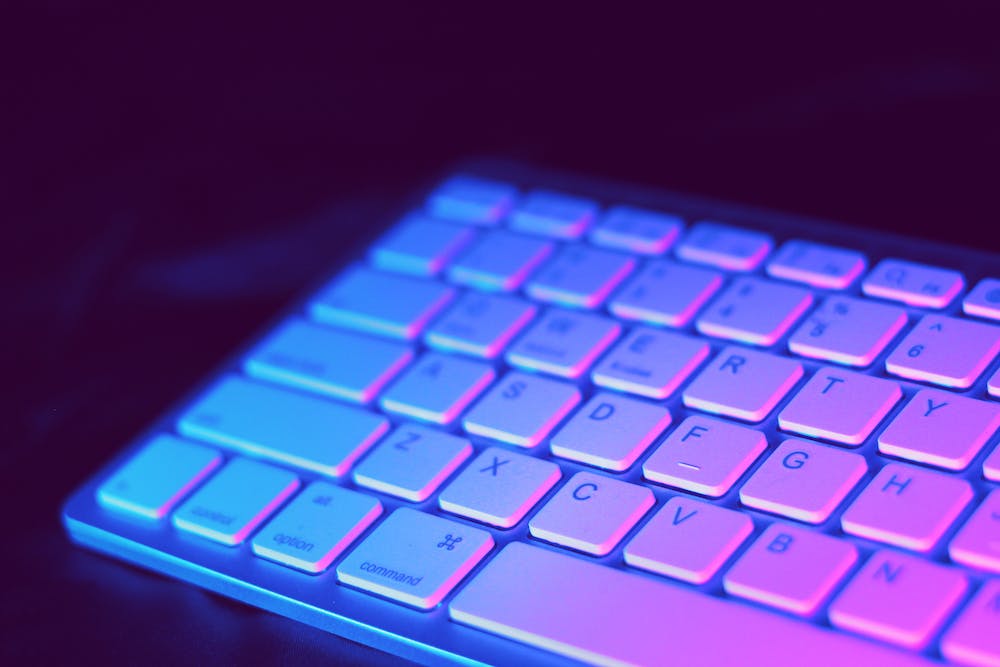
PHP is a powerful server-side scripting language that is widely used for web development. IT provides various features and functions that make it a popular choice for building dynamic websites and web applications. One of the key functions in PHP that significantly enhances the modularity and reusability of code is require()
.
Understanding require() in PHP
The require()
function in PHP is used to include a specific file into the current script. This means that the code from the included file is copied into the file that contains the require()
statement. If the file cannot be included (i.e., it does not exist or cannot be accessed), PHP will generate a fatal error and halt the execution of the script.
There are different variations of the require()
function in PHP, such as require_once()
and include()
, each with its own specific use cases. However, in this article, we will focus on the standard require()
function and its impact on web development.
The Power of Code Reusability
One of the primary advantages of using require()
in web development is the ability to reuse code across multiple files. This is particularly useful when a certain piece of code, such as a function or a configuration file, needs to be shared and accessed by different parts of a Website or web application.
By using require()
, developers can create modular and organized code structures, where common functionality can be placed in separate files and then included wherever necessary. This not only improves the maintainability of the codebase but also reduces the likelihood of redundancy and errors.
Example:
// config.php
<?php
$database_host = 'localhost';
$database_username = 'root';
$database_password = 'password';
$database_name = 'example_database';
?>
// index.php
<?php
require('config.php');
// Use the database configuration variables here
?>
Enhancing Modularity and Scalability
Another significant advantage of using require()
is its impact on the modularity and scalability of a web project. As websites and web applications grow in complexity, the ability to organize and manage code becomes increasingly important.
With require()
, developers can create separate modules for different aspects of a project, such as user authentication, database interactions, and templating. These modules can then be included into the main scripts as needed, allowing for a more structured and manageable codebase.
Example:
// authentication.php
<?php
require('db.php');
// Code for user authentication
?>
// index.php
<?php
require('authentication.php');
// Use the authentication functionality here
?>
Improving Security and Error Handling
From a security perspective, the use of require()
in web development can also contribute to better error handling and more secure code execution. Since PHP generates a fatal error when a required file cannot be included, it forces developers to ensure that the necessary files are present and accessible.
By strategically using require()
and require_once()
where appropriate, developers can implement a more robust error-handling mechanism and prevent the execution of incomplete or compromised scripts. This can help mitigate the risk of security vulnerabilities that arise from missing or incorrect file dependencies.
Example:
// functions.php
<?php
require('logger.php');
// Register error and exception handlers
?>
// index.php
<?php
require('functions.php');
// Execute the main functionality of the script
?>
Conclusion
The require()
function in PHP is a powerful tool for enhancing the functionality and maintainability of web development projects. By allowing for code reusability, modularity, and improved security, require()
plays a crucial role in creating efficient and organized codebases.
Web developers can leverage the power of require()
to build scalable and secure websites and web applications that are easier to manage and maintain over time. With proper implementation and understanding of its capabilities, require()
can significantly contribute to the success of PHP-based projects.
FAQs
Q: What is the difference between require() and include() in PHP?
A: The primary difference between require() and include() is that require() will produce a fatal error if the file cannot be included, while include() will only produce a warning and continue the script execution.
Q: When should I use require_once() instead of require()?
A: You should use require_once() when you want to include a file and ensure that it is only included once, regardless of how many times the statement is executed within the script.
Q: Can I use require() to include files from external sources?
A: Yes, require() can be used to include files from external sources, such as URLs, as long as the allow_url_fopen directive is enabled in the PHP configuration.
Q: Is it considered best practice to use require() for all file inclusions?
A: While require() offers stronger error handling compared to include(), the choice between the two depends on the specific requirements of the project. It’s important to consider the impact of including a file on the overall functionality and performance of the script.
Q: Can require() be used to load a file based on a conditional statement?
A: Yes, require() can be used within conditional statements to include files dynamically based on certain conditions. This can be useful for loading specific files depending on the context of the script.
References: