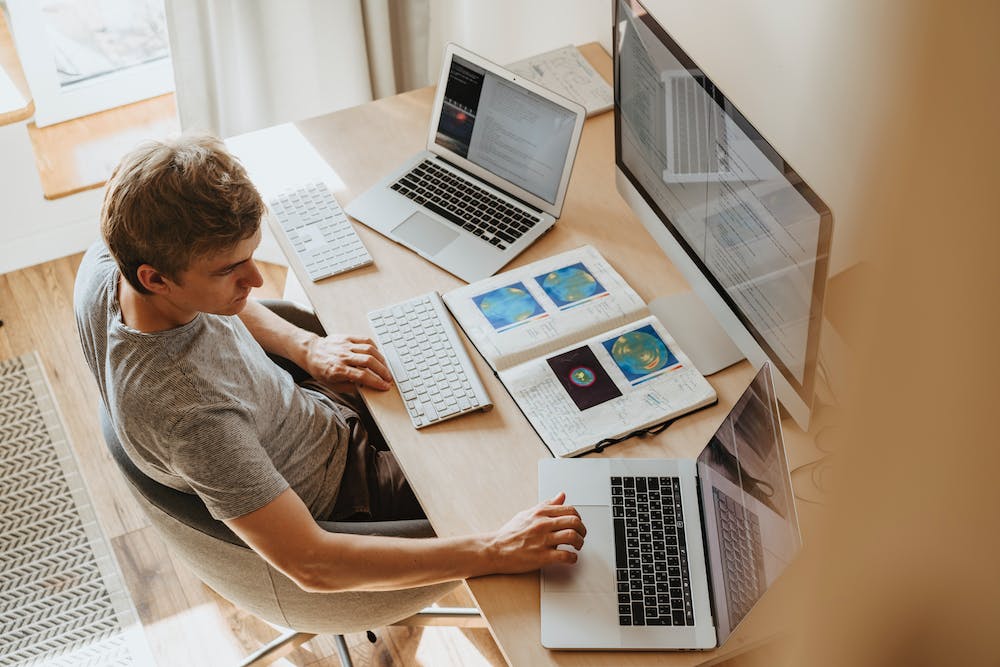
PHP is a powerful scripting language that is widely used for web development. One of the most commonly used functions in PHP is getDate(), which allows developers to work with dates and times in various ways. In this article, we will explore some incredible hacks and tricks that you can use with PHP getDate() to accomplish amazing things with dates and times.
1. Convert Date and Time Formats
One of the most common tasks when working with dates and times is converting them from one format to another. With getDate(), you can easily convert a date and time from one format to another. For example, you can convert a date from ‘YYYY-MM-DD’ format to ‘DD-MM-YYYY’ format using the following code:
$date = '2022-05-15';
$newDate = date('d-m-Y', strtotime($date));
echo $newDate; // Output: 15-05-2022
2. Calculate Age from Date of Birth
Another common use case for getDate() is calculating a person’s age from their date of birth. This can be achieved by subtracting the birth date from the current date and then extracting the years. Here’s a simple example:
$dob = '1990-10-05';
$age = date_diff(date_create($dob), date_create('now'))->y;
echo $age; // Output: 32
3. Find the Day of the Week
Using getDate(), you can easily find out which day of the week a particular date falls on. This can be useful for various applications such as scheduling, event planning, and more. Here’s an example of how to find the day of the week for a given date:
$date = '2022-05-15';
$dayOfWeek = date('l', strtotime($date));
echo $dayOfWeek; // Output: Sunday
4. Add or Subtract Days from a Date
With getDate(), you can easily add or subtract days from a given date. This can be useful for tasks such as calculating future or past dates, scheduling, and more. Here’s an example of how to add or subtract days from a date:
$date = '2022-05-15';
$newDate = date('Y-m-d', strtotime($date . ' +1 day'));
echo $newDate; // Output: 2022-05-16
5. Get the Number of Days in a Month
Sometimes, you may need to find out how many days are there in a specific month. With getDate(), you can easily achieve this by using the following code:
$month = 5; // May
$year = 2022;
$daysInMonth = cal_days_in_month(CAL_GREGORIAN, $month, $year);
echo $daysInMonth; // Output: 31
Conclusion
As you can see, PHP getDate() is an incredibly versatile function that can be used for a wide range of date and time-related tasks. Whether you need to convert date formats, calculate ages, find the day of the week, or perform other date manipulations, getDate() has got you covered. By mastering these hacks and tricks, you can take your PHP date manipulation skills to the next level and impress your peers with your coding prowess.
FAQs
Q: Can I use getDate() with time as well?
A: Yes, getDate() can be used with both date and time. You can manipulate time in the same way as you would manipulate dates.
Q: Are there any limitations to using getDate()?
A: While getDate() is a powerful function, IT‘s important to keep in mind that it is limited to the capabilities of the PHP language itself. For more advanced date and time manipulation, you may need to use additional libraries or tools.
Q: Can I use getDate() for timezone conversions?
A: While you can use getDate() to work with timezones, it’s important to be aware of the limitations and potential issues that can arise when working with timezones. It’s always best to use a dedicated library or tool for timezone conversions to ensure accuracy and reliability.