouette analysis, or domain knowledge.
Q: What are some applications of K Means Clustering?
K Means Clustering has applications in customer segmentation, anomaly detection, image segmentation, text clustering, and recommendation systems, among others.
Q: Which library is used for implementing K Means Clustering in Python?
The scikit-learn library provides a KMeans class for implementing K Means Clustering in Python.
Q: Can K Means Clustering handle categorical variables?
No, K Means Clustering cannot handle categorical variables directly. Categorical variables need to be converted to numerical representations before applying the algorithm.