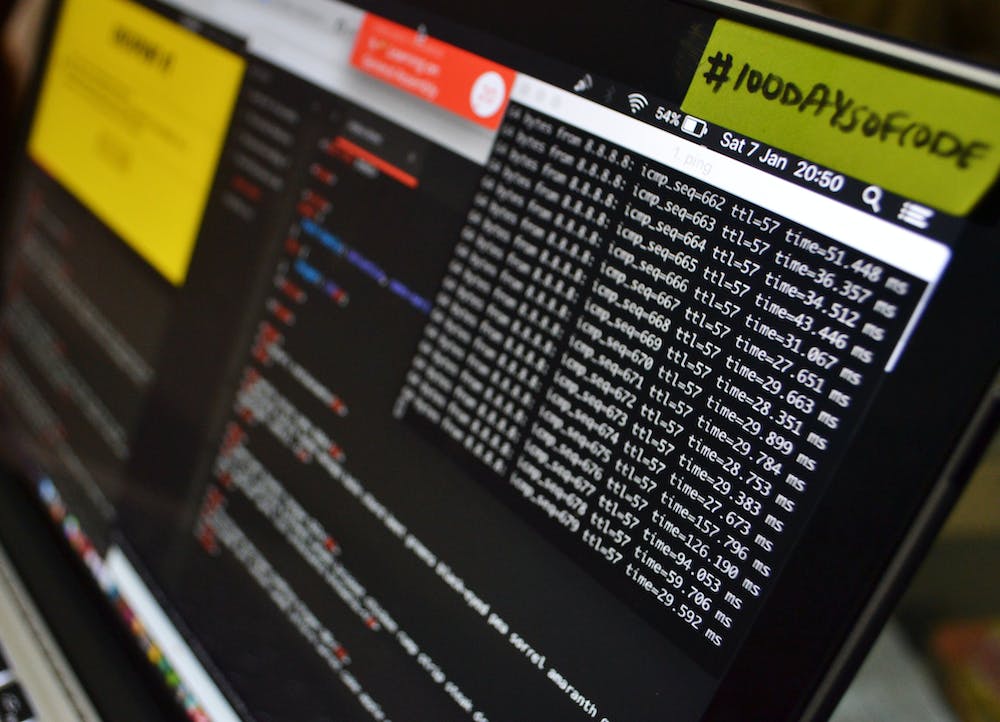
In JavaScript, arrays play a vital role in managing and manipulating data. There are numerous built-in methods that allow developers to perform various operations on arrays efficiently. In this article, we will explore the top 10 JavaScript list methods that every developer should know.
1. forEach()
The forEach()
method is used to execute a provided function once for each array element. IT is particularly useful for iterating through an array and performing operations on each element.
2. map()
The map()
method creates a new array with the results of calling a provided function on every element in the array. It is an excellent choice for transforming the elements of an array without changing the original array.
3. filter()
The filter()
method creates a new array with all elements that pass the test implemented by the provided function. It is commonly used to extract specific elements from an array based on a condition.
4. reduce()
The reduce()
method applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single value. This method is extremely powerful and can be used for various purposes such as calculating the sum of array elements or flattening an array.
5. find()
The find()
method returns the value of the first element in the array that satisfies the provided testing function. It is particularly useful when you need to find a specific element in an array based on a condition.
6. findIndex()
Similar to the find()
method, the findIndex()
method returns the index of the first element in the array that satisfies the provided testing function. It comes in handy when you need to find the index of a specific element in an array.
7. some()
The some()
method tests whether at least one element in the array passes the test implemented by the provided function. It is useful for checking if the array contains at least one element that satisfies a condition.
8. every()
The every()
method tests whether all elements in the array pass the test implemented by the provided function. It is particularly useful for checking if all elements in the array satisfy a specific condition.
9. sort()
The sort()
method is used to sort the elements of an array in place and returns the sorted array. It is commonly used for sorting arrays of primitive types, but can be customized to sort complex data structures.
10. concat()
The concat()
method is used to merge two or more arrays and returns a new array. It is particularly useful when you need to combine multiple arrays into a single array.
Conclusion
Understanding and mastering these top 10 JavaScript list methods is essential for any developer working with arrays. These methods provide a powerful set of tools for managing and manipulating array data, and can significantly improve the efficiency and readability of your code. By incorporating these methods into your development workflow, you can unlock the full potential of JavaScript arrays and streamline your programming tasks.
FAQs
What is the difference between forEach() and map() methods?
The forEach()
method executes a provided function once for each array element and does not return a new array. In contrast, the map()
method creates a new array with the results of calling a provided function on every element in the array.
When should I use the reduce() method?
The reduce()
method is useful when you need to accumulate a single value from the elements of an array, such as finding the sum of array elements, calculating an average, or flattening an array of arrays.
Can the sort() method be customized to handle complex sorting requirements?
Yes, the sort()
method can be customized by providing a compare function that defines the sorting order. This allows you to handle complex sorting requirements for non-primitive data types.
Are there any performance considerations when using these list methods?
While these list methods provide powerful functionality, it is important to consider the performance implications, especially when working with large arrays. In some cases, using these methods may result in better performance compared to manual iteration and manipulation of arrays.
In summary, JavaScript list methods offer a robust set of tools for working with arrays. By mastering these methods, developers can streamline their array manipulation tasks and improve the efficiency and readability of their code.