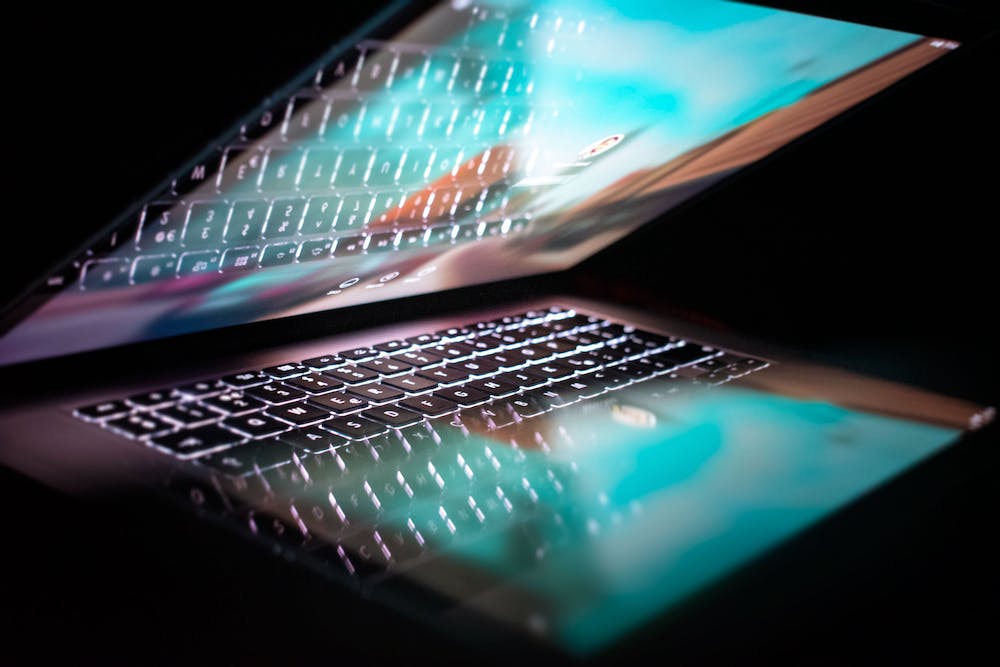
Introduction
JavaScript is a popular programming language that is widely used for developing interactive webpages. Whether you are a beginner or an experienced developer, there are always new things to learn and explore in JavaScript. In this article, we will unveil the top 10 secrets of JavaScript that will surely amaze you and enhance your understanding of the language.
1. JavaScript Closures
One of the most powerful features of JavaScript is its support for closures. Closures allow you to access variables even after the function containing them has finished executing. This can be extremely useful, especially when dealing with asynchronous operations or creating private variables. For example:
function createCounter() {
let count = 0;
return function() {
return ++count;
};
}
const counter = createCounter();
console.log(counter()); // Output: 1
console.log(counter()); // Output: 2
2. Promises and Async/Await
JavaScript introduced Promises, which are a way to handle asynchronous operations more elegantly. Promises allow you to write cleaner and more readable code. Furthermore, with the introduction of async/await in ES2017, handling asynchronous operations has become even easier. Async/await allows you to write asynchronous code that looks and behaves like synchronous code. Here’s an example using async/await:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('An error occurred:', error);
}
}
fetchData();
3. Throttling and Debouncing
Throttling and debouncing are techniques used to optimize the execution of functions that are triggered by events such as scrolling or resizing the window. Throttling limits the rate at which a function can be executed, while debouncing ensures that a function is only executed once after a series of rapid invocations. These techniques are essential for improving performance in scenarios where frequent event triggering occurs. Here’s an example of throttling using JavaScript:
function throttle(func, delay) {
let lastExecuted = 0;
return function() {
const now = Date.now();
if (now - lastExecuted >= delay) {
func();
lastExecuted = now;
}
};
}
window.addEventListener('scroll', throttle(() => {
console.log('Throttled function execution');
}, 200));
4. Hoisting
In JavaScript, variable and function declarations are hoisted to the top of their containing scope during the compilation phase. This means that you can use variables and functions before they are declared. However, only the declarations are hoisted, not the initializations. IT‘s important to be aware of hoisting to avoid unexpected behaviors in your code. For example:
console.log(message); // Output: undefined
var message = 'Hello, world!';
5. Prototype-Based Inheritance
JavaScript uses prototype-based inheritance, which is different from class-based inheritance in other languages. In JavaScript, objects can inherit properties and methods directly from other objects. This allows for flexibility and dynamic behavior. To create an object that inherits from another object, you can use the Object.create()
method. Here’s an example:
const parent = {
name: 'Parent',
greet() {
console.log(`Hello, ${this.name}!`);
}
};
const child = Object.create(parent);
child.name = 'Child';
child.greet(); // Output: Hello, Child!
