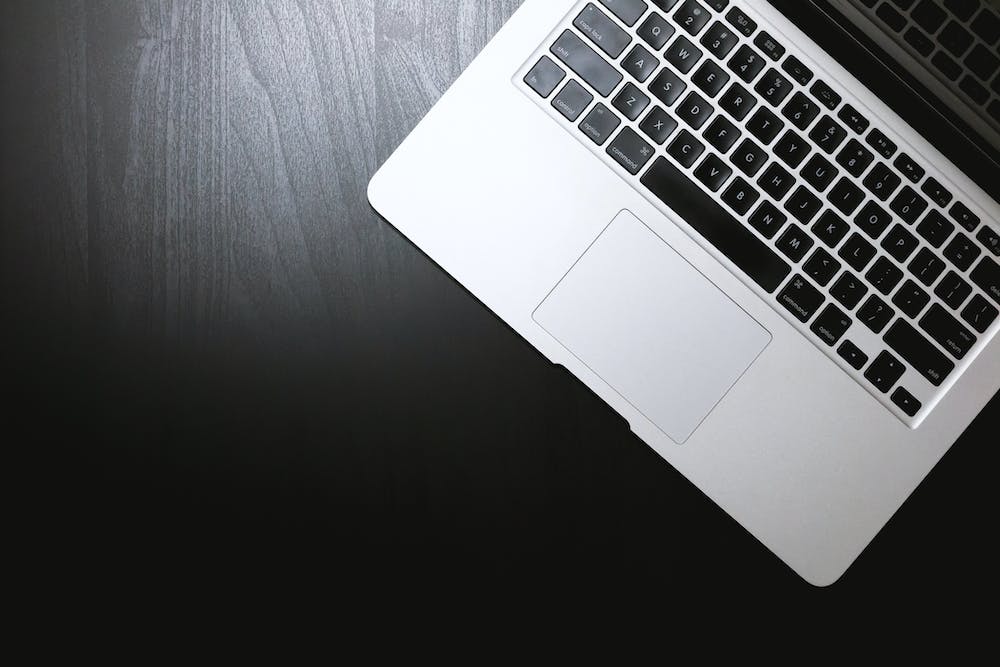
Python is a powerful and widely-used programming language that is known for its simplicity and readability. IT is frequently used in web development, data analysis, machine learning, and automation, making it an essential skill for any aspiring software developer or data scientist. In this ultimate guide, we will provide you with everything you need to know to learn Python and become proficient in it.
Why Learn Python?
There are several reasons why learning Python is a great investment in your future. Firstly, Python is beginner-friendly, with an easy-to-understand syntax that makes it a great language to start with. This means that even individuals with no prior programming experience can quickly pick up Python and start building useful applications.
Furthermore, Python has a large and active community, which means that there are plenty of resources, libraries, and frameworks available for you to use. This makes it a versatile language that can be used for a wide range of applications, from web development to scientific computing.
Getting Started with Python
The first step in learning Python is to install the Python interpreter on your computer. You can download the latest version of Python from the official Website, and choose the appropriate installer for your operating system.
Once Python is installed, you can start writing and running Python code using a text editor or an integrated development environment (IDE). There are several popular IDEs for Python, such as PyCharm, VS Code, and Jupyter Notebook, each offering different features and benefits.
Basic Python Syntax
Python has a clean and easy-to-read syntax, which makes it a great language for both beginners and experienced programmers. Here are some basic Python syntax rules that you should be familiar with:
- Python statements are typically written on separate lines.
- Indentation is used to define code blocks, rather than curly braces or keywords like “end” or “fi” in other languages.
- Python uses dynamic typing, which means that you don’t have to declare the data type of a variable when you define it.
- Python uses the # symbol to indicate comments, which are ignored by the interpreter.
Python Data Types and Variables
Python supports several data types, including integers, floating-point numbers, complex numbers, strings, lists, tuples, dictionaries, and sets. Variables in Python are used to store data values, and you can assign a value to a variable using the equals sign (=).
