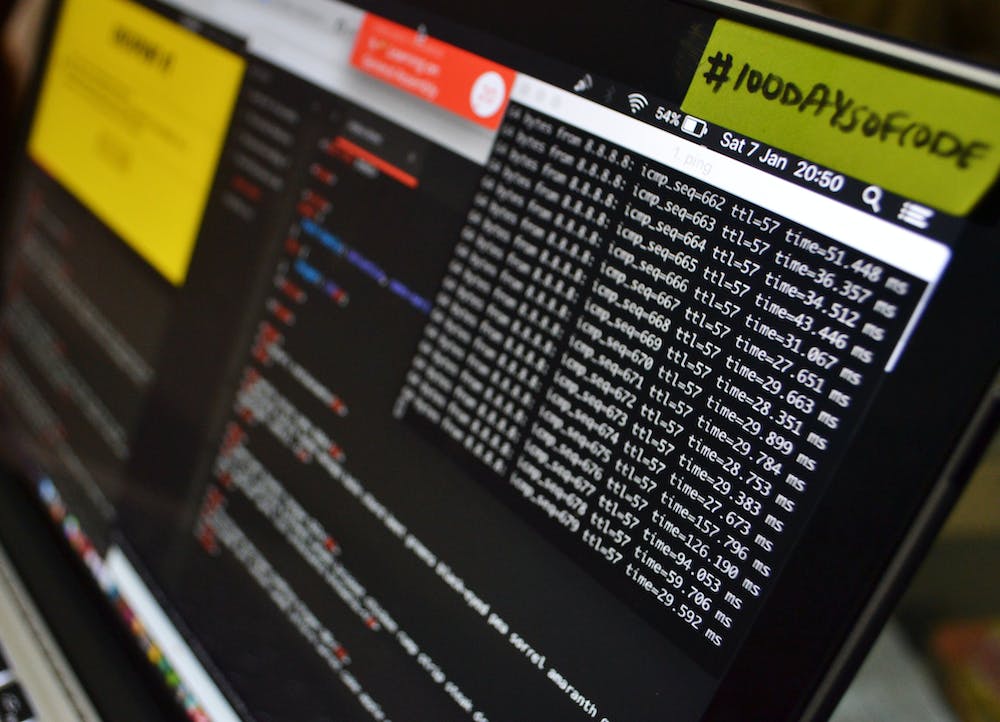
PHP is a powerful scripting language that is widely used for web development. One of its key features is the ability to handle URL parameters using the GET method. This allows developers to create dynamic and interactive web applications that can process user input and display customized content. In this article, we will explore the power of PHP and how IT can be leveraged to create dynamic web applications using the GET URL methodologies.
Understanding the GET Method
The GET method is one of the two main methods used for sending data through a URL. It is commonly used for retrieving data from a server, such as fetching web pages or querying a database. When a form is submitted using the GET method, the form data is appended to the URL as query parameters. This makes it easy to see and share the data being sent, but it also has limitations on the amount of data that can be sent and is not secure for sensitive information.
Using PHP to Process GET Parameters
PHP provides a superglobal array called $_GET
that can be used to access the GET parameters sent to a page. This array contains key-value pairs of the parameters in the URL, making it easy to access and process the data. For example, if a URL contains ?name=John&age=30
, the $_GET
array will have the values 'John'
and 30
accessible by the keys 'name'
and 'age'
respectively.
Here is an example of using PHP to process GET parameters:
<?php
// Check if the name parameter is set
if(isset($_GET['name'])) {
// Display a personalized message
echo "Hello, " . $_GET['name'] . "!";
} else {
// Display a default message
echo "Hello, Guest!";
}
?>
In this example, we check if the 'name'
parameter is set in the URL. If it is, we display a personalized message with the value of the 'name'
parameter. If it is not set, we display a default message for guests. This simple example demonstrates the power of using PHP to process GET parameters and create dynamic content based on user input.
Building Dynamic Web Applications with PHP and GET Parameters
Using PHP and GET parameters, developers can build dynamic web applications that respond to user input and generate customized content. For example, an e-commerce Website could use GET parameters to filter products based on category, price, or other criteria. A news website could use GET parameters to display articles based on the selected category or search query. The possibilities are endless, and the ability to process user input through the URL makes it easy to create interactive and personalized web experiences.
Here is an example of using PHP and GET parameters to build a simple product filtering feature for an e-commerce website:
<?php
// Get the selected category from the URL
if(isset($_GET['category'])) {
$selectedCategory = $_GET['category'];
// Query the database for products in the selected category
$products = // Query products from the database based on the selected category
// Display the filtered products
foreach($products as $product) {
echo $product['name'] . " - " . $product['price'];
}
} else {
// Display all products
$products = // Query all products from the database
foreach($products as $product) {
echo $product['name'] . " - " . $product['price'];
}
}
?>
In this example, we check if the 'category'
parameter is set in the URL. If it is, we query the database for products in the selected category and display them. If it is not set, we display all products. This simple example shows how PHP and GET parameters can be used to create dynamic and personalized content based on user input.
Best Practices for Using GET Parameters in PHP
While the GET method is powerful for handling URL parameters, it is important to follow best practices to ensure security and reliability. Here are some best practices for using GET parameters in PHP:
- Sanitize and validate input: Always sanitize and validate user input to prevent security vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks.
- Avoid using sensitive information: Do not use the GET method to send sensitive information such as passwords or user credentials, as the data is visible in the URL.
- Limit the amount of data: The GET method has a limitation on the amount of data that can be sent through the URL. Avoid sending large amounts of data using the GET method.
- Use URL encoding: When sending special characters or spaces in the URL, use URL encoding to ensure that the data is properly interpreted by the server.
Conclusion
The power of PHP and the GET URL methodologies provides developers with a powerful tool for creating dynamic web applications that respond to user input and generate customized content. By leveraging the $_GET
superglobal array, developers can access and process GET parameters with ease, allowing for the creation of interactive and personalized web experiences. However, it is important to follow best practices when using GET parameters to ensure the security and reliability of the web application.
FAQs
Q: Can I use the GET method to send sensitive information?
A: It is not recommended to use the GET method to send sensitive information, as the data is visible in the URL and can be easily intercepted.
Q: Is there a limitation on the amount of data that can be sent using the GET method?
A: Yes, the GET method has a limitation on the amount of data that can be sent through the URL. If you need to send large amounts of data, consider using the POST method instead.
Q: How can I prevent security vulnerabilities when using GET parameters in PHP?
A: It is important to sanitize and validate user input to prevent security vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks. Always validate and sanitize input before using it in your application.
Q: Can I use GET parameters for URL tracking and analytics?
A: Yes, GET parameters can be used for URL tracking and analytics. By appending tracking parameters to the URL, you can track user behavior and gather analytics data for your web application.
Q: Are there any PHP libraries or frameworks that can help with processing GET parameters?
A: There are various PHP libraries and frameworks that can help with processing GET parameters, such as Laravel, Symfony, and CodeIgniter. These frameworks provide built-in functionality for handling URL parameters and can make the process easier and more efficient.