6. Event Delegation
Event delegation is a technique that allows you to attach a single event listener to a parent element, instead of attaching multiple listeners to individual child elements. This is particularly useful when you have a large number of dynamically created elements or when you want to improve performance. By utilizing event bubbling, you can handle events efficiently. Here’s an example:
const parentElement = document.querySelector('.parent');
parentElement.addEventListener('click', function(event) {
if (event.target.classList.contains('child')) {
console.log('Child element clicked');
}
});
7. Modules
JavaScript modules allow you to organize your code into reusable and maintainable pieces. Modules encapsulate the implementation details of a functionality and expose a public interface for other parts of your code to use. With modules, you can easily share code between different files without polluting the global namespace. Here’s an example of a module:
// math.js
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a - b;
}
// main.js
import { add, subtract } from './math.js';
console.log(add(5, 3)); // Output: 8
console.log(subtract(10, 4)); // Output: 6
8. Date Manipulation
JavaScript provides powerful built-in methods for manipulating dates and times. Whether you need to display a custom date format, calculate the difference between two dates, or perform any other date-related operations, JavaScript has got you covered. The Date
object and its associated methods make working with dates a breeze. Here’s an example:
const now = new Date();
console.log(now.getFullYear()); // Output: 2022
console.log(now.getMonth()); // Output: 5 (month is zero-based, so 5 represents June)
console.log(now.getDate()); // Output: 28
console.log(now.getHours()); // Output: 15
console.log(now.getMinutes()); // Output: 55
console.log(now.getSeconds()); // Output: 10
9. Error Handling
Proper error handling is crucial for writing robust and reliable JavaScript code. JavaScript provides a variety of mechanisms for handling errors, such as try/catch blocks and throwing custom errors. By effectively handling errors, you can prevent your application from crashing and provide meaningful feedback to users. Here’s an example of try/catch block:
try {
// Code that might throw an error
throw new Error('Something went wrong!');
} catch (error) {
console.error(error.message); // Output: Something went wrong!
}
10. Arrow Functions
Arrow functions are a concise syntax alternative to traditional function expressions in JavaScript. They provide a more compact and readable way to define functions, especially for shorter functions. Arrow functions also have lexical scoping for the this
keyword, which solves some of the common pitfalls of regular functions. Here’s an example:
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map((number) => number ** 2);
console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Conclusion
JavaScript is a versatile language with many hidden secrets and advanced features. In this article, we revealed the top 10 secrets of JavaScript that can take your skills to the next level. From closures and promises to event delegation and arrow functions, each secret showcased a powerful aspect of the language. By mastering these secrets, you can enhance your productivity and write more efficient and maintainable JavaScript code.
FAQs
Q: How can I start learning JavaScript?
A: To start learning JavaScript, you can check out online tutorials, enroll in online courses, or read books dedicated to JavaScript programming. Additionally, practice is key, so try coding small projects to reinforce your knowledge.
Q: Can I use JavaScript on the server side?
A: Yes, you can use JavaScript on the server side using platforms like Node.js. Node.js allows you to execute JavaScript code outside of the browser, enabling backend development with JavaScript.
Q: Are JavaScript and Java the same?
A: No, JavaScript and Java are two different programming languages. Although they share similar names, syntax, and some basic concepts, they are distinct languages designed for different purposes.
Q: Is JavaScript only used for web development?
A: Although JavaScript is primarily known for its use in web development, IT has expanded beyond the web browser. JavaScript frameworks and platforms like Electron allow you to build desktop applications using JavaScript.
Q: Can I use JavaScript to create mobile applications?
A: Yes, you can develop mobile applications using JavaScript by utilizing frameworks like React Native or Ionic. These frameworks provide a way to write cross-platform mobile apps using JavaScript, which can significantly speed up development.