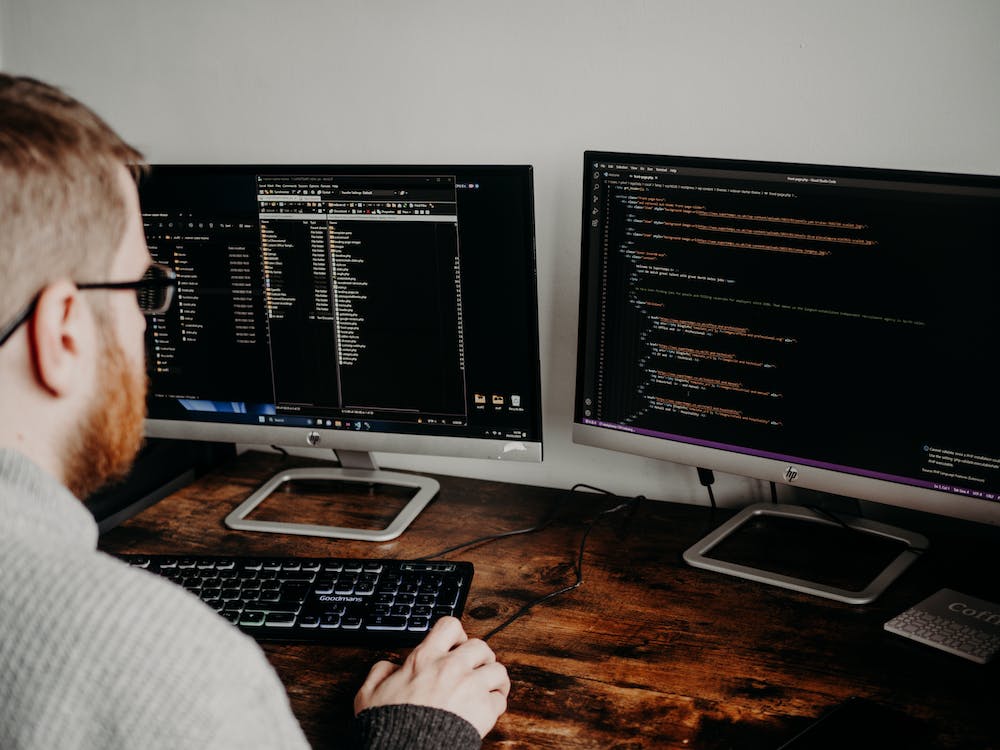
When IT comes to programming, understanding the fundamentals of program structure and interpretation is essential for building efficient and reliable software. Whether you’re a beginner or an experienced programmer, having a solid grasp of these concepts is crucial for writing clean, maintainable, and scalable code.
What is Program Structure?
Program structure refers to the way in which a program is organized. It involves breaking down a program into smaller, manageable parts, often referred to as modules or functions, and defining how these parts interact with each other. A well-structured program is easier to understand, maintain, and extend, as it promotes code reusability and reduces complexity.
There are several key elements of program structure, including:
- Modularity: Breaking down a program into smaller, independent modules that can be easily maintained and reused.
- Abstraction: Hiding the complexity of a module’s implementation and exposing only the essential details to the rest of the program.
- Encapsulation: Bundling data and methods that operate on the data into a single unit, known as a class, and restricting access to the data through defined interfaces.
- Decomposition: Breaking down a problem into smaller, more manageable sub-problems, allowing for easier problem-solving and code organization.
What is Program Interpretation?
Program interpretation, also known as code interpretation, refers to the process of executing a program by interpreting and executing its source code directly. This is in contrast to program compilation, where the source code is translated into machine code before execution. Interpreted programs are generally easier to develop and debug, as changes to the source code can be immediately executed without the need for recompilation.
There are various ways in which programming languages can be interpreted, including:
- Scripting Languages: Languages like Python, Ruby, and JavaScript are often interpreted, allowing for rapid prototyping and development.
