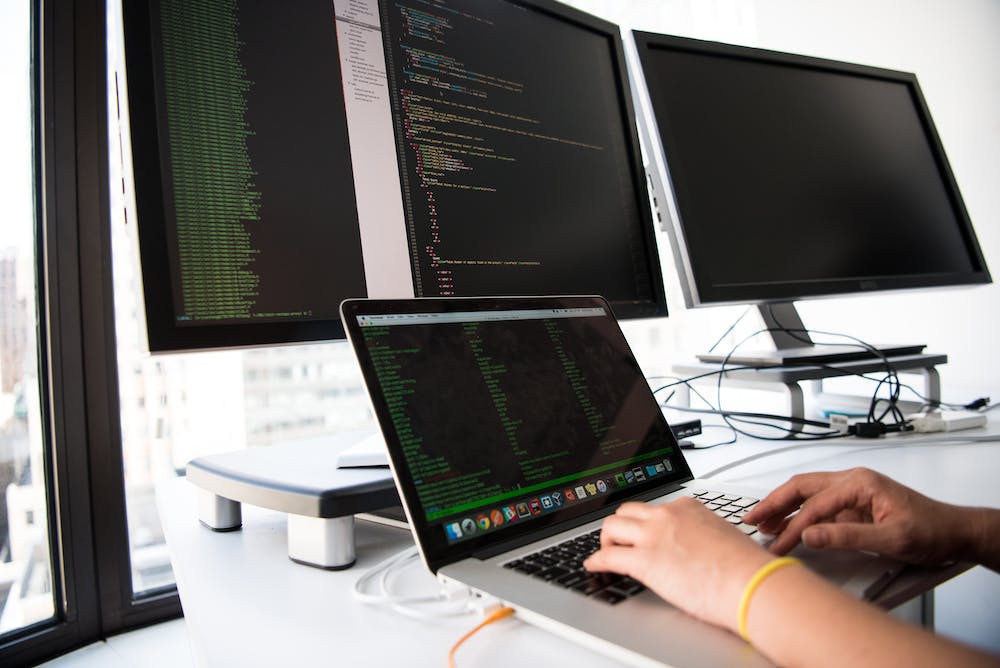
Python is a powerful and versatile programming language that is widely used for web development, data analysis, and automation. One of the key features of Python is its requests library, which allows developers to make HTTP requests to interact with web services and APIs. In this article, we will uncover some surprising secrets about Python requests response codes that you may not be aware of. By the end of this article, you’ll gain a deeper understanding of how response codes work and how to handle them effectively in your Python applications.
Understanding Response Codes
Before we dive into the surprising secrets of Python requests response codes, let’s first understand what response codes are and why they are important. When you make an HTTP request to a web server, the server responds with a status code that indicates the outcome of the request. These status codes are three-digit numbers that are grouped into different categories, such as 2xx for successful requests, 4xx for client errors, and 5xx for server errors.
For example, the most common response code is 200, which indicates that the request was successful. On the other hand, a response code of 404 means that the requested resource was not found on the server. Understanding these response codes is crucial for building robust and reliable web applications, as they allow you to handle different scenarios and provide appropriate feedback to users.
Surprising Secrets Revealed
Now, let’s uncover some surprising secrets about Python requests response codes that you may not be aware of. These insights will help you write more efficient and resilient code when working with HTTP requests in your Python applications.
1. Redirection Handling
When you make an HTTP request, the server may respond with a redirection status code, such as 301 or 302, which indicates that the requested resource has been moved to a different location. In Python requests, these redirections are automatically followed by default, meaning that the library will transparently handle the redirection and return the final response. However, you can customize this behavior by using the `allow_redirects` parameter when making a request.
import requests
response = requests.get('https://example.com', allow_redirects=False)
if response.status_code == 301:
print('The requested resource has been permanently moved')
elif response.status_code == 302:
print('The requested resource has been temporarily moved')
2. Retrying Failed Requests
In some cases, the initial HTTP request may fail due to network issues or server unavailability. Python requests provides a convenient way to automatically retry failed requests using the `Retry` class from the `urllib3` library. By configuring a retry policy, you can specify the maximum number of retry attempts, backoff intervals, and status codes to retry on.
import requests
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
session = requests.Session()
retry_strategy = Retry(
total=3,
status_forcelist=[500, 502, 503, 504],
method_whitelist=frozenset(['GET']),
backoff_factor=1
)
adapter = HTTPAdapter(max_retries=retry_strategy)
session.mount('http://', adapter)
session.mount('https://', adapter)
response = session.get('https://example.com')
3. Custom Error Handling
Python requests allows you to define custom error handling logic for specific response codes using the `Response.raise_for_status()` method. This method will raise an HTTPError exception if the response status code indicates a client or server error. By catching and handling these exceptions, you can implement custom error recovery or notification mechanisms in your application.
import requests
try:
response = requests.get('https://example.com')
response.raise_for_status()
except requests.exceptions.HTTPError as errh:
print('Http Error:', errh)
except requests.exceptions.ConnectionError as errc:
print('Error Connecting:', errc)
except requests.exceptions.Timeout as errt:
print('Timeout Error:', errt)
except requests.exceptions.RequestException as err:
print('OOps: Something Else', err)
Conclusion
In this article, we have uncovered some surprising secrets about Python requests response codes that can help you write more robust and reliable code. By understanding how redirection handling, retry policies, and custom error handling work in Python requests, you can improve the resilience and performance of your web applications. These insights will enable you to handle different HTTP response scenarios effectively and provide a better user experience.
FAQs
Q: Can I customize the default behavior for following redirects in Python requests?
A: Yes, you can use the `allow_redirects` parameter to control the default behavior for following redirects in Python requests. Setting this parameter to `False` will prevent automatic redirection, allowing you to handle redirections manually.
Q: How can I implement a retry policy for failed HTTP requests in Python requests?
A: You can use the `Retry` class from the `urllib3` library to configure a retry policy for failed HTTP requests in Python requests. By specifying the maximum number of retry attempts, status codes to retry on, and backoff intervals, you can define a customized retry strategy.
Q: Is IT possible to define custom error handling for specific response codes in Python requests?
A: Yes, you can use the `Response.raise_for_status()` method to define custom error handling logic for specific response codes in Python requests. This method will raise an HTTPError exception for client or server errors, allowing you to catch and handle these exceptions in your code.