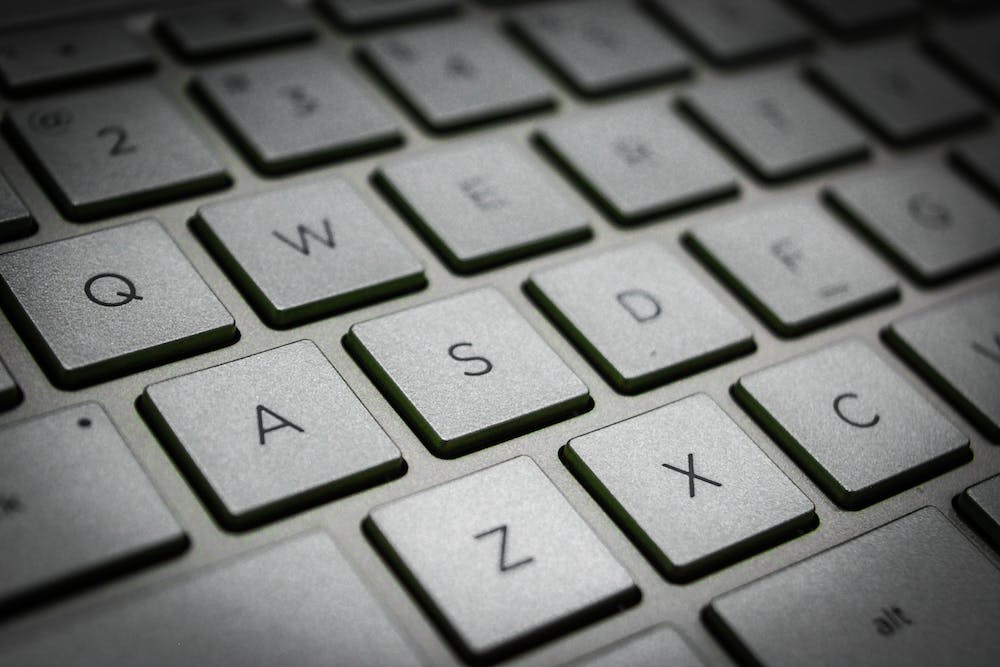
The Basics of PHP Programming for Beginners
Introduction
PHP is a widely used open-source scripting language that is specifically designed for web development. IT is a powerful tool that enables developers to create dynamic and interactive websites. If you are new to PHP programming, this article will provide you with an overview of the basics of PHP programming and get you started on your journey to becoming a proficient PHP developer.
Setting up a PHP Development Environment
Before you can start programming in PHP, you need to set up a PHP development environment on your computer. The first step is to install a web server such as Apache or Nginx, which will host your PHP files. You will also need to install PHP itself. There are several ways to install PHP, depending on your operating system. Once you have PHP installed, you can use a text editor or an integrated development environment (IDE) to write your PHP code. Some popular text editors for PHP programming include Sublime Text, Atom, and Notepad++. Alternatively, you can use an IDE like PhpStorm or Visual Studio Code, which provide additional features such as code completion and debugging.
Basic Syntax
PHP scripts are enclosed in special tags, , which allow you to switch between HTML and PHP code seamlessly. Let’s start with a simple example:
“`php
echo “Hello, world!”;
?>
“`
In this example, the PHP code inside the `` tags will be executed on the server and the output, “Hello, world!”, will be sent to the client’s browser. The `echo` statement is used to output text or variables. PHP statements must end with a semicolon (;).
Variables and Data Types
Like most programming languages, PHP allows you to store data in variables. A variable is created by using the dollar sign ($) followed by the variable name. PHP variables are dynamically typed, which means they do not have to be declared with a specific data type. Here’s an example:
“`php
$name = “John”;
$age = 25;
$isStudent = true;
?>
“`
In this example, we have created three variables: `$name` which stores a string, `$age` which stores an integer, and `$isStudent` which stores a boolean value.
Control Structures
Control structures allow you to control the flow of your PHP program. The most commonly used control structures are if-else statements and loops.
If-else Statements
An if-else statement is used to execute a block of code if a certain condition is true, and another block of code if the condition is false. Here’s an example:
“`php
$age = 20;
if ($age < 18) {
echo “You are not old enough to vote.”;
} else {
echo “You are eligible to vote.”;
}
?>
“`
In this example, if the variable `$age` is less than 18, the first block of code will be executed, otherwise, the second block of code will be executed.
Loops
Loops are used to repeat a block of code multiple times. The most commonly used loops in PHP are the `for` loop and the `while` loop.
For Loop
The `for` loop allows you to execute a block of code a specified number of times. Here’s an example:
“`php
for ($i = 1; $i <= 10; $i++) {
echo $i . “, “;
}
?>
“`
In this example, the loop will iterate 10 times, and each time IT will output the current value of the variable `$i` followed by a comma. The output will be “1, 2, 3, 4, 5, 6, 7, 8, 9, 10,”.
While Loop
The `while` loop allows you to execute a block of code as long as a certain condition is true. Here’s an example:
“`php
$i = 1;
while ($i <= 10) {
echo $i . “, “;
$i++;
}
?>
“`
In this example, the loop will iterate as long as the variable `$i` is less than or equal to 10. The output will be the same as the `for` loop example.
Conclusion
In this article, we have covered the basics of PHP programming for beginners. We have discussed how to set up a PHP development environment, the basic syntax of PHP, variables and data types, as well as control structures such as if-else statements and loops. By understanding these fundamentals, you are now equipped to start writing PHP code and exploring the endless possibilities of web development with PHP.
FAQs
Q: What is PHP?
PHP is a widely used open-source scripting language that is specifically designed for web development. IT is a powerful tool that enables developers to create dynamic and interactive websites.
Q: How do I set up a PHP development environment?
To set up a PHP development environment, you need to install a web server such as Apache or Nginx, and then install PHP itself. You can use a text editor or an integrated development environment (IDE) to write your PHP code.
Q: What are the basic syntax and data types in PHP?
PHP scripts are enclosed in `` tags. PHP variables are created using the dollar sign ($) followed by the variable name. PHP supports various data types such as strings, integers, floats, booleans, arrays, and objects.
Q: How do I control the flow of my PHP program?
PHP provides control structures such as if-else statements and loops to control the flow of your program. If-else statements are used to execute different blocks of code based on certain conditions, while loops allow you to repeat a block of code multiple times.