- Virtual Machines: Some languages, such as Java and C#, are first compiled into an intermediate bytecode, which is then interpreted by a virtual machine at runtime.
- Just-In-Time Compilation: This hybrid approach involves interpreting the source code initially and compiling it into machine code as it runs for improved performance.
Key Concepts in Program Structure and Interpretation
Understanding the fundamentals of program structure and interpretation involves familiarizing yourself with several key concepts that are essential for writing high-quality code. Some of these concepts include:
Data Types and Structures
Data types and structures form the foundation of any program, defining how data is stored, accessed, and manipulated. Understanding different data types, such as integers, floating-point numbers, strings, and booleans, is crucial for writing efficient and bug-free code. Furthermore, learning about data structures, such as arrays, lists, queues, and trees, allows for more complex data organization and manipulation.
Control Structures
Control structures, such as conditionals (if-else statements) and loops (for, while, and do-while), define the flow of execution within a program. Mastering these structures enables you to make decisions, iterate over data, and control the program’s behavior based on specific conditions.
Functions and Procedures
Functions and procedures encapsulate a set of instructions that perform a specific task, promoting code reuse and modularity. Understanding how to define, call, and pass parameters to functions is crucial for building modular and maintainable programs.
Object-Oriented Programming (OOP)
Object-oriented programming is a programming paradigm that promotes the use of objects to represent data and behavior. Learning about classes, objects, inheritance, polymorphism, and encapsulation is essential for building complex, reusable, and maintainable software.
Error Handling and Exception Control
Dealing with errors and exceptions is a critical aspect of writing reliable and robust programs. Understanding how to handle and recover from errors, as well as how to raise and catch exceptions, can prevent unexpected program crashes and improve overall program stability.
Best Practices in Program Structure and Interpretation
While understanding the core concepts of program structure and interpretation is important, following best practices can further enhance the quality of your code. Some best practices to consider include:
Code Readability
Writing code that is easy to read and understand is crucial for improving maintainability and collaboration. Use meaningful variable and function names, and properly indent and format your code for clarity.
Modularity and Reusability
Break down your code into smaller, reusable modules and functions to reduce complexity and redundancy. This promotes code reusability and makes it easier to maintain and extend your programs.
Documentation
Include comments and documentation to explain the purpose and functionality of your code. Documenting your code helps others understand its logic and aids in debugging and maintenance.
Error Handling
Implement robust error handling and exception control to ensure your programs gracefully handle unexpected situations. Proper error handling can prevent crashes and improve the user experience.
Testing and Debugging
Thoroughly test your code and debug any issues before deployment. Utilize testing frameworks and debugging tools to identify and fix errors in your programs.
Conclusion
Understanding the fundamentals of program structure and interpretation is crucial for writing clean, maintainable, and efficient code. By grasping concepts such as program organization, data types, control structures, functions, and best practices, you can build reliable and scalable software applications. Continuously honing your skills in program structure and interpretation will not only improve your coding abilities but also enhance the quality and performance of your programs.
FAQs
Q: What is the difference between compilation and interpretation?
A: Compilation involves translating source code into machine code before execution, resulting in an executable file. Interpretation, on the other hand, involves directly executing the source code without prior compilation.
Q: How does program structure affect software development?
A: Well-structured programs are easier to maintain, extend, and collaborate on, leading to improved productivity and code quality. They promote modularity, reusability, and code readability.
Q: Why is error handling important in program development?
A: Effective error handling prevents unexpected program crashes and improves the overall stability and reliability of software, leading to a better user experience.
Q: Should I prioritize speed or readability when writing code?
A: Striking a balance between speed and readability is essential. While writing efficient code is important, prioritizing readability ensures that your code can be easily understood, maintained, and extended by others.
Q: How can I improve my understanding of program structure and interpretation?
A: Practice, study, and collaborate with other programmers to deepen your understanding of these concepts. Utilize resources such as online courses, books, and coding communities to enhance your skills.