Here are some examples of variables and data types in Python:
x = 5 # integer
y = 3.14 # floating-point number
name = "John" # string
fruits = ["apple", "banana", "cherry"] # list
person = {"name": "John", "age": 30} # dictionary
Control Flow and Loops in Python
Python supports several control flow and loop structures, which are used to make decisions and repeat code blocks. The most common control flow structures in Python are if, elif, and else, while the most common loop structures are for and while.
Here is an example of an if statement and a for loop in Python:
if x > 10:
print("x is greater than 10")
elif x < 10:
print("x is less than 10")
else:
print("x is equal to 10")
for fruit in fruits:
print(fruit)
Functions and Modules in Python
Functions are used to group code into reusable blocks, which can be called from other parts of the program. In Python, you can define functions using the def keyword, and you can pass arguments to a function and return values from it.
Modules are used to organize related functions and variables into separate files, which can be imported and used in other programs. Python comes with a standard library of modules, and you can also create your own custom modules.
def greet(name):
return "Hello, " + name
import math
print(math.sqrt(16))
Working with Files and I/O in Python
Python provides several built-in functions and modules for working with files and performing input/output operations. You can open, read, write, and close files using the built-in open() function and the file object’s methods.
Here is an example of reading and writing to a file in Python:
# Reading from a file
file = open("example.txt", "r")
print(file.read())
file.close()
# Writing to a file
file = open("example.txt", "w")
file.write("Hello, World!")
file.close()
Advanced Python Topics
Once you have mastered the basics of Python, you can explore more advanced topics and techniques to become a proficient Python programmer. Some of the advanced topics in Python include:
- Object-oriented programming (OOP)
- Error handling and exception handling
- Regular expressions and string manipulation
- Database access and manipulation with SQL
- Web scraping and automation
- Data analysis and visualization with pandas and matplotlib
- Machine learning and artificial intelligence with libraries like scikit-learn and TensorFlow
Learning about these advanced topics will make you a more well-rounded and versatile programmer, and it will open up a wide range of career opportunities in fields like web development, data science, and machine learning.
Best Practices for Learning Python
Learning Python, like any new skill, requires patience, dedication, and practice. Here are some best practices that you should keep in mind as you embark on your Python learning journey:
- Start with simple projects: Begin your learning journey by working on simple projects and gradually move on to more complex ones. This will help you reinforce your understanding of Python and build confidence in your programming skills.
- Practice regularly: Consistent practice is key to mastering Python. Set aside time each day to write code, solve problems, and experiment with new concepts.
- Engage with the community: Join online forums, attend meetups, and participate in coding challenges to connect with other Python enthusiasts and learn from their experiences.
- Contribute to open-source projects: Contributing to open-source projects is a great way to apply what you have learned, gain real-world experience, and build a professional portfolio.
- Stay updated: The field of programming is constantly evolving, so make sure to stay updated with the latest Python developments, libraries, and best practices.
Conclusion
Python is a powerful and versatile programming language that is ideal for beginners and experienced programmers alike. With its easy-to-read syntax, active community, and extensive library of modules, Python is an essential skill for anyone interested in software development, data analysis, and automation. By following the best practices outlined in this guide and continuously learning and practicing, you can become a proficient Python programmer and open up a wide range of career opportunities. So, what are you waiting for? Get started with Python today and take your programming skills to the next level!
FAQs
Q: What are some good resources to learn Python?
A: There are plenty of resources available for learning Python, including online tutorials, interactive coding platforms, books, and video courses. Some popular resources include Codecademy, Coursera, Udemy, and the official Python documentation.
Q: Is Python a good language for beginners?
A: Yes, Python is an excellent language for beginners due to its simple and readable syntax. It is a great language to start with if you have no prior programming experience.
Q: Can Python be used for web development?
A: Yes, Python is commonly used for web development, thanks to frameworks like Django and Flask. These frameworks make it easy to build complex web applications using Python.
Q: What are some career opportunities for Python programmers?
A: Python programmers can pursue various career opportunities, including web developer, data analyst, software engineer, machine learning engineer, and data scientist. Python is also widely used in fields like finance, healthcare, and scientific research.
Q: How can I improve my Python skills?
A: To improve your Python skills, you should practice regularly, work on projects, engage with the community, and stay updated with the latest Python developments. Contributing to open-source projects and attending coding challenges can also help you gain practical experience.