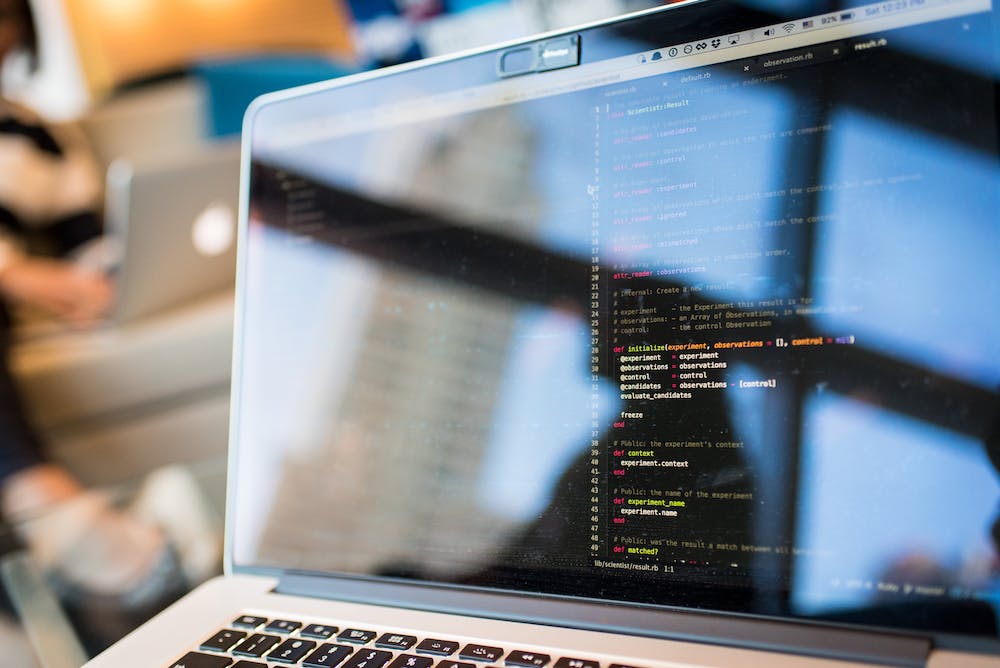
Python is a versatile and powerful programming language that is widely used in a variety of applications, including web development, data analysis, artificial intelligence, and more. One of the reasons for Python’s popularity is its readability and simplicity. However, Python is also known for its flexibility and expressiveness, allowing developers to create some truly mind-blowing source code examples. In this article, we’ll explore some unbelievable Python source code examples that will showcase the language’s power and versatility.
The Zen of Python
Before we dive into the source code examples, IT‘s important to understand the ethos of Python. The Zen of Python, a set of guiding principles for writing computer programs in Python, emphasizes readability, simplicity, and beauty. This philosophy is evident in the Python language itself, as well as in the source code examples we’re about to explore.
Example 1: Generating Fibonacci Sequence
The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1. In Python, we can generate the Fibonacci sequence using a simple recursive function:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
for i in range(10):
print(fibonacci(i))
This concise and elegant source code demonstrates Python’s ability to express complex mathematical concepts in a clear and intuitive manner. The use of recursion in this example is particularly impressive, showcasing Python’s support for functional programming techniques.
Example 2: Web Scraping with Beautiful Soup
Web scraping is the process of extracting data from websites, and Python is a popular choice for web scraping due to its rich set of libraries. Beautiful Soup is a Python library for pulling data out of HTML and XML files. The following source code example demonstrates how easy it is to use Beautiful Soup for web scraping:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
links = soup.find_all('a')
for link in links:
print(link.get('href'))
This source code showcases Python’s simplicity and expressiveness when it comes to web scraping. With just a few lines of code, we can retrieve all the hyperlinks from a web page, demonstrating Python’s versatility for data extraction tasks.
Example 3: Creating a REST API with Flask
Flask is a lightweight and easy-to-use web framework for Python, making it a great choice for building RESTful APIs. The following source code example demonstrates how to create a simple REST API using Flask:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/hello', methods=['GET'])
def get_hello():
return jsonify({'message': 'Hello, World!'})
if __name__ == '__main__':
app.run()
This source code exemplifies Python’s ability to create powerful web applications with minimal effort. The Flask framework allows us to define web routes and handle HTTP requests with ease, showcasing Python’s ability to handle complex networking tasks.
Example 4: Machine Learning with Scikit-Learn
Python is widely used in the field of machine learning, and Scikit-Learn is a popular library for building machine learning models. The following source code example showcases an implementation of a simple linear regression model using Scikit-Learn:
from sklearn.linear_model import LinearRegression
import numpy as np
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 6, 8, 10])
model = LinearRegression().fit(X, y)
print(model.coef_)
This source code demonstrates Python’s power for data analysis and machine learning. With just a few lines of code, we can create and train a machine learning model, showcasing Python’s strength in the field of artificial intelligence.
Conclusion
These unbelievable Python source code examples showcase the language’s power and versatility across a range of domains, from mathematics and web development to machine learning. Python’s readability, simplicity, and expressiveness make it a popular choice for developers looking to create elegant and efficient solutions to complex problems. Whether you’re a beginner or an experienced developer, Python’s rich set of libraries and frameworks make it a language worth mastering.
FAQs
1. What is Python?
Python is a high-level programming language known for its simplicity and readability. It is widely used in web development, data analysis, artificial intelligence, and more.
2. Why is Python popular?
Python is popular due to its readability, simplicity, versatility, and the abundance of libraries and frameworks available for various domains such as web development, data analytics, and machine learning.
3. What are some popular libraries and frameworks for Python?
Some popular libraries and frameworks for Python include NumPy, pandas, Beautiful Soup, Flask, Django, Scikit-Learn, and TensorFlow, among others.