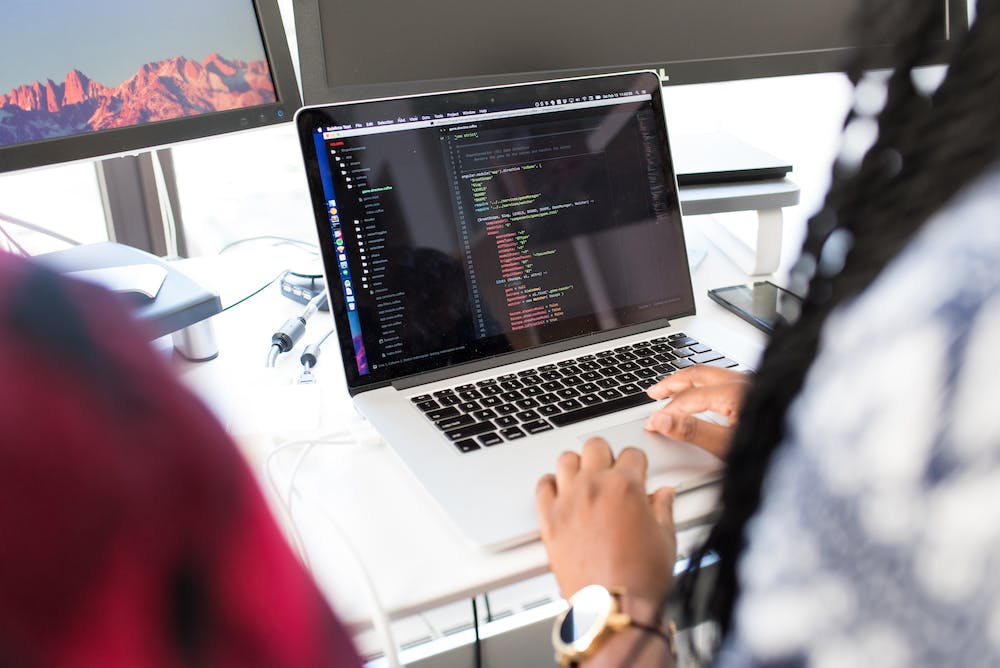
PHP is a popular scripting language that is widely used for web development. IT offers a wide range of functions that allow developers to perform various tasks efficiently. In this article, we will discuss the top 10 essential PHP functions that every developer should know.
1. echo()
The echo() function is used to output one or more strings. IT is commonly used to display content on a web page. For example, echo(“Hello, World!”); will display the text “Hello, World!” on the web page.
2. strlen()
The strlen() function is used to calculate the length of a string. IT returns the number of characters in a string. For example, strlen(“Hello, World!”); will return 13.
3. strpos()
The strpos() function is used to find the position of the first occurrence of a substring in a string. IT returns the position as an integer if found, otherwise IT returns FALSE. For example, strpos(“Hello, World!”, “World”); will return 7.
4. array()
The array() function is used to create an array. IT can be used to create both indexed and associative arrays. For example, $fruits = array(“apple”, “banana”, “orange”); will create an indexed array of fruits.
5. count()
The count() function is used to count the number of elements in an array. IT returns the number of elements as an integer. For example, count($fruits); will return 3.
6. date()
The date() function is used to format a date and time. IT can be used to display the current date and time, as well as manipulate dates and times. For example, date(“Y-m-d”); will display the current date in the format YYYY-MM-DD.
7. strtolower()
The strtolower() function is used to convert a string to lowercase. IT returns the converted string. For example, strtolower(“HELLO”); will return “hello”.
8. strtoupper()
The strtoupper() function is used to convert a string to uppercase. IT returns the converted string. For example, strtoupper(“hello”); will return “HELLO”.
9. explode()
The explode() function is used to split a string into an array. IT takes two parameters – the delimiter and the string to split. For example, $str = “apple, banana, orange”; $fruits = explode(“, “, $str); will create an array $fruits with three elements.
10. file_get_contents()
The file_get_contents() function is used to read the contents of a file into a string. IT returns the file contents as a string. For example, $content = file_get_contents(“file.txt”); will read the contents of the file.txt into the $content variable.
FAQs
Q1. How can I check if a variable is empty in PHP?
You can use the empty() function to check if a variable is empty. IT returns TRUE if the variable is empty, otherwise IT returns FALSE. For example, if(empty($name)) { echo “Name is empty”; } will display “Name is empty” if the $name variable is empty.
Q2. What is the difference between include() and require() functions?
The include() and require() functions are used to include and evaluate the specified file in PHP. The main difference between them is how they handle errors. If a file is not found by the include() function, a warning will be issued and the script will continue execution. On the other hand, if a file is not found by the require() function, a fatal error will be issued and the script will stop execution.
Q3. How can I redirect in PHP?
You can use the header() function to redirect in PHP. IT is used to send a raw HTTP header to the browser. For example, header(“Location: https://example.com”); will redirect the user to the specified URL.
Q4. How can I send email in PHP?
You can use the mail() function to send email in PHP. IT is used to send email from a web server. For example, mail(“[email protected]”, “Subject”, “Message”); will send an email with the specified subject and message to the recipient’s email address.
Q5. How can I validate user input in PHP?
You can use various techniques to validate user input in PHP, such as regular expressions or built-in functions like filter_var(). Regular expressions can be used to validate complex patterns, while filter_var() provides a convenient way to validate common data types like email addresses or URLs.
In conclusion, these are some of the essential PHP functions that every developer should know. Mastering these functions will significantly enhance your ability to develop efficient and effective PHP applications.