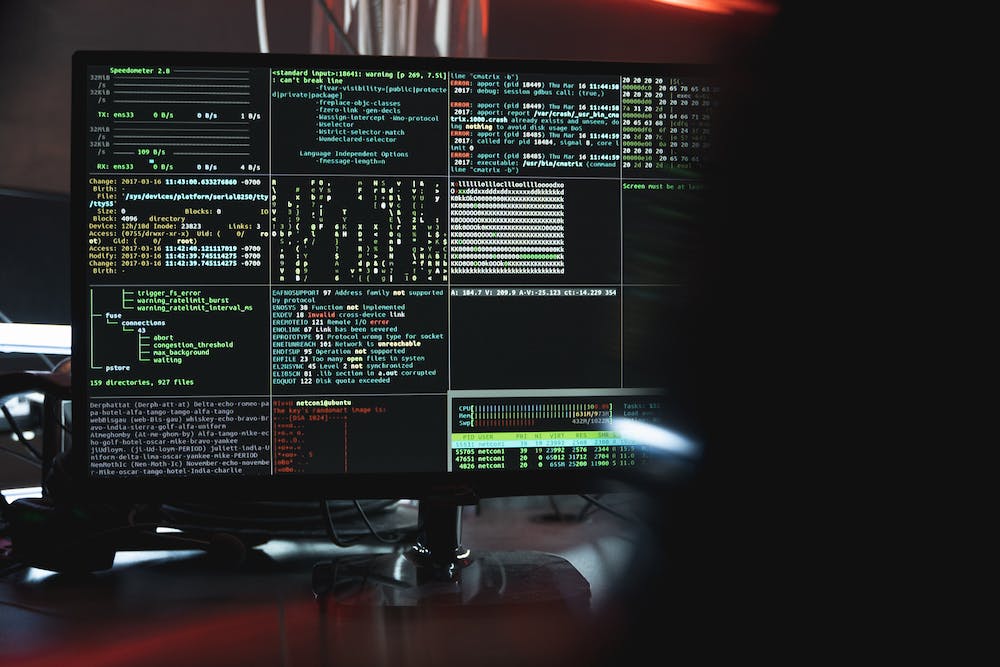
JavaScript is one of the most popular programming languages used for web development. IT is used to create interactive and dynamic content on the web. As a developer, it is essential to have a strong command on JavaScript. In this article, we will discuss the top 10 JavaScript programs that every developer should know.
1. Hello World Program
The Hello World program is a classic introductory program for any programming language. It simply displays the text “Hello, World!” on the screen. Here is a simple example in JavaScript:
console.log("Hello, World!");
2. Variables and Data Types
Understanding variables and data types is fundamental in any programming language. In JavaScript, you can declare a variable using the var
, let
, or const
keyword. Here is an example:
var name = "John";
let age = 25;
const PI = 3.14;
3. Conditional Statements
Conditional statements allow you to make decisions in your code. In JavaScript, you can use the if-else
statement to perform different actions based on different conditions. Here is an example:
let x = 10;
if (x > 5) {
console.log("x is greater than 5");
} else {
console.log("x is less than or equal to 5");
}
4. Loops
Loops are used to execute a block of code repeatedly. In JavaScript, you can use the for
loop, while
loop, or do-while
loop. Here is an example of a for
loop:
for (let i = 0; i < 5; i++) {
console.log(i);
}
5. Functions
Functions are reusable blocks of code that perform a specific task. In JavaScript, you can define a function using the function
keyword. Here is an example:
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("John");
6. Arrays
Arrays are used to store multiple values in a single variable. In JavaScript, you can create an array using square brackets. Here is an example:
let fruits = ["apple", "banana", "orange"];
console.log(fruits[1]); // Output: banana
7. Objects
Objects are used to store key-value pairs. In JavaScript, you can create an object using curly braces. Here is an example:
let person = {
name: "John",
age: 25,
city: "New York"
};
console.log(person.name); // Output: John
8. DOM Manipulation
Document Object Model (DOM) manipulation is essential for interacting with HTML elements on a web page. In JavaScript, you can use the document
object to access and manipulate HTML elements. Here is an example:
let heading = document.getElementById("heading");
heading.innerHTML = "Hello, World!";
9. Event Handling
Event handling is important for creating interactive web applications. In JavaScript, you can use the addEventListener
method to attach an event to an HTML element. Here is an example:
let button = document.getElementById("button");
button.addEventListener("click", function() {
alert("Button clicked!");
});
10. Asynchronous Programming
Asynchronous programming is used to perform tasks concurrently without blocking the main thread. In JavaScript, you can use callbacks, promises, or async/await for asynchronous programming. Here is an example using promises:
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data fetched successfully");
}, 2000);
});
}
fetchData().then(data => {
console.log(data); // Output: Data fetched successfully
});
Conclusion
These top 10 JavaScript programs are essential for every developer to know. They form the foundation of JavaScript programming and are used in almost every web application. By mastering these programs, you can become a proficient JavaScript developer and build robust and interactive web applications.
FAQs
What is JavaScript?
JavaScript is a programming language used to create interactive and dynamic content on the web.
What are the essential programs every JavaScript developer should know?
Some essential programs every JavaScript developer should know include the Hello World program, variables and data types, conditional statements, loops, functions, arrays, objects, DOM manipulation, event handling, and asynchronous programming.
How can I learn and improve my JavaScript programming skills?
You can learn and improve your JavaScript programming skills by practicing regularly, building small projects, and taking online courses or tutorials. Additionally, collaborating with other developers and studying code from open source projects can also help improve your skills.
Which JavaScript framework should I learn?
There are several JavaScript frameworks available, such as React, Angular, and Vue.js. The framework you should learn depends on your specific project requirements and personal preferences. It’s essential to research and understand the unique features and use cases of each framework before making a decision.
What are some resources to further my understanding of JavaScript?
Some resources to further your understanding of JavaScript include MDN Web Docs, online courses on platforms like Udemy and Coursera, and books such as “Eloquent JavaScript” by Marijn Haverbeke. Additionally, participating in developer communities and attending tech conferences can also provide valuable insights and knowledge.