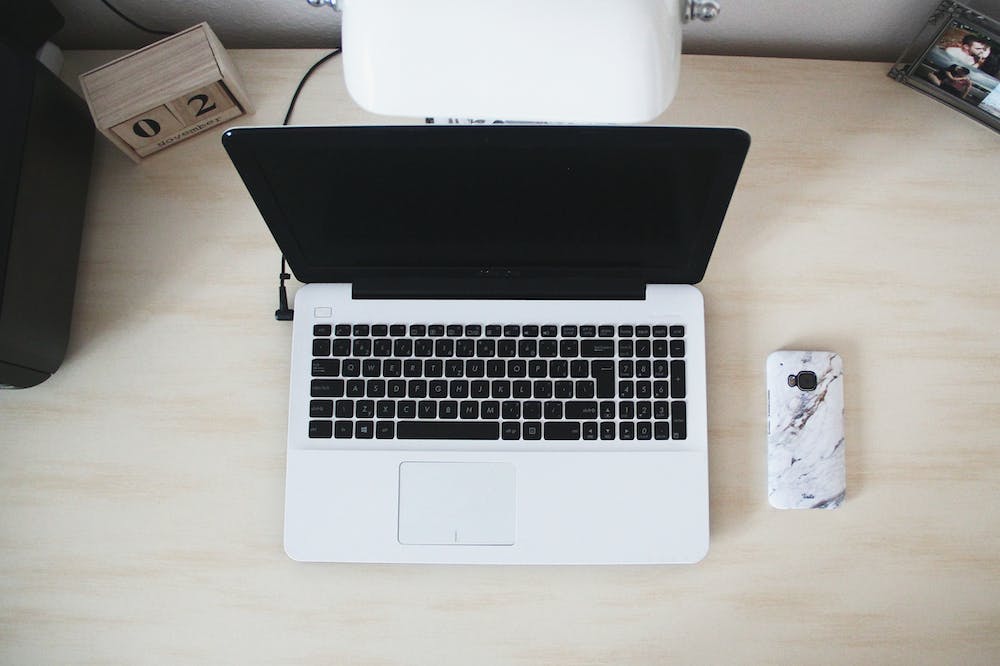
The Basics of Node.js: A Beginner’s Guide
Node.js is a powerful JavaScript runtime environment that allows developers to build scalable and high-performance web applications. IT is built on Chrome’s V8 JavaScript engine, which provides excellent speed and efficiency. Whether you are a beginner or an experienced developer, IT is essential to understand the basics of Node.js to unlock its full potential. In this beginner’s guide, we will explore the fundamental concepts and features of Node.js.
What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code on the server-side. Traditionally, JavaScript was executed on the client-side, running within a web browser. However, with Node.js, developers can now execute JavaScript code on the server-side, enabling them to build full-fledged web applications using only JavaScript.
Node.js provides an event-driven, non-blocking I/O model, which makes IT efficient and suitable for building real-time applications that require high concurrency. IT uses an event loop to handle concurrent requests, allowing multiple operations to be performed simultaneously without blocking or waiting for each other to complete.
Features of Node.js
Node.js comes with several features that make IT a popular choice among developers:
- Asynchronous and Non-Blocking: Node.js uses an event-driven architecture, allowing developers to write non-blocking code. This means that multiple operations can be executed concurrently, resulting in faster and more efficient web applications.
- Scalable: Node.js is highly scalable due to its non-blocking I/O model. IT can handle a large number of concurrent connections without consuming excessive system resources.
- Extensive Package Ecosystem: Node.js has a vast ecosystem of packages and libraries available through npm (Node Package Manager). This allows developers to easily integrate third-party modules and reuse code, reducing development time and effort.
- Real-time Applications: With its event-driven architecture and support for WebSockets, Node.js is an excellent choice for building real-time applications such as chat applications, collaborative tools, and gaming servers.
- Server-side Rendering: Node.js can be used for server-side rendering of web pages, enabling better SEO (Search Engine Optimization) and improved performance.
Setting up Node.js
Before you start building applications with Node.js, you need to set up your development environment. Follow these steps to get started:
- Download and install Node.js from the official Website (https://nodejs.org).
- Verify the installation by opening a command prompt or terminal and running the following command:
node -v
If the installation was successful, you will see the version of Node.js installed on your system.
Creating a Simple Hello World Application
Now that you have Node.js set up, let’s create a simple “Hello World” application. Open a text editor and create a new file named “app.js”.
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.listen(3000, 'localhost', () => {
console.log('Server running at http://localhost:3000/');
});
Save the file and open a command prompt or terminal in the same directory. Run the following command to start the server:
node app.js
You should see a message indicating that the server is running at http://localhost:3000/. Open your web browser and navigate to that URL. You should see the “Hello, World!” message displayed.
Conclusion
Node.js is a powerful runtime environment that allows developers to build high-performance web applications using JavaScript on the server-side. Its non-blocking and event-driven architecture makes IT suitable for building real-time applications and handling high concurrency. With its extensive package ecosystem and scalability, Node.js has become a popular choice for developers worldwide.
FAQs
1. What is the difference between Node.js and JavaScript?
JavaScript is a programming language that can be executed in various contexts, such as web browsers. Node.js, on the other hand, is a runtime environment that allows JavaScript to be executed on the server-side. Node.js provides additional features and APIs specifically designed for server-side development.
2. Is Node.js only used for web development?
No, Node.js can be used for various types of applications, including web development, command-line tools, desktop apps, and more. Its versatility and extensive package ecosystem make IT suitable for a wide range of use cases.
3. What is npm?
npm (Node Package Manager) is the default package manager for Node.js. IT allows developers to install, manage, and share reusable packages and libraries. With npm, you can easily integrate third-party modules into your Node.js projects.
4. Is Node.js suitable for beginners?
Yes, Node.js can be a great choice for beginners, especially if you are already familiar with JavaScript. Its simplicity and vast community support make IT easier to get started and find resources for learning.
5. Can Node.js be used with other programming languages?
Yes, Node.js can be used alongside other programming languages. IT can act as a backend server for applications written in languages such as Python, Ruby, or Java. This allows developers to leverage the benefits of Node.js while still using their preferred programming language for other parts of the application.