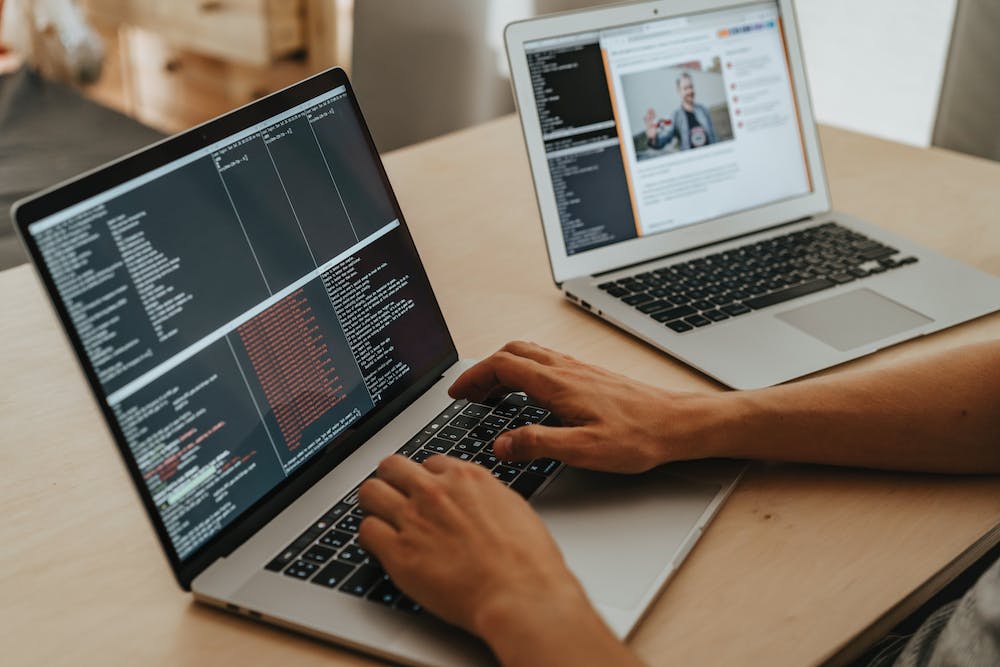
PHP is a powerful scripting language that is widely used for web development. One of its key features is arrays, which are used to store multiple values in a single variable. PHP arrays offer a wide range of functions and methods for searching and manipulating data. In this article, we will explore some surprising PHP array find tricks that will take your coding skills to the next level.
1. Using array_search()
The array_search()
function is a handy tool for finding the key of a specific value in an array. IT returns the key if the value is found, and false
if the value is not present in the array. Let’s take a look at an example:
“`php
$colors = array(“red”, “green”, “blue”, “yellow”);
$key = array_search(“blue”, $colors);
echo “The key for ‘blue’ is: ” . $key;
“`
In this example, the array_search()
function would return 2, as “blue” is the third element in the array and array keys start from 0.
2. Using array_column()
The array_column()
function is used to extract a single column of values from a multi-dimensional array. This can be incredibly useful when you have an array of data and want to retrieve a specific column of values. Here’s an example:
“`php
$users = array(
array(“id” => 1, “name” => “John”, “age” => 25),
array(“id” => 2, “name” => “Jane”, “age” => 30),
array(“id” => 3, “name” => “Mike”, “age” => 22)
);
$names = array_column($users, “name”);
print_r($names);
“`
After running this code, the output will be an array containing the names of the users: Array ( [0] => John [1] => Jane [2] => Mike )
3. Using array_filter()
The array_filter()
function is a powerful tool for filtering values in an array based on a specified condition. It takes a callback function as its second argument, which is applied to each value in the array. Here’s an example to demonstrate its usage:
“`php
$numbers = array(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
$even_numbers = array_filter($numbers, function($n) {
return $n % 2 == 0;
});
print_r($even_numbers);
“`
After running this code, the output will be an array containing the even numbers: Array ( [1] => 2 [3] => 4 [5] => 6 [7] => 8 [9] => 10 )
4. Using in_array()
The in_array()
function is a simple yet effective way to check if a value exists in an array. It returns true
if the value is found, and false
if the value is not present in the array. Here’s an example:
“`php
$fruits = array(“apple”, “banana”, “orange”, “grape”);
if (in_array(“banana”, $fruits)) {
echo “We found the banana!”;
} else {
echo “The banana is missing!”;
}
“`
In this case, the output would be: “We found the banana!”
5. Using array_key_exists()
The array_key_exists()
function is used to check if a specific key exists in an array. It returns true
if the key exists, and false
if the key is not present in the array. Here’s an example:
“`php
$data = array(
“name” => “John”,
“age” => 25,
“city” => “New York”
);
if (array_key_exists(“age”, $data)) {
echo “The ‘age’ key exists!”;
} else {
echo “The ‘age’ key is missing!”;
}
“`
In this example, the output would be: “The ‘age’ key exists!”
Conclusion
PHP arrays are incredibly versatile and offer a wide range of functions and methods for finding and manipulating data. By mastering the array find tricks outlined in this article, you can streamline your coding and take your PHP skills to the next level. Whether you’re working with simple arrays or complex multi-dimensional arrays, these tricks can help you improve the efficiency and readability of your code. Experiment with these functions and explore their capabilities to unlock the full potential of PHP arrays.
FAQs
1. Can I use these PHP array find tricks with multi-dimensional arrays?
Yes, all the array find tricks mentioned in this article can be used with multi-dimensional arrays. You can apply these functions and methods to extract, filter, and search for values within multi-dimensional arrays to streamline your data manipulation tasks.
2. Are there any performance considerations when using these array find tricks?
While PHP array functions are generally efficient, it’s important to consider performance implications when working with large arrays. Some functions, such as array_search()
and array_column()
, may have higher time complexity for large arrays, so it’s wise to test and benchmark your code to ensure optimal performance.
3. Where can I find more information about PHP array functions and methods?
For comprehensive documentation and examples of PHP array functions and methods, you can refer to the official PHP manual at php.net. Additionally, there are many online resources, tutorials, and forums where you can explore advanced array manipulation techniques and best practices.