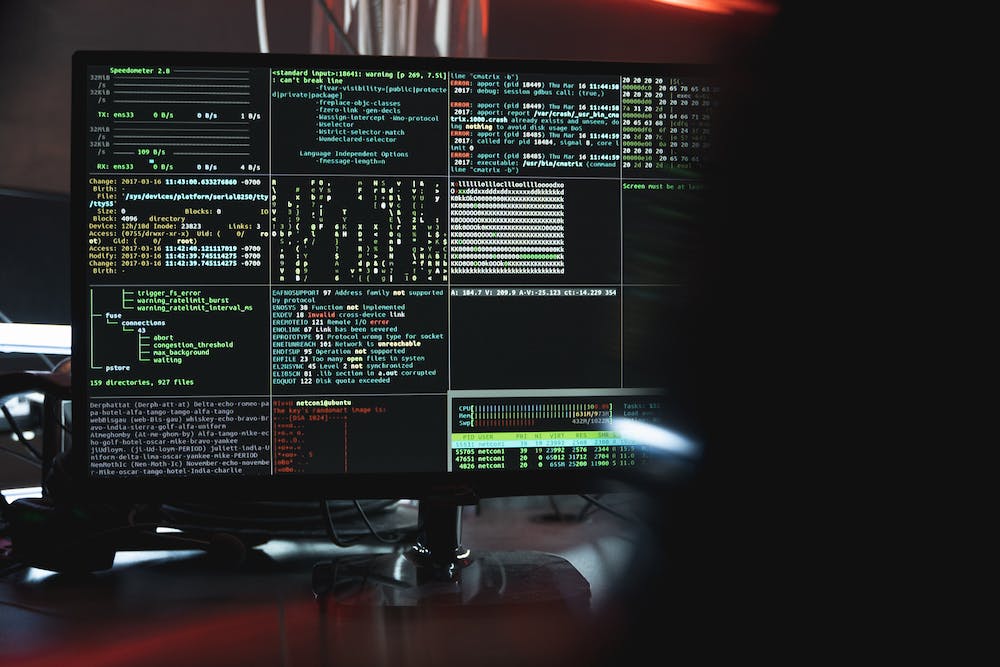
In PHP, a variable is used to store data, such as a name or a number. Variables can be used to store different types of data, like text or numbers. In this article, we will explore the basics of PHP variables, including how to declare, assign, and use them effectively in your code.
What is a Variable?
A variable in PHP is a symbol that represents a value. IT can be used to store and manipulate data within a program. Variables are essential in programming, as they allow us to work with data in a dynamic way. In PHP, variables are denoted by a dollar sign ($), followed by the name of the variable.
Declaring Variables
To declare a variable in PHP, you simply need to use the dollar sign followed by the variable name, like this:
$name;
$age;
$salary;
Once declared, you can then assign a value to the variable using the assignment operator (=). For example:
$name = "John";
$age = 25;
$salary = 2500.50;
Variable Names
Variable names in PHP must start with a letter or underscore, followed by any number of letters, numbers, or underscores. Variable names are case-sensitive, meaning that $name and $Name would be considered different variables.
It’s important to choose meaningful and descriptive variable names to make your code more readable and maintainable. For example, instead of using $n for a variable representing the number of items, you could use $numberOfItems.
Data Types in PHP
PHP supports various data types for variables, including:
- String: A sequence of characters, like “Hello, World!”
- Integer: A whole number, like 25
- Float: A number with a decimal point, like 2500.50
- Boolean: A value that can be either true or false
- Array: A collection of values
- Object: An instance of a class
- Null: A variable that has no value
Assigning Values to Variables
As mentioned earlier, you can assign a value to a variable using the assignment operator. Here are a few examples:
$name = "John";
$age = 25;
$isMale = true;
$prices = array(10, 20, 30);
$person = new Person();
$salary = null;
Using Variables in PHP
You can use variables in PHP to perform various operations, such as concatenating strings, performing mathematical calculations, and more. Here are some examples:
$firstName = "John";
$lastName = "Doe";
$fullName = $firstName . " " . $lastName;
echo $fullName; // Output: John Doe
$num1 = 10;
$num2 = 5;
$sum = $num1 + $num2;
echo $sum; // Output: 15
Conclusion
Variables are an essential concept in PHP programming. They allow us to store and manipulate data, making our code dynamic and flexible. By understanding how to declare, assign, and use variables effectively, you can enhance the functionality and efficiency of your PHP applications.
FAQs
1. Can variables in PHP change their data type?
Yes, in PHP, variables can change their data type as the program executes. This is known as dynamic typing.
2. Can I use spaces in variable names?
No, variable names in PHP cannot contain spaces. Use underscores or camel case for multi-word variable names.
3. Is it necessary to declare a variable before using it in PHP?
No, it’s not necessary to declare a variable before using it in PHP, but it’s considered a best practice to do so to avoid errors and improve code readability.
4. Are variables in PHP case-sensitive?
Yes, variable names in PHP are case-sensitive. $name and $Name would be considered different variables.
5. Can I use numbers at the beginning of a variable name in PHP?
No, variable names in PHP cannot start with a number. They must start with a letter or an underscore.
6. How do I delete a variable in PHP?
In PHP, you can unset a variable using the unset() function. For example: unset($variableName);
7. Can I use special characters in variable names?
Yes, you can use special characters like $, _, etc., in variable names in PHP.
It’s essential to have a good understanding of variables in PHP to write efficient and effective code. By following the basics of declaring, assigning, and using variables, you can take full advantage of this fundamental concept in PHP programming.