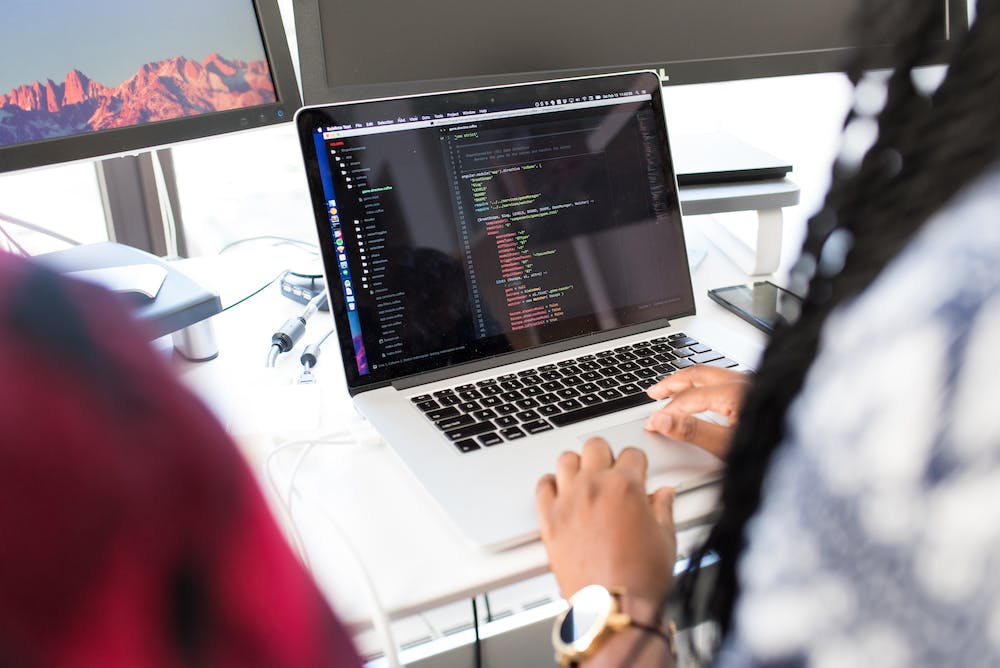
Sending emails through a Website is a common requirement for many web applications. PHP provides a built-in function to send emails, making IT a popular choice for developers. In this tutorial, we will cover the step-by-step process of sending emails with PHP, including setting up a mail server, creating a contact form, and handling the email sending process.
Prerequisites
Before we start, make sure you have a basic understanding of PHP and HTML. Additionally, you will need access to a web server with PHP support. If you don’t have a web server, you can use a local development environment like XAMPP or WAMP to follow along.
Step 1: Setting up the Mail Server
The first step in sending emails with PHP is to set up the mail server. Most web servers have a built-in mail server, so you don’t need to install anything. However, if you are using a local development environment, you may need to configure the SMTP settings.
To configure the SMTP settings, open the php.ini file and locate the [mail function] section. Update the SMTP settings according to your email provider’s instructions. For example, if you are using Gmail, you will need to set the SMTP server to smtp.gmail.com and provide your Gmail username and password.
Step 2: Creating a Contact Form
Once the mail server is set up, you need to create a contact form on your website. This form will collect the user’s input, such as their name, email address, and message, and send it to the recipient’s email address.
Here’s an example of a simple contact form in HTML:
“`html
“`
In this example, the form’s action attribute is set to “send-email.php”, which is the PHP script responsible for sending the email.
Step 3: Sending the Email with PHP
Now, let’s create the send-email.php script that will handle the email sending process. This script will use the PHP mail() function to send the email. Here’s an example of the send-email.php script:
“`php
if ($_SERVER[“REQUEST_METHOD”] == “POST”) {
$name = $_POST[“name”];
$email = $_POST[“email”];
$message = $_POST[“message”];
$to = “[email protected]”;
$subject = “New Email from $name”;
$body = “Name: $name\nEmail: $email\n\n$message”;
if (mail($to, $subject, $body)) {
echo “Email sent successfully”;
} else {
echo “Email failed to send”;
}
}
“`
In this script, we first check if the request method is POST, indicating that the form has been submitted. We then retrieve the user input from the $_POST superglobal array and use it to construct the email message. Finally, we call the mail() function with the recipient’s email address, subject, and body, and display a success or failure message based on the result.
Step 4: Testing the Contact Form
With the send-email.php script in place, you can now test the contact form by submitting a message. After submitting the form, you should see a success message if the email is sent successfully. If the email fails to send, double-check your SMTP settings and ensure that the recipient’s email address is correct.
Conclusion
Congratulations! You have successfully learned how to send emails with PHP. By following this step-by-step tutorial, you can now implement email functionality in your web applications. Sending emails with PHP is a powerful feature that enhances user interaction and communication on your website.
FAQs
Q: Can I send HTML-formatted emails with PHP?
A: Yes, PHP’s mail() function supports sending HTML-formatted emails. You can include HTML tags in the email body to format the content, add images, and create links.
Q: How can I send attachments with PHP emails?
A: You can send email attachments using the PHP mail() function by specifying the path to the file as an additional parameter. For more advanced email handling, you may consider using third-party libraries like PHPMailer.
Q: Is it possible to send multiple recipients in a single email using PHP?
A: Yes, you can send emails to multiple recipients by separating the email addresses with commas in the $to parameter of the mail() function.
Q: What are the common issues in sending emails with PHP?
A: Common issues with sending emails with PHP include incorrect SMTP settings, email being marked as spam, and limitations on shared hosting environments. To address these issues, consider using dedicated email services or SMTP relay services.