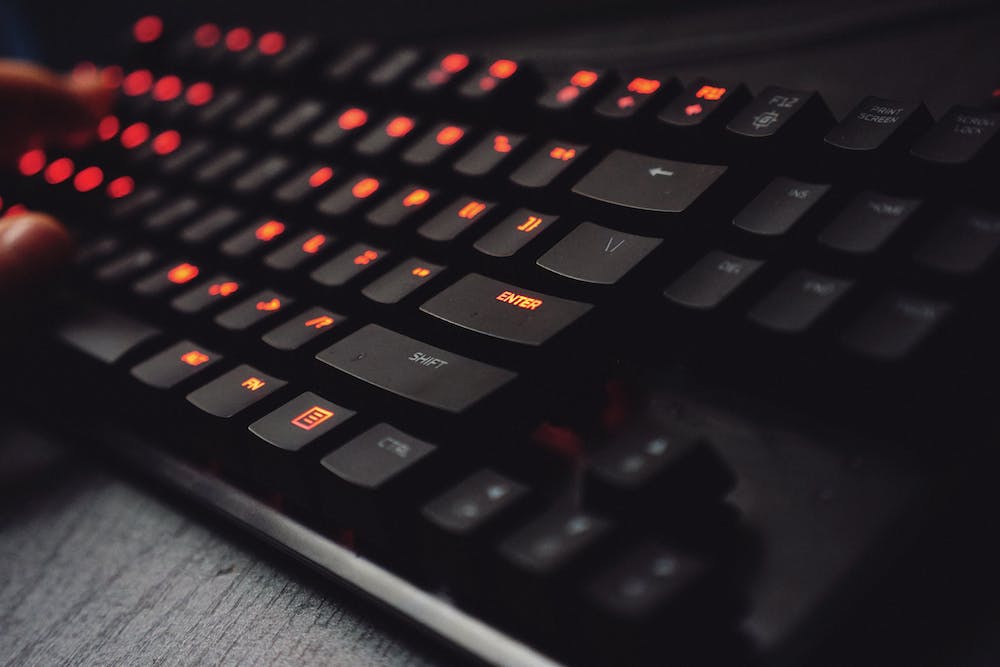
In Python, managing date and time is a common task in programming. Python provides a powerful module called “datetime” to work with dates, times, and time intervals. In this article, we will explore the top 10 Python datetime format codes that you probably didn’t know existed. These format codes will blow your mind and make date and time manipulation in Python a breeze. So, let’s dive in and discover these amazing datetime format codes!
#1 %A: Weekday Name (Full)
With the format code “%A”, you can get the full name of the weekday from a datetime object. For example:
import datetime
dt = datetime.datetime.now()
print(dt.strftime("%A"))
# Output: "Thursday"
#2 %B: Month Name (Full)
Similarly, the format code “%B” allows you to retrieve the full name of the month from a datetime object. Here’s an example:
import datetime
dt = datetime.datetime.now()
print(dt.strftime("%B"))
# Output: "July"
#3 %c: Locale’s Date and Time
The format code “%c” returns the date and time representation appropriate for the locale. This includes the date, time, and timezone information. Here’s how you can use IT:
import datetime
dt = datetime.datetime.now()
print(dt.strftime("%c"))
# Output: "Thu Jul 22 15:27:48 2021"
#4 %d: Day of the Month (Zero-Padded)
If you want to retrieve the day of the month in zero-padded form (e.g., 01, 02, …, 31), you can use the format code “%d”. Here’s an example:
import datetime
dt = datetime.datetime.now()
print(dt.strftime("%d"))
# Output: "22"
#5 %F: ISO 8601 Date
The format code “%F” provides the date representation in the ISO 8601 format (e.g., “YYYY-MM-DD”). This format is widely used in various applications and systems. Here’s how you can use it:
import datetime
dt = datetime.datetime.now()
print(dt.strftime("%F"))
# Output: "2021-07-22"
#6 %I: Hour (12-Hour Clock)
If you need to retrieve the hour in 12-hour clock format (e.g., 01, 02, …, 12), you can use the format code “%I”. Here’s an example:
import datetime
dt = datetime.datetime.now()
print(dt.strftime("%I"))
# Output: "03"
#7 %j: Day of the Year
The format code “%j” returns the day of the year as a zero-padded decimal number (e.g., 001, 002, …, 366). Here’s how you can use it:
import datetime
dt = datetime.datetime.now()
print(dt.strftime("%j"))
# Output: "203"
#8 %p: AM or PM
With the format code “%p”, you can retrieve the AM or PM designation for the time. Here’s an example:
import datetime
dt = datetime.datetime.now()
print(dt.strftime("%p"))
# Output: "PM"
#9 %U: Week Number (Sunday as the First Day of the Week)
If you want to get the week number with Sunday as the first day of the week, you can use the format code “%U”. Here’s how you can use it:
import datetime
dt = datetime.datetime.now()
print(dt.strftime("%U"))
# Output: "29"
#10 %Z: Timezone Name
The format code “%Z” provides the name of the timezone. This can be useful when you need to display the timezone information. Here’s an example:
import datetime
dt = datetime.datetime.now()
print(dt.strftime("%Z"))
# Output: "PDT"
Conclusion
Python’s datetime module offers a wide range of format codes to manipulate dates and times in various formats. In this article, we’ve explored the top 10 datetime format codes that you probably didn’t know existed. These format codes can be incredibly useful in your Python programming tasks, and they can save you a lot of time and effort in managing date and time data. So, the next time you work with datetime objects in Python, remember these format codes and make the most of them!
FAQs
Q: Can I create custom format codes for datetime objects in Python?
A: No, Python’s datetime module has a predefined set of format codes that you can use to format datetime objects. However, you can combine multiple format codes to achieve the desired output.
Q: Are datetime format codes the same in all programming languages?
A: No, different programming languages may have different format codes for manipulating dates and times. It’s important to refer to the documentation of the specific programming language you are working with.
Q: Where can I find more information about Python’s datetime module and its format codes?
A: You can refer to the official documentation of Python’s datetime module for detailed information about its format codes and other functionalities. Additionally, you can explore online resources and tutorials to deepen your understanding of datetime manipulation in Python.
With these top 10 Python datetime format codes, you now have a powerful arsenal to handle date and time data in your Python projects. Whether you’re building a web application, processing data, or generating reports, these format codes will undoubtedly come in handy. So, make sure to leverage the versatility of Python’s datetime module and elevate your date and time manipulation skills to the next level!