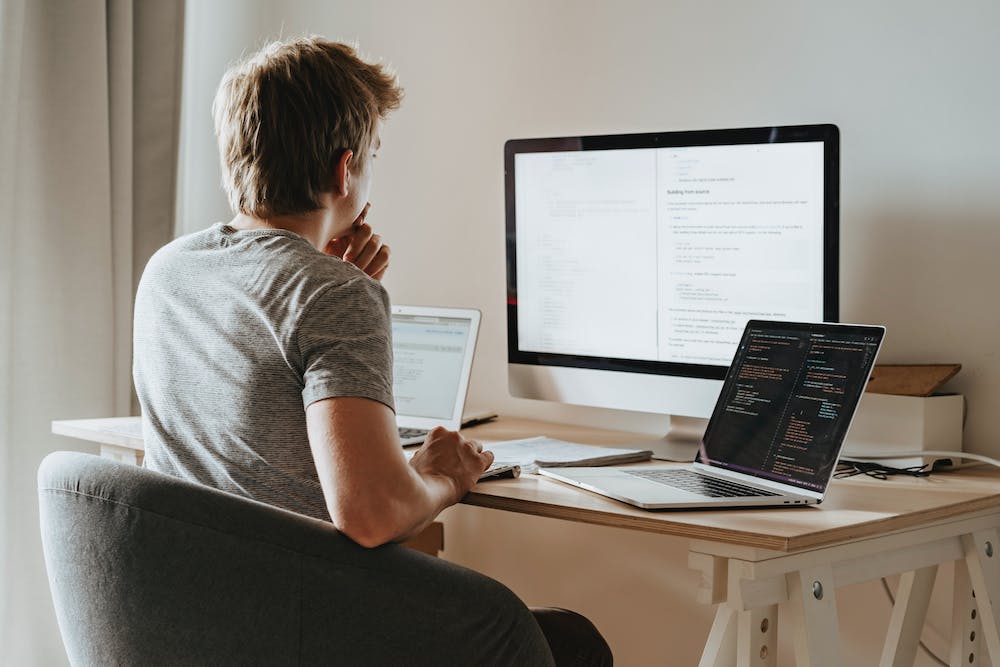
In Laravel, date and time manipulation is an essential part of many applications. Understanding and effectively utilizing the date functions provided by Laravel can greatly enhance your workflow and allow you to accomplish amazing things. In this article, we will explore some unbelievable tips and tricks for mastering Laravel’s date functions.
Tip 1: Carbon, Laravel’s Powerful Date Extension
Laravel utilizes Carbon, a powerful extension for handling date and time manipulation. Carbon extends PHP’s native DateTime class with additional useful methods, making IT ideal for dealing with dates and times in Laravel applications.
For example, you can easily perform calculations such as adding or subtracting hours, minutes, or seconds from a given date using Carbon’s convenient methods. Additionally, Carbon provides an intuitive interface to format dates based on your preferred localization settings.
Tip 2: Using the Carbon Instance
When working with Laravel’s date functions, IT‘s important to understand that most of these functions return a Carbon instance. This means you can directly chain Carbon’s methods to further manipulate or format your dates.
For instance, suppose you want to get the day of the week for a specific date. You can achieve this by chaining the `format()` method after calling the `->dayOfWeek()` function.
$date = Carbon::parse('2022-01-01')->dayOfWeek()->format('l');
This will return “Saturday” as output. By utilizing the power of Carbon’s methods, you can easily extract the desired information from a date and format IT according to your needs with ease.
Tip 3: Localization and Internationalization
In Laravel, handling dates in multiple languages becomes effortless. Laravel’s localization features allow you to quickly switch between different locales and display dates in the desired format.
You can set the application’s default locale in the `config/app.php` file by defining the `locale` option. Additionally, you can use Laravel’s localization feature to define language-specific date formats, making IT simple to display dates correctly for different regions.
'locale' => 'en', // Change this to your desired default language
Tip 4: Date Casting in Eloquent Models
Laravel allows you to cast attributes of Eloquent models to specific data types, including dates. This feature is particularly useful when interacting with database records that include date columns.
By defining the `date` cast on an attribute of an Eloquent model, Laravel will automatically convert IT into a Carbon instance when retrieving the record from the database. This saves time and effort by avoiding manual conversion.
protected $casts = [
'birthday' => 'date',
];
Tip 5: Advanced Date Filtering
Laravel offers a wide range of convenient methods to filter and query data based on date conditions. These methods are especially helpful when dealing with time-sensitive data or generating reports within a specific time frame.
For example, you can use the `whereDate()` method to filter records based on a specific date. This function will automatically compare the date portion of the attribute against the provided value.
$invoices = Invoice::whereDate('created_at', '2022-01-01')->get();
Conclusion
Mastering Laravel’s date functions opens up a world of possibilities in terms of date and time manipulation. By harnessing the power of Carbon and Laravel’s extensive features, you can effortlessly handle complex date calculations, display dates in various languages, and filter records based on specific time criteria.
Remember to explore the Laravel documentation for a comprehensive list of date functions and their capabilities.
FAQs Section
Q: How do I format a date in Laravel?
A: Laravel provides the `format()` method in Carbon to format dates according to your desired output. You can specify the format pattern using the `strftime` function format codes or by defining custom formats using characters like `Y`, `m`, `d`, `H`, `i`, `s`, etc. For example:
$date = Carbon::now()->format('Y-m-d H:i:s');
This will format the current date and time in the year-month-day hour:minute:second format.
Q: How do I add or subtract days from a specific date in Laravel?
A: Using Carbon’s `addDays()` and `subDays()` methods, you can easily add or subtract days from a given date. For instance:
$date = Carbon::now()->addDays(7); // Add 7 days to the current date
$date = Carbon::now()->subDays(3); // Subtract 3 days from the current date
These methods allow you to perform various date manipulations seamlessly.
Q: Can I compare dates in Laravel’s Eloquent queries?
A: Yes, Laravel provides several methods to compare dates in Eloquent queries. You can use methods like `whereDate()`, `whereMonth()`, `whereYear()`, and more to filter records based on specific date criteria. For example:
$users = User::whereDate('date_of_birth', '=', '2022-01-01')->get();
This will retrieve all users whose date of birth is January 1, 2022.
Q: How can I display dates in different languages using Laravel?
A: Laravel’s localization features allow you to easily switch between different languages and display dates according to the desired locale. You can set the application’s default locale and define language-specific date formats. Refer to Laravel’s documentation on localization to learn more about implementing multi-language support for